Why can't I delete my bullets?
Author |
Message |
GlobeTrotter
|
Posted: Sun Jun 20, 2004 4:26 pm Post subject: Why can't I delete my bullets? |
|
|
code: |
setscreen ("graphics;offscreenonly")
var x, y, dir, speed : real
var chars : array char of boolean
var bullets : int := 0
var bulletx, bullety, bulletdir : flexible array 1 .. bullets of real
const bulletdelay := 20
var temp := bulletdelay
x := maxx div 2
y := maxy div 2
dir := 0
speed := 1
loop
cls
delay (10)
Input.KeyDown (chars)
if chars (KEY_LEFT_ARROW) then
dir += 1
elsif chars (KEY_RIGHT_ARROW) then
dir -= 1
end if
if chars (KEY_UP_ARROW) then
x += cosd (dir) * speed
y += sind (dir) * speed
elsif chars (KEY_DOWN_ARROW) then
x -= cosd (dir) * (speed / 2)
y -= sind (dir) * (speed / 2)
end if
temp += 1
if chars (KEY_ENTER) and temp >= bulletdelay then
temp := 0
bullets += 1
new bulletx, bullets
new bullety, bullets
new bulletdir, bullets
bulletx (bullets) := x + cosd (dir) * 10
bullety (bullets) := y + sind (dir) * 10
bulletdir (bullets) := dir
end if
drawfilloval (x div 1, y div 1, 10, 10, 12)
drawfilloval (round (x + cosd (dir) * 10), round (y + sind (dir) * 10), 2, 2, 7)
for i : 1 .. bullets
bulletx (i) += cosd (bulletdir (i)) * speed * 2
bullety (i) += sind (bulletdir (i)) * speed * 2
drawfilloval (bulletx (i) div 1, bullety (i) div 1, 1, 1, 4)
if bulletx (i) > 0 and bulletx (i) < maxx and bullety (i) > 0 and bullety (i) < maxy then
else
for k : i .. bullets
bulletx (k) := bulletx (k - 1)
bullety (k) := bullety (k - 1)
bulletdir (k) := bulletdir (k - 1)
end for
bullets -= 1
end if
end for
View.Update
end loop
|
The section
code: |
for k : i .. bullets
bulletx (k) := bulletx (k - 1)
bullety (k) := bullety (k - 1)
bulletdir (k) := bulletdir (k - 1)
end for
bullets -= 1
|
Should delete the bullet that hits the wall. I get an array out of range error. How should I properly do this? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
naoki

|
Posted: Sun Jun 20, 2004 6:12 pm Post subject: (No subject) |
|
|
i haven't run your program, but it looks like your array is out of range because ... your array is out of range.
see your flexible array? it starts from 1 to a variable.
see your "for i" ? it also goes from 1 to something. good start.
but notice your "k" statements? you change values at "k" to values at "k - 1". if the first bullet happens to go off the screen and doesn't hit an enemy, then you're attempting to set bullet (1)'s values to bullet (0). since your flexible array doesn't have any values at (0), it messes up.
hope it helped |
|
|
|
|
 |
GlobeTrotter
|
Posted: Sun Jun 20, 2004 10:06 pm Post subject: (No subject) |
|
|
code: |
setscreen ("graphics;offscreenonly")
var x, y, dir, speed : real
var chars : array char of boolean
var bullets : int := 0
var size := 30
var bulletx, bullety, bulletdir : flexible array 1 .. bullets of real
const bulletdelay := 20
var temp := bulletdelay
var hit : boolean := false
var checkx, checky : real
x := maxx div 2
y := maxy div 2
dir := 0
speed := 1
loop
cls
delay (10)
Input.KeyDown (chars)
if chars (KEY_LEFT_ARROW) then
dir += 1
elsif chars (KEY_RIGHT_ARROW) then
dir -= 1
end if
if chars (KEY_UP_ARROW) then
checky := y + sind (dir) * speed
checkx := x + cosd (dir) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars (KEY_DOWN_ARROW) then
checky := y - sind (dir) * speed
checkx := x - cosd (dir) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars ('0') then
checky := y + sind (dir - 90) * speed
checkx := x + cosd (dir - 90) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars (KEY_CTRL) then
checky := y + sind (dir + 90) * speed
checkx := x + cosd (dir + 90) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
