How to make a sprite go 360 and move correspondingly
Author |
Message |
david92ng
|
Posted: Tue Jul 29, 2008 9:47 pm Post subject: How to make a sprite go 360 and move correspondingly |
|
|
I am making this space shooter game.
The sprite has to be able to turn 360 and move to a target angle. I also need help in movement of the sprite, moving 360 has different changes in x and y. and it must corospond to current angle. Also, i want a delayed turn, not like (current angle = target angle)The thing is... i have no idea how to do it...
how it will be able to do that is that the target angle will be determined by the poisition of the mouse, which i already figured out.
My old approach was to check if target angle was larger or smaller then current angle. But movement really defied physics because movement and angle of where its pointing is dependant upon themselves. So... if someone can tell me the code or at least tell me the theory please.
Also, how do i make bullets go 360? My bullets are basically a line, (x,y,x2,y2) and after a loop, x:=x2 y:=y2. Therefore, my bullets fly. but i cant get to control the speed of it. I tried to find the slope of the trejectory of the bullet and kinda try it that way but its all confusing... ugh,,
So please... can someone help me write this...? im sure your games have this simple code/concept that i havent seen yet...(i know forum rules but really need this done, working on this too long >.<)
Thank you all |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Zeroth
|
|
|
|
 |
Tony
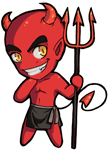
|
Posted: Wed Jul 30, 2008 1:21 am Post subject: RE:How to make a sprite go 360 and move correspondingly |
|
|
vector geometry makes movement really easy. polar vectors help with rotation. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Wed Jul 30, 2008 8:49 am Post subject: RE:How to make a sprite go 360 and move correspondingly |
|
|
Note: This requires at least grade 10 math (assuming an Ontario high school). You will need to know sine / cosine / tangent rules, but should be able to survive with just that.
Rotating images:
In Turing, there is a handy function called Pic.Rotate() which will create a copy of the image, rotate that copy, then return the reference to it (pic ID). What you should do is do a sufficient number of rotations (usually 72 should do - 360 degrees in increments of 5 degrees, it's difficult to tell with less than that) and store them all in an array, when your game starts:
Turing: |
var masterPic : int % Master copy of the picture, loaded from file
var numRotations : int := 72 % Using this to allow you to change the number of increments you use
var pics : array 1..numRotations of int % Contains all the images corresponding to various rotations
% Load the master copy of the picture
Pic.fromFile ( "myPic" ) % Look up this library function yourself, I don't have Turing here
% Make all the rotated pictures
for i : 1..numRotations
% The i * (360 / numRotations) part is the number of degrees to rotate by...work it out on paper yourself if you doubt it.
pics ( i ) := Pic.Rotate ( masterPic, (360/numRotations )*i )
end for
|
Rotating the image each time you want to draw also works, but only for about five seconds - after that, Turing has created too many pictures and dies. Using this method, you only create 73 pictures, instead of the thousands you would otherwise.
Moving Along an Arbitrary Path:
I'm going to assume you have some way of storing the degree of rotation of your objects, and some common baseline used as 0 degrees, and where 90 degrees is, etc.
Assuming that you choose like math does:
- 0 degrees is to the RIGHT
- 90 degrees is UP
- 180 degrees is LEFT
- 270 degrees is DOWN
Then all you need is this:
Turing: |
x + = speed * cos ( angle )
y + = speed * sin ( angle )
|
That's about all that's required. Yes, it looks too easy, but it's really not. It would get slightly trickier in a different coordinate system, but not too badly.
Seeking Bullets:
You mention some things that sound like you want to make bullets that seek their target. In this case, you have two options: implement something that's actually a seeking algorithm for such a device (difficult) or make a good-looking approximation (easy).
I couldn't tell you how to actually seek properly, but I can tell you that the easy way is to just have the missiles act like their target is really massive - calculate gravity between target and missile and apply that to the missile's current speed (later applying the missile's speed to it's position). This would require at least grade 11 Physics and some math skill. |
|
|
|
|
 |
david92ng
|
Posted: Wed Jul 30, 2008 7:04 pm Post subject: Re: How to make a sprite go 360 and move correspondingly |
|
|
I am JUST grade 10.... and thanks for your help guys. vectory geometry and polar grid, no idea how to use because i havent learnt it yet. will ask my tutor for math help.
Im pro with turning =D just suck at math T.T but i will eventually figure it out. |
|
|
|
|
 |
Tony
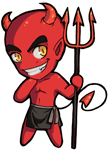
|
Posted: Wed Jul 30, 2008 8:05 pm Post subject: Re: How to make a sprite go 360 and move correspondingly |
|
|
david92ng @ Wed Jul 30, 2008 7:04 pm wrote: Im pro with turning =D just suck at math
That's kind of like like being pro at using a(n English) keyboard, and trying to write an essay in French.
That is to say that programming (especially in game dev) is largely an application of Math. So while you will get to all this Math eventually, you might want to look into things like geometry and physics on your own. It sounds like your programming level is well above the math, so the latter needs to catch up. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
david92ng
|
Posted: Wed Jul 30, 2008 8:10 pm Post subject: Re: How to make a sprite go 360 and move correspondingly |
|
|
lol, i havent started physics... you only start physics in grade 11 in canada. and that beginning stuff on vectors in grade 10 isnt helpful.
I hate math yet i love programming... why is that O.o mabie because i like createing games. lol
i already know some of that stuff at the top but i cant figure how to combine movement and rotation to be in unison. |
|
|
|
|
 |
Tony
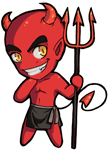
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|