Author |
Message |
Moofassa
|
Posted: Wed Apr 13, 2005 8:11 am Post subject: Turing Functions. |
|
|
mod div ceil floor. These are all predefined functions in turing. I know what they do, but im curious to know how they work. What is the actual code behind these functions?
ex. function mod
this does this then
this
bla end mod
yeah, anyone know? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
jamonathin

|
Posted: Wed Apr 13, 2005 8:31 am Post subject: (No subject) |
|
|
Think of how those commands work. I'll let you figure it out yourself.
Hint: Use if statements and round |
|
|
|
|
 |
Moofassa
|
Posted: Wed Apr 13, 2005 8:34 am Post subject: (No subject) |
|
|
Ok Thanks. I have another question, though. Would you know how to get the Greatest Common Divisor out of two numbers?
For example:
GCD of 25 and 15 = 5 |
|
|
|
|
 |
Tony
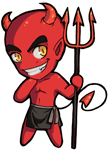
|
Posted: Wed Apr 13, 2005 8:48 am Post subject: (No subject) |
|
|
Turing: |
function GCD (num1, num2 : int) : int
var fac1, fac2 : flexible array 0 .. 0 of int
for i : 1 .. num1
if num1 mod i = 0 then
new fac1, upper (fac1 ) + 1
fac1 (upper (fac1 )) := i
put i
end if
end for
put "**"
for i : 1 .. num2
if num2 mod i = 0 then
new fac2, upper (fac2 ) + 1
fac2 (upper (fac2 )) := i
put i
end if
end for
result 0
end GCD
put GCD (25, 15)
|
The above function displays all the factors of two numbers. What you then do is filter out all the factors that are unique to just one number or another (compare element in one array against all in another).
This should leave you with two identical arrays of common factors between the two numbers. Highest element is your answer |
|
|
|
|
 |
Duncan
|
Posted: Wed Apr 13, 2005 9:13 am Post subject: (No subject) |
|
|
Hey, I was just looking at your reply and was wondering what a "flexible array" and a "new" is in that program you wrote.
Thanks |
|
|
|
|
 |
Martin

|
Posted: Wed Apr 13, 2005 9:17 am Post subject: (No subject) |
|
|
Tony being a bad programmer.
Type 'flexible' in turing and press F10. |
|
|
|
|
 |
Duncan
|
Posted: Wed Apr 13, 2005 9:21 am Post subject: (No subject) |
|
|
I have done that but I still don't see what it does. Do you know the definition for a flexible array? |
|
|
|
|
 |
Martin

|
Posted: Wed Apr 13, 2005 9:28 am Post subject: (No subject) |
|
|
It's an array with a variable upper bound. It allows you to change the length of the array whenever you want. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Moofassa
|
Posted: Wed Apr 13, 2005 9:34 am Post subject: (No subject) |
|
|
These are awesome thanks. Back to my original question. What is the actual code behind mod div ceil and floor. I've figured most of them out. but in my own crappy code. So just curious if any of you guys would help me out thanks! |
|
|
|
|
 |
Martin

|
Posted: Wed Apr 13, 2005 10:23 am Post subject: (No subject) |
|
|
mod - gives the remainder when dividing two integers.
5 mod 3 is the remainder when 5 is divided by 3, or 2.
div - gives the largest integer less than the quotient of the two numbers.
5 div 2 is the largest integer less than (5/2) 2.5, or 2.
ceil - rounds a real number up to the least integer greater than the number.
ceil (2.3) = 3.
floor - rounds a real number down to the greatest integer less than the number.
floor (5.9) = 5 |
|
|
|
|
 |
|