help - computer guessing game
Author |
Message |
whoareyou

|
Posted: Tue Mar 08, 2011 9:48 am Post subject: help - computer guessing game |
|
|
What is it you are trying to achieve?
The computer is supposed to guess a number you have thought of.
The computer should guess a number that you have thought of until it gets it right.
It should be smart, so if the computer guesses 2 and you say that the number is low, it would go
to one and then if you press low to that, if would say you changed your number.
What is the problem you are having?
I have no idea where to start.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
I will upload the sample program (exe) file later for you to actually see what its supposed to look like.
Turing: |
var hidden, guess : int
var reply : string (1)
put "See if you can guess the hidden number"
put "It is between 1 and 99 inclusive"
loop
var count : int := 0
put "Do you want to play? Answer y or n " ..
get reply
exit when reply = "n"
randint (hidden, 1, 99)
loop
put "Enter your guess ",
"(any number between 1 and 99) " ..
get guess
count := count + 1
if guess < hidden then
put "You are low"
elsif guess > hidden then
put "You are high"
else
put "You got it in ", count, " guesses"
exit
end if
end loop
end loop
|
Please specify what version of Turing you are using
4.1.1
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
mirhagk
|
Posted: Tue Mar 08, 2011 2:21 pm Post subject: RE:help - computer guessing game |
|
|
your code is doing the opposite, it's letting the user guess, the computer is not doing the guess, verify what it's actually supposed to do.
|
|
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 3:15 pm Post subject: Re: help - computer guessing game |
|
|
yes i know. this is the fundamental code; i have to rework it so that its the opposite.
but i dont know where to start.
i've also included the sample program so that you can see what i'm talking about.
Description: |
|
 Download |
Filename: |
GuessNumberComputer.rar |
Filesize: |
267.1 KB |
Downloaded: |
174 Time(s) |
|
|
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 4:37 pm Post subject: Re: help - computer guessing game |
|
|
here is my code so far.
its not working the way i want it too.
for example, when it produces a number (i.e. 60) and i say that the number is higher, then it will show numbers below 60 sometimes
Turing: |
var high: int:= 99
var low: int:= 1
var reply_yesno: string
var reply_highlow: string
var fguess: int
var count: int:= 0
randint (fguess, 1, 99)
put "I will try to find out a number you guess between 1 and 99 inclusive."
loop
put "Do you want to play? Y or N?"
get reply_yesno
if reply_yesno = "Y" or reply_yesno = "n" or reply_yesno = "y" or reply_yesno = "n" then
else
put "Invalid response!"
end if
exit when reply_yesno = "N" or reply_yesno = "n"
loop
put "Is your number ", fguess, "?"
get reply_yesno
if reply_yesno = "Y" or reply_yesno = "y" then
put "Great! I got in in ", count, " tries!"
else
put "Is your number 'high'er or 'low'-er?"
get reply_highlow
if reply_highlow = "high" then
fguess := low
randint (fguess, low, high )
elsif reply_highlow = "low" then
fguess: = high
randint (fguess, low, high )
else
put "Incorrect Response!"
end if
end if
end loop
end loop
|
|
|
|
|
|
 |
Tony
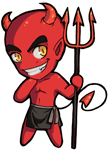
|
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 5:17 pm Post subject: Re: help - computer guessing game |
|
|
Tony @ Tue Mar 08, 2011 5:09 pm wrote: whoareyou @ Tue Mar 08, 2011 4:37 pm wrote: it will show numbers below 60 sometimes
Yes. Since you are telling the computer to guess by picking a random number.
