Moving Levels.
Author |
Message |
magicman

|
Posted: Thu May 20, 2004 9:06 am Post subject: Moving Levels. |
|
|
I'm making a level for my game. It's a long level. When the player reaches the end of the screen, i want it to move with the player. What is the code to do that???¿¿¿
thanks for the help.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
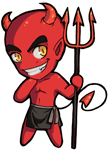
|
Posted: Thu May 20, 2004 12:39 pm Post subject: (No subject) |
|
|
you keep the player in the center of the screen and draw the level relative to the player.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
magicman

|
Posted: Thu May 20, 2004 2:53 pm Post subject: (No subject) |
|
|
How would you make the player jump???¿¿¿
|
|
|
|
|
 |
SuperGenius
|
Posted: Thu May 20, 2004 2:58 pm Post subject: (No subject) |
|
|
have it check for the up button(or whichever button) being pressed, and then have the y coord of the sprite/picture increase and then decrease
|
|
|
|
|
 |
magicman

|
Posted: Mon May 31, 2004 6:59 am Post subject: (No subject) |
|
|
What kind of coding would i have to do for the y coord to increase and decrease??
|
|
|
|
|
 |
SuperGenius
|
Posted: Mon May 31, 2004 2:52 pm Post subject: (No subject) |
|
|
Here's a diagram. Dont let it confuse you because it has nothing to do with the x coord. It is a rough parabola. It would be a little tough to do because for every unit of time the change in position is a different amount. I'll give you an example. (keyword example)
code: |
yheight=timeelapsed**
|
time elapsed is the time that has passed since the jump started (when the key was pressed. You will also need to add a constant to the y value (unless your man normally walks at coord (x,0). The constant you need to add is the height of the ground that you used in your game (ex if he is running on a flat surface, that is the number that I am talking about)
Description: |
|
Filesize: |
6.9 KB |
Viewed: |
4172 Time(s) |

|
|
|
|
|
|
 |
Cervantes

|
Posted: Mon May 31, 2004 3:07 pm Post subject: (No subject) |
|
|
erg, personally I wouldn't use Time.Elapsed.
IMO, here's a simpler way of doing it.
code: |
setscreen ("offscreenonly")
var keys : array char of boolean
var jump := false %boolean. since it's declared as false turing knows its boolean
var x, y, vy : real %vy = velocity in the y plane. aka, the upwards velocity of the player.
x := 100
y := 100
const gravity := 0.1
loop
Input.KeyDown (keys)
if keys (KEY_UP_ARROW) and not jump then %"and not jump" is the same as "and jump = false"
jump := true
vy := 5
end if
if jump then %"if jump then" is the same as "if jump = true then"
y += vy % same as y := y + vy
vy -= gravity % same as vy := vy - gravity
if y <= 100 then
jump := false
end if
end if
drawfilloval (round (x), round (y), 15, 15, red)
delay (10)
View.Update
drawfilloval (round (x), round (y), 15, 15, white)
end loop
|
hope this helps
|
|
|
|
|
 |
SuperGenius
|
Posted: Mon May 31, 2004 4:06 pm Post subject: (No subject) |
|
|
i just meant that as an example, but i dunno the time module and stuff, so i just wrote that.
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rhomer
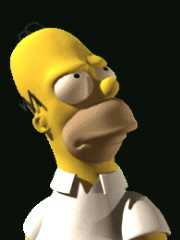
|
Posted: Mon May 31, 2004 6:05 pm Post subject: (No subject) |
|
|
My idea for you is to base all object as a distance away from the start of the ball...
I just started working off Cervantes code...
Its crappy cuz i did it very fast but it illustrates my point i think..
code: | setscreen ("offscreenonly")
var chars : array char of boolean
var jump : boolean := false
var ballx, bally, vy : real := 0
var xdir : int := 1
var boxx1, boxx2 : int
var xchange : int := 0
const gravity := 0.1
ballx := maxx div 2
bally := 100
boxx1 := maxx + 1
boxx2 := maxx + 11
loop
Input.KeyDown (chars)
if chars (KEY_UP_ARROW) and jump = false then
jump := true
vy := 5
elsif chars (KEY_RIGHT_ARROW) then
xchange += 1
if xchange > 100 then
boxx1 -= 1
boxx2 -= 1
end if
elsif chars (KEY_LEFT_ARROW) then
xchange += -1
boxx1 += 1
boxx2 += 1
end if
if jump = true then
bally += vy
vy -= gravity
if bally <= 100 then
jump := false
end if
end if
if ballx > boxx1 - 15 and ballx < boxx2 + 15 and bally < 200 then
ballx := boxx1 - 15
end if
drawfillbox (boxx1, 85, boxx2, 185, blue)
drawfilloval (round (ballx), round (bally), 15, 15, red)
delay (10)
View.Update
drawfillbox (boxx1, 85, boxx2, 185, white)
drawfilloval (round (ballx), round (bally), 15, 15, white)
end loop
|
|
|
|
|
|
 |
guruguru

|
Posted: Mon May 31, 2004 6:19 pm Post subject: (No subject) |
|
|
This topic had the similary problem of moving the background relative to the player. Click!
As for jumping, a little procedure like this can be called whenever up is pressed. Just a slight modification of rhomers code.
code: |
procedure ballJump
vy := 5
loop
bally += vy
vy -= gravity
cls
Draw.FillOval (round(ballx), round(bally), 15, 15, red)
View.Update
delay(10)
exit when bally <= 100
end loop
end ballJump
|
|
|
|
|
|
 |
s_climax
|
Posted: Wed Jun 02, 2004 9:04 pm Post subject: (No subject) |
|
|
code: |
function CircumColor (centrex : real, centrey : real, xradius : int, yradius : int) : boolean
var tempx, tempy : int
var collide : boolean
collide := false
for i : 1 .. 31
tempx := (round ((xradius + 1) * (sin (i)))) + round (centrex)
tempy := (round ((yradius + 1) * (cos (i)))) + round (centrey)
if whatdotcolor (tempx, tempy) ~= 0 then
collide := true
exit
end if
end for
result collide
end CircumColor
|
This is a function I made for this program. Draw some boxes and jump around. It should tell you whether you're character has hit anything not white
|
|
|
|
|
 |
magicman

|
Posted: Fri Jun 04, 2004 12:58 pm Post subject: (No subject) |
|
|
hey. I also need an easy way to make the screen move when my guy goes to the end of the run window.
|
|
|
|
|
 |
|
|