Author |
Message |
josh
|
Posted: Sun Oct 03, 2004 12:24 pm Post subject: Factorial Calculator |
|
|
I have to make a program that accepts a number between 1 and 30 from the user and then calcualte the factorial of the number. The code I have so far when I input the number 4 it says the factorial is 0 when it should be 24.
here is my code:
code: |
%----------------------------------------------------------------------------
%Factorial Calculator
%By: Josh Rosen
%Date: October 3, 2004.
%Version: 1.0.0
%
%Input: A number between 1 and 30
%Output: The factorial of the inputted number
%Process: Use a loop to continue. Use a for loop within the continuous loop to calculate.
%----------------------------------------------------------------------------
%Declare Variables
var fact : int %The factorial of the inputed number
var num : int %The number to calculate the factorial for
var cont : string %Holds the users answer to continue or not
%Continuous loop
loop
cls
put "What number would youy like to calculate the factorial of? (number must be between 1 and 30)"
get num
fact := num
for decreasing i : num .. 1
fact:= i*(i-1)
end for
put "The factorial of ", num, " is ", fact
put ""
put "Do you wish to continue? Y/N"
get cont
exit when cont = "N" or cont = "n"
end loop
|
Can someone tell me what I am doing wrong. I know it has something to do with my equation inside of the for loop.
thanx |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
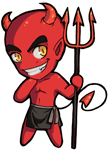
|
Posted: Sun Oct 03, 2004 1:24 pm Post subject: (No subject) |
|
|
code: |
for decreasing i : num .. 1
fact:= i*(i-1)
|
i decreases down to 1, at which point it is
code: |
fact := 1 * (1 - 1)
|
1 * 0 is clearly 0
what you want is
code: |
fact := 1
for i : 1 .. num
fact:= fact * i
end for
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
josh
|
Posted: Sun Oct 03, 2004 2:03 pm Post subject: (No subject) |
|
|
Thanx for the help.
I should have realized that.  |
|
|
|
|
 |
wtd
|
Posted: Sun Oct 03, 2004 2:11 pm Post subject: (No subject) |
|
|
The recursive solution is simpler by far.
code: | function factorial(n : int) : int
if n = 1 then
result 1
else
result n * factorial(n - 1)
end if
end factorial |
|
|
|
|
|
 |
josh
|
Posted: Sun Oct 03, 2004 2:28 pm Post subject: (No subject) |
|
|
I am in grade 10 compsci adn we havent learnt functions yet. I know about them from Java but my teach wanted us to use loops within loops. |
|
|
|
|
 |
apomb

|
Posted: Mon Oct 04, 2004 9:16 am Post subject: (No subject) |
|
|
just a question rysticlight, you learned java before Turing!? why??
i started turing in gr 10 programming then java in gr 11 ... i found that the ppl that didnt have the background in turing didint do as well, is/was this the case in your class? |
|
|
|
|
 |
josh
|
Posted: Mon Oct 04, 2004 1:08 pm Post subject: (No subject) |
|
|
I took a two week course over the summer where you learn game programming in Java, made my own asteroids game which I am tryign to figure out how to compile so I can post here. I use eclipse.
there are difingntly alot of ppl in my class who should not be here but since we are learning turing I thik they will be O.k. My school does turing in grade 10, VB in grade 11, and Java in grade 12 |
|
|
|
|
 |
apomb

|
Posted: Mon Oct 04, 2004 1:26 pm Post subject: (No subject) |
|
|
WOW ... thats a kinda back asswards approach, although VB is Very object oriented and has some similarities to java (note some meaning none) however, have they ever considered C++ in 12 and JAVA in 11 and turing in 10 ... (and Flash, javascript and html in Gr 12-web developing) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
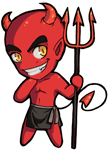
|
Posted: Mon Oct 04, 2004 1:43 pm Post subject: (No subject) |
|
|
vb is not object oriented... atleast not in the sence its taught
and the bottom line is that schools teach whatever the teacher wants.. or rather whatever your teacher might have a remote knowledge of  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
josh
|
Posted: Mon Oct 04, 2004 1:57 pm Post subject: (No subject) |
|
|
no I don't think my school would ever consider C++ right now (even though they have Visual C++ ) but I think this is the first year they did Java in grade 12.
and tony my skool hgas no web developing course that I know of (they do a bit of flash in comtech) |
|
|
|
|
 |
apomb

|
|
|
|
 |
wtd
|
Posted: Mon Oct 04, 2004 5:58 pm Post subject: (No subject) |
|
|
For what it's worth, VB.NET is very object-oriented. That doesn't make it good, but it's more important to know why a language sucks than just that it does. |
|
|
|
|
 |
|