Hashes
Author |
Message |
wtd
|
Posted: Thu Mar 31, 2005 1:25 pm Post subject: Hashes |
|
|
A Hash, or "associative array" can be thought of as very similar to a regular array, except that instead of indexing by numbers we can (in terms of Ruby at least) index by anything. Most commonly we use strings.
So, if I want to have information about an individual, I could write:
code: | info = ["Bob Smith", 42, "129 55th Ave"] |
Now, how to I retrieve the name? Oh, right. I need to remember that it's the first element in the array.
Now I could change the age:
But, in these cases I have to go back and look at the original creation of the array to see where each item is. There has to be a better way.
There is. Instead of using an array, use a hash.
code: | info = { "name" => "Bob Smith", "age" => 42, "address" => "129 55th Ave" } |
Now, let's get the name again.
code: | name = info["name"] |
And let's change the age.
Any other questions?  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
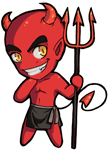
|
Posted: Thu Mar 31, 2005 3:06 pm Post subject: (No subject) |
|
|
I was actually hoping for a bit more advanced hash handling.
Such as looping through the elements and adding new hashes to the list. |
|
|
|
|
 |
wtd
|
Posted: Thu Mar 31, 2005 3:57 pm Post subject: (No subject) |
|
|
Getting all keys:
code: | my_hash = {"foo" => "bar"}
my_hash.keys |
Getting all values:
code: | my_hash = {"foo" => "bar"}
my_hash.values |
Looping:
code: | my_hash = {"foo" => "bar"}
my_hash.each { |key, value| puts "#{key}: #{value}" }
my_hash.each { |pair| puts "#{pair.first}: #{pair.last}" }
for key, value in my_hash
puts "#{key}: #{value}"
end
for pair in my_hash
puts "#{pair.first}: #{pair.last}"
end |
Determining if a hash contains a particular key or value:
code: | my_hash = {"foo" => "bar"}
my_hash.has_key? "foo"
my_hash.has_value? "bar" |
Adding to a hash:
code: | my_hash = {"foo" => "bar"}
my_hash["hello"] = "world" |
|
|
|
|
|
 |
wtd
|
Posted: Thu Mar 31, 2005 11:25 pm Post subject: (No subject) |
|
|
More.
Let's start with removing entries from a hash if they meet certain conditions. Ket's say we want to remove any entry where the value is exactly two times the key.
code: | my_hash = {"a" => "aa", "b" => "bbb", 9 => 19, 4 => 8}
new_hash = my_hash.reject { |key, value| value == key * 2 } |
new_hash is now:
code: | {"b" => "bbb", 9 => 19} |
Next... ever want the keys and values switched?
code: | my_hash = {"a" => "aa", "b" => "bbb", 9 => 19, 4 => 8}
new_hash = my_hash.invert |
And one last trick:
Ever want to retrieve multiple entries into an array?
code: | my_hash = {"a" => "aa", "b" => "bbb", 9 => 19, 4 => 8}
my_array = my_hash.indexes("a", 9) |
|
|
|
|
|
 |
wtd
|
Posted: Fri Apr 01, 2005 11:59 pm Post subject: (No subject) |
|
|
Anything else anyone would like to know about Ruby hashes? |
|
|
|
|
 |
Tony
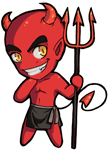
|
Posted: Sat Apr 02, 2005 1:06 pm Post subject: (No subject) |
|
|
thx wtd, awesome examples as always  |
|
|
|
|
 |
|
|