end if
if chars ('a') then
size -= 1
elsif chars ('d') then
size += 1
end if
temp += 1
if chars (KEY_ENTER) and temp >= bulletdelay then
temp := 0
bullets += 1
new bulletx, bullets
new bullety, bullets
new bulletdir, bullets
bulletx (bullets) := x + cosd (dir) * size
bullety (bullets) := y + sind (dir) * size
bulletdir (bullets) := dir
end if
%drawoval (x div 1, y div 1, size, size, 7)
drawline (round (x + cosd (dir) * size), round (y + sind (dir) * size), round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), 7)
drawfill (x div 1, y div 1, 2, 7)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 5, size div 5, 7)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 10, size div 10, 0)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 40, size div 40, 7)
drawfilloval (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), 2, 2, 7)
drawfilloval (round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 2, 2, 7)
drawfilloval (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), 2, 2, 7)
drawfilloval (round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 2, 2, 7)
drawline (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 7)
drawline (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 7)
drawline (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 7)
drawline (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 7)
drawbox (10, 10, maxx - 10, maxy - 10, 7)
for i : 1 .. bullets
bulletx (i) += cosd (bulletdir (i)) * speed * 2
bullety (i) += sind (bulletdir (i)) * speed * 2
drawfilloval (bulletx (i) div 1, bullety (i) div 1, 1, 1, 4)
if bulletx (i) > 20 and bulletx (i) < maxx - 20 and bullety (i) > 20 and bullety (i) < maxy - 20 then
else
for k : i .. bullets - 1
bulletx (k) := bulletx (k + 1)
bullety (k) := bullety (k + 1)
bulletdir (k) := bulletdir (k + 1)
end for
bullets -= 1
end if
end for
View.Update
end loop
|
Okay I managed to fix that, but I've got another problem. Why doesn't my drawfill command work properly? Why doesn't it fill in the tank? For some reason, it fills in the whole area. |
|
|
|
|
 |
Tony
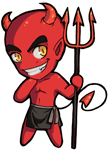
|
Posted: Mon Jun 21, 2004 12:10 am Post subject: (No subject) |
|
|
could it by any chance have something to do with you commenting out the circle to be filled  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
this_guy

|
Posted: Mon Jun 21, 2004 9:27 am Post subject: (No subject) |
|
|
wow....great code....
couple things...
sometimes the bullets travel slower than the tank, and also the game's really slow on my comp. |
|
|
|
|
 |
GlobeTrotter
|
Posted: Mon Jun 21, 2004 2:43 pm Post subject: (No subject) |
|
|
tony wrote: could it by any chance have something to do with you commenting out the circle to be filled 
No it doesn't. That circle is for testing purposes only. I want a filled in rectangle. Turing's drawfillbox does not allow you to rotate it. If I uncomment that circle, I get a filled in circle. I'm not sure why turing does not fill in the rectange only.
code: |
drawline (50, 0, 100, 50, 7)
drawline (100, 50, 50, 100, 7)
drawline (50, 100, 0, 50, 7)
drawline (0, 50, 50, 0, 7)
drawfill (50, 50, 2, 7)
|
This is basically what I want to happen. I want a filled in rectangle, and I know it should work since it works in this example. I don't know why it doesnt work in my other example. |
|
|
|
|
 |
Cervantes

|
|
|
|
 |
GlobeTrotter
|
Posted: Mon Jun 21, 2004 3:27 pm Post subject: (No subject) |
|
|
oops, that was stupid. Thanks.
I've got another question now. How can I make it so that when I hold down enter my game doesn't freeze. I understand why it freezes in my next example, I simply can't think of another way to accomplish the same task.
