Help making Spray Can effect for paint program
Author |
Message |
Neja
|
Posted: Sun Jan 18, 2004 7:01 pm Post subject: Help making Spray Can effect for paint program |
|
|
I need to make a spray can effect ( random dots) around where th user clicks. I need an idea on how to do it or at least how to have a set perimeter. For example, if a user clicks on the coordianates (10,151) how would I make it so that in a small area around said coordnates is filled with dots?
I've fooled around for a bit with it, and I haven't gotten very far. I've been using Rand.Int. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Andy
|
Posted: Sun Jan 18, 2004 7:24 pm Post subject: (No subject) |
|
|
here, nxt time post ur attempts
code: |
proc spray (x, y, radius, clr : int)
var tempx, tempy : int
randint (tempx, -radius, radius)
randint (tempy, -radius, radius)
if (tempx ** 2 + tempy ** 2) < radius ** 2 then
drawdot (tempx + x, tempy + y, clr)
end if
end spray
var mx, my, mb : int
var count := 0
loop
mousewhere (mx, my, mb)
if mb = 1 then
spray (mx, my, 20, blue)
count += 1
if count = 10 then
count := 0
delay (1)
end if
else
count := 0
end if
end loop
|
|
|
|
|
|
 |
Neja
|
Posted: Sun Jan 18, 2004 7:39 pm Post subject: (No subject) |
|
|
Thank you.
Um, but could you explain proc spray? I'm not really able to follow it. |
|
|
|
|
 |
Andy
|
Posted: Sun Jan 18, 2004 7:42 pm Post subject: (No subject) |
|
|
well basicly i randomly generate dots and plug them in the circle formula to make sure they form a circle |
|
|
|
|
 |
McKenzie

|
Posted: Sun Jan 18, 2004 9:43 pm Post subject: (No subject) |
|
|
What Dodge is saying is:
If we did a randint from -20 to 20 in both x and y from some point we would get a square. eg. code: |
loop
randint (dx, -20, 20)
randint (dy, -20, 20)
drawdot(10+dx, 151 + dy,1) %per your example
end loop
|
If you want to ensure that they fall in a circle you use the equation of a circle (you can even understand the math with just pythagorean theorem.)
From there Dodge makes a very generic procedure to use with any location and any radius. |
|
|
|
|
 |
Neja
|
Posted: Sun Jan 18, 2004 10:57 pm Post subject: (No subject) |
|
|
Thank you 'Dodge' & Mr.Mckenzie. |
|
|
|
|
 |
Pickles

|
Posted: Sat Apr 17, 2004 1:49 pm Post subject: also need help |
|
|
code: | elsif kind = spray then
loop
Mouse.Where (x, y, button)
if button = 1 then
GUI.DrawDot (canvas, Rand.Int (x - 10, x + 10),Rand.Int(y - 10,y + 10), clr1)
x := mx
y := my
end if
end loop
end if |
Im making the spray paint (Right now I just have it as a square, but i can make it into a circle later.) The problem I run into is the GUI.DrawDot part on the canvas. To start off the spray can works but is not set at the mouse position, instead it is set up and to the right, and i cant figure out how to get it so its always with the mouse. Another problem is that the dots draw really slow. And finally, I cant click any other buttons after I have used the spray can, its still using it while im trying to click buttons to change.
If I use Draw.Dot it starts where the mouse is and everything, and draws fast enough.. Why is it different with GUI? |
|
|
|
|
 |
Pickles

|
Posted: Sat Apr 17, 2004 4:13 pm Post subject: .. |
|
|
Ive come to realize that the amount is off by is where the canvas starts.. By that i mean to say, that if i click at the bottom left corner of the run window it will be at the bottom left corner of the canvas, and will always be that distance away from my mouse |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
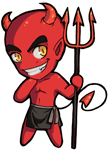
|
Posted: Sat Apr 17, 2004 5:19 pm Post subject: (No subject) |
|
|
Here's how GUI's DrawDot works
code: |
procedure DrawDot (x, y, clr : int)
BeforeDraw
Draw.Dot (x + originalX, y + originalY, clr)
AfterDraw
end DrawDot
|
So yes, it does draw relative to the bottom left corner of the canvas.
If you want to draw using global values you should use
code: |
GUI.DrawDot(x - canvasX, y - canvasY, color)
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Pickles