If you rewrite you code to use Rand.Int instead of randint, you will probably see your problem.
i tried to re-write the code using the Rand.Int, and no difference. also, i think im changing the range of the random integer when i write
Turing: |
put "Is your number 'high'er or 'low'-er?"
get reply_highlow
if reply_highlow = "high" then
fguess := low
randint (fguess, low, high )
elsif reply_highlow = "low" then
fguess: = high
randint (fguess, low, high )
else
put "Incorrect Response!"
|
|
|
|
|
|
 |
Tony
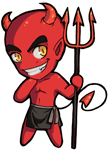
|
Posted: Tue Mar 08, 2011 5:22 pm Post subject: RE:help - computer guessing game |
|
|
The latest code still uses randint, not Rand.Int
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 6:12 pm Post subject: Re: help - computer guessing game |
|
|
is there a difference between randint and Rand.Int? they seem pretty similar except that Rand.Int is assigned to the var directly (i.e. var hello:int:=Rand.Int ...) where as randint changes the value later on ... (i.e. randint (hello, 1, 6)
the way i learned in school was randint ...
anyways, the i updated my code to use the Rand.Int, and it still does not make a difference.
Turing: |
var high : int := 99
var low : int := 1
var reply_yesno : string
var reply_highlow : string
var fguess : int:= Rand.Int (low, high)
var count : int := 0
put "I will try to find out a number you guess between 1 and 99 inclusive."
loop
put "Do you want to play? Y or N?"
get reply_yesno
if reply_yesno = "Y" or reply_yesno = "n" or reply_yesno = "y" or reply_yesno = "n" then
else
put "Invalid response!"
end if
exit when reply_yesno = "N" or reply_yesno = "n"
loop
put "Is your number ", fguess, "?"
get reply_yesno
if reply_yesno = "Y" or reply_yesno = "y" then
put "Great! I got in in ", count, " tries!"
else
put "Is your number 'high'er or 'low'-er?"
get reply_highlow
if reply_highlow = "high" then
fguess := low
fguess := Rand.Int (low, high)
elsif reply_highlow = "low" then
fguess := high
fguess := Rand.Int (low, high)
else
put "Incorrect Response!"
end if
end if
end loop
end loop
|
i dont understand why it keeps doing that when i put the if statement to change the range of the random integer being generated.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
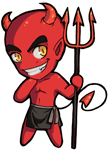
|
Posted: Tue Mar 08, 2011 6:16 pm Post subject: RE:help - computer guessing game |
|
|
Good. Now look at this part really hard. Read it out loud to yourself. Explain it to a rubber duck -- http://compsci.ca/blog/rubber-ducks-help-best-with-computer-science/
Try to answer the question: how is the "high" guess different from "low" guess?
code: |
if reply_highlow = "high" then
fguess := low
fguess := Rand.Int (low, high)
elsif reply_highlow = "low" then
fguess := high
fguess := Rand.Int (low, high)
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 6:32 pm Post subject: RE:help - computer guessing game |
|
|
For "HIGH"
if the number is higher than what the computer guessed, it would take that current number stored in fguess and make that the new "low" value, then the computer will generate a new fguess using the fguess as a starting point, to high, which is still 99.
For "LOW"
if the number is lower than what the computer guess, then that current number would be stored as the new high value, then a new fguess would be generated starting from low to the previous number the computer generated.
|
|
|
|
|
 |
Tony
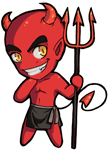
|
Posted: Tue Mar 08, 2011 6:44 pm Post subject: RE:help - computer guessing game |
|
|
That sounds like the right thing to do. Good.
But in your code, compare the lines where the number is actually picked:
code: |
fguess := Rand.Int (low, high)
...
fguess := Rand.Int (low, high)
|
low and high never change.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 7:08 pm Post subject: Re: help - computer guessing game |
|
|
so basically, i was just setting fguess back to one for "high" and back to 99 for "low"
so to fix that ...
Turing: |
if reply_highlow = "high" then
low := fguess + 1
fguess := Rand.Int (low, high)
elsif reply_highlow = "low" then
high := fguess - 1
fguess := Rand.Int (low, high)
|
?
and the +1 and -1 are so that the numbers don't repeat themselves?
|
|
|
|
|
 |
Tony
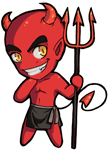
|
|
|
|
 |
whoareyou

|
Posted: Tue Mar 08, 2011 9:01 pm Post subject: RE:help - computer guessing game |
|
|
ok THANK YOU!
i think i'll be learning about algorithms laters on in the semester!
|
|
|
|
|
 |
|
|