code: | setscreen ("graphics;offscreenonly")
var x, y, dir, speed : real
var chars : array char of boolean
var bullets : int := 0
var size := 30
var bulletx, bullety, bulletdir, bulletvz, bulletz : flexible array 1 .. bullets of real
var explosions : int := 0
var explodex, explodey, explodetime : flexible array 1 .. explosions of real
const bulletdelay := 20
var temp := bulletdelay
var temp1 := 0
var hit : boolean := false
var checkx, checky : real
x := maxx div 2
y := maxy div 2
var grav : real := 0.1
dir := 0
speed := 1
loop
cls
delay (1)
Input.KeyDown (chars)
if chars (KEY_LEFT_ARROW) then
dir += 1
elsif chars (KEY_RIGHT_ARROW) then
dir -= 1
end if
if chars (KEY_UP_ARROW) then
checky := y + sind (dir) * speed
checkx := x + cosd (dir) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars (KEY_DOWN_ARROW) then
checky := y - sind (dir) * speed
checkx := x - cosd (dir) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars ('0') then
checky := y + sind (dir - 90) * speed
checkx := x + cosd (dir - 90) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
elsif chars (KEY_CTRL) then
checky := y + sind (dir + 90) * speed
checkx := x + cosd (dir + 90) * speed
if checky >= 10 + size and checky <= maxy - 10 - size then
y := checky
end if
if checkx >= 10 + size and checkx <= maxx - 10 - size then
x := checkx
end if
end if
if chars ('a') then
size -= 1
elsif chars ('d') then
size += 1
end if
temp += 1
if chars (KEY_ENTER) and temp >= bulletdelay then
loop
Input.KeyDown (chars)
if temp1 <= 300 then
delay (2)
temp1 += 1
end if
exit when ~chars (KEY_ENTER)
end loop
temp := 0
bullets += 1
new bulletx, bullets
new bullety, bullets
new bulletdir, bullets
new bulletvz, bullets
new bulletz, bullets
bulletx (bullets) := x + cosd (dir) * size
bullety (bullets) := y + sind (dir) * size
bulletdir (bullets) := dir
bulletz (bullets) := 50
bulletvz (bullets) := temp1 / 50
temp1 := 0
end if
%drawoval (x div 1, y div 1, size, size, 7)
drawline (round (x + cosd (dir) * size), round (y + sind (dir) * size), round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), 7)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 5, size div 5, 7)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 10, size div 10, 0)
drawfilloval (round (x + cosd (dir) * (size / 2)), round (y + sind (dir) * (size / 2)), size div 40, size div 40, 7)
drawfilloval (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), 2, 2, 7)
drawfilloval (round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 2, 2, 7)
drawfilloval (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), 2, 2, 7)
drawfilloval (round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 2, 2, 7)
drawline (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 7)
drawline (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 7)
drawline (round (x - cosd (dir - size) * size), round (y - sind (dir - size) * size), round (x + cosd (dir + size) * size), round (y + sind (dir + size) * size), 7)
drawline (round (x + cosd (dir - size) * size), round (y + sind (dir - size) * size), round (x - cosd (dir + size) * size), round (y - sind (dir + size) * size), 7)
drawfill (x div 1, y div 1, 2, 7)
drawbox (10, 10, maxx - 10, maxy - 10, 7)
for i : 1 .. bullets
bulletx (i) += cosd (bulletdir (i)) * speed * 2
bullety (i) += sind (bulletdir (i)) * speed * 2
bulletz (i) += bulletvz (i)
bulletvz (i) -= grav
drawfilloval (bulletx (i) div 1, bullety (i) div 1, bulletz (i) div 50, bulletz (i) div 50, RGB.AddColor (1 - bulletz (i) / 300, 1 - bulletz (i) / 300, 1 -
bulletz (i) / 300))
if bulletx (i) > 20 and bulletx (i) < maxx - 20 and bullety (i) > 20 and bullety (i) < maxy - 20 and bulletz (i) >= 49 then
else
explosions += 1
new explodex, explosions
new explodey, explosions
new explodetime, explosions
explodex (explosions) := bulletx (i)
explodey (explosions) := bullety (i)
explodetime (explosions) := 30
for k : i .. bullets - 1
bulletx (k) := bulletx (k + 1)
bullety (k) := bullety (k + 1)
bulletdir (k) := bulletdir (k + 1)
bulletz (k) := bulletz (k + 1)
bulletvz (k) := bulletvz (k + 1)
end for
bullets -= 1
end if
end for
for i : 1 .. explosions
explodetime (i) -= 1
drawfilloval (explodex (i) div 1, explodey (i) div 1, explodetime (i) div 4 + 7, explodetime (i) div 4 + 7, RGB.AddColor (explodetime (i) / 15, explodetime (i) / 15, 0))
if explodetime (i) <= 0 then
for k : i .. explosions - 1
explodex (k) := explodex (k + 1)
explodey (k) := explodey (k + 1)
explodetime (k) := explodetime (k + 1)
end for
explosions -= 1
end if
end for
View.Update
end loop
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|