|
Posted: Sat Apr 17, 2004 9:16 pm Post subject: Thank you |
|
|
Thank you ever so much, the only other Question i would have about it would be is there a way to increase the speed in which they draw the dots ? I dont have a delay in there or anything now. and it seems slugish.. |
|
|
|
|
 |
Tony
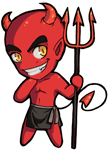
|
|
|
|
 |
the_short1
|
Posted: Sun Apr 18, 2004 4:30 pm Post subject: (No subject) |
|
|
cool..... i just had my paintcan effect from my paint program to be
code: |
for a : 1.. 25
drawdot (mx+Rand.Int(mx-5,mx+5),my+Rand.Int(my-5,my+5),colr)
end for
|
that drew 25 dots around the mouse position.... i didn;t realy venture into the circle deal...
Thanks DODGE!!!... thats a nice way to do that... i like it a lot...
NOTE: im really good in math... i just not good at getting it into turing  |
|
|
|
|
 |
TheZsterBunny

|
Posted: Sun Apr 25, 2004 3:01 pm Post subject: (No subject) |
|
|
heh. this takes me back to my spray can days:
code: |
setscreen ("offscreenonly,nobuttonbar,graphics:640;480")
Mouse.ButtonChoose ("multibutton")
var mousex, mousey, button, dotx, doty, spraypat : int
var density, size : int % for the size of the spray
var c1 : int % color
c1 := brightred
% Defaults
density := 50
size := 20
proc spray
mousex := 0
mousey := 0
drawfillbox (mousex, mousey, mousex + size, mousey + size, black)
drawfilloval (mousex + (size div 2), mousey + (size div 2), size div 2, size div 2, 0)
for specks : 1 .. density
randint (dotx, mousex, mousex + size)
randint (doty, mousey, mousey + size)
drawdot (dotx, doty, c1)
end for
drawbox (mousex - 1, mousey - 1, mousex + size + 1, mousey + size + 1, 214)
drawoval (mousex + (size div 2), mousey + (size div 2), size div 2, size div 2, 214)
drawfill (mousex, mousey, 0, 214)
drawfill (mousex + size, mousey, 0, 214)
drawfill (mousex, mousey + size, 0, 214)
drawfill (mousex + size, mousey + size, 0, 214)
drawoval (mousex + (size div 2), mousey + (size div 2), size div 2, size div 2, 0)
spraypat := Pic.New (mousex, mousey, mousex + size, mousey + size)
drawfillbox (mousex, mousey, mousex + size + 1, mousey + size + 1, 0)
end spray
loop
spray
mousewhere (mousex, mousey, button)
if button = 1 then
Pic.Draw (spraypat, mousex - (size div 2), mousey - (size div 2), picMerge)
end if
Pic.Free (spraypat)
View.Update
end loop
|
i wish i'd known the circle formula then. |
|
|
|
|
 |
Paul
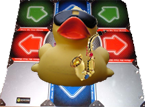
|
Posted: Sun Apr 25, 2004 3:18 pm Post subject: (No subject) |
|
|
whats at the bottom left corner? u can't draw there. |
|
|
|
|
 |
the_short1
|
Posted: Sun Apr 25, 2004 5:34 pm Post subject: (No subject) |
|
|
OMG!!!!! i did NOT know of drawfill.... that is AWSOME!!! that will make my paint prog bettter...
also.... that is EXACTLY what i needed for my Jezzball program!!!! **i know colors of wall so thats good...
[color=blue]+BITS[/c] ZesterBunny
.... ummm.... though of a hitch,.,,,
getting it to fill an area until it hits anycolor other then the one that was clicked on... