Any base to another
Author |
Message |
TaKer
|
Posted: Mon May 31, 2010 5:30 pm Post subject: Any base to another |
|
|
I need help in creating a function that will convert one base to another.
Say if i was converting base 7 to base 12.
Is there a pattern to creating functions to any base?
i Understand the math involved to go from base to base, but the coding part i cant seem to get.
Here is a code that i created to convert base 7 to base 12.
Tell me wat i did wrong or i could do better.
Turing: |
function base7tobase12(iNum:int):string
var D1: int := iNum div12
var D2: int := iNum mod 12
var temp : string :""
if D1 = 10 then
temp := temp + "A"
elsif D1 = 11 then
temp := temp + "B"
end if
if D2 = 10 then
temp := temp + "A"
elsif D2 = 11 then
temp := temp + "B"
end if
result temp
end base7tobase12
|
Please specify what version of Turing you are using
<Answer Here> |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Cezna

|
Posted: Mon May 31, 2010 5:51 pm Post subject: RE:Any base to another |
|
|
I know that to make it a little simpler, you can change the
temp := temp + "A"
to
temp += "A"
For the actual base converter, use the values of the columns of the starting number
For example, for binary:
ones column: 1 (2 ^ 0)
twos column: 1 (2 ^ 1)
fours column: 0 (2 ^ 2)
eights column: 0 (2 ^ 3)
sixteens column: 0 (2 ^ 4)
So it is the base to the power of the column, time the value in the column
I know this probably isn't a lot of help, but it's all I can think of.
I'll edit if I think of anything more, but I doubt I will. |
|
|
|
|
 |
TaKer
|
Posted: Mon May 31, 2010 5:57 pm Post subject: Re: Any base to another |
|
|
Ok scratch my first idea into to going from base to base directly. Is going from base 7 to decimal, then decimal to base 12 easier?
If so i kno i would need 2 functions. Can anyone help me in converting the base 7 to decimal? |
|
|
|
|
 |
Cezna

|
Posted: Mon May 31, 2010 6:37 pm Post subject: Re: Any base to another |
|
|
let's say the value of each column is c
the value in column c is represented as x]
the base of the current system (in the case of the first calculation, 7), will be base
to get the new value, multiply x by base to the power of c
so the new value is x * base ** c
then just pull off any overflow (carry), and send it to the next column
if you want any easy way to check the column, I would just convert the number to a string using intstr, then have
x := strint ((intstr (the whole number to be converted would go here)) (c))
That's not very clean, but it should work. |
|
|
|
|
 |
Tony
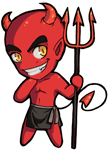
|
Posted: Mon May 31, 2010 6:48 pm Post subject: Re: Any base to another |
|
|
TaKer @ Mon May 31, 2010 5:30 pm wrote:
if D1 = 10 then
temp := temp + "A"
That's a lot of repetition if you want to go up to Z (and beyond? any base, right?)
look into ord and chr pair. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DtY

|
Posted: Mon May 31, 2010 6:49 pm Post subject: Re: Any base to another |
|
|
TaKer @ Mon May 31, 2010 5:57 pm wrote: Ok scratch my first idea into to going from base to base directly. Is going from base 7 to decimal, then decimal to base 12 easier?
If so i kno i would need 2 functions. Can anyone help me in converting the base 7 to decimal? Depends on what you mean by to decimal;
To convert between two bases, the easiest way is to first convert it to the native base, for a human, this would be base 10, for a computer it would be base 2. When you read the number in, and add it to the total sum, it is converting it to the native base, which on the computer is base 2. If you did it on paper, base 10 would be convenient, because that's what's easy to work with. Converting to decimal on a computer directly would be a challenge, but when you say convert to decimal, you are probably referring to the native base, the one that the computer can do arithmetic on.
The way you would convert one base to another is to add up the value of all the digits, for example 2#1001 would be (1*2^0) + (0*2^1) + (0*2^2) + (1*2^3) in whatever base you want to convert to. So, if you wanted to convert to base 5, you would need to be able to do arithmetic on base 5, which you would have to implement yourself.
Rather, as you just asked, it would be easier to convert it first to the native base, since you can do arithmetic on that. |
|
|
|
|
 |
Insectoid

|
Posted: Mon May 31, 2010 6:50 pm Post subject: RE:Any base to another |
|
|
There is no easy way to convert base X to base Y other than going to base 10 first. Converting anything to base 10 is easy, and 10 to any other base is easy as well. It's prolly your best bet. |
|
|
|
|
 |
DtY

|
Posted: Mon May 31, 2010 7:15 pm Post subject: Re: RE:Any base to another |
|
|
Insectoid @ Mon May 31, 2010 6:50 pm wrote: There is no easy way to convert base X to base Y other than going to base 10 first. Converting anything to base 10 is easy, and 10 to any other base is easy as well. It's prolly your best bet. As I explained in my post, doing that on a computer is just as hard, you want to go to the native base, which would be base 2. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Mon May 31, 2010 7:21 pm Post subject: RE:Any base to another |
|
|
Ah, I didn't see your post as you posted it while I was typing. Looking at the times, a mere two minutes passed between Tony's post and mine. |
|
|
|
|
 |
TaKer
|
Posted: Mon May 31, 2010 9:34 pm Post subject: RE:Any base to another |
|
|
i do not understand how to put this into code using 2 functions.
base7 to decimal- mathematically
you multiply each column of number, so
first column wud be (7**2) multiplied by the number in the column
second column (7**1) multiplied by the number in tht column
third column (7**0) multiplied by the number in tht column
Once u get the three products add them together and you get the decimal number.
decimal to base12- mathematically
get the decimal number obtained from the previous function.
for the first column it would be (12**2) divided my the number and then subtact from the original number
second column (12**1) divided by the new number then subtract the remainder
third column (12**0) divided with the remaining amount |
|
|
|
|
 |
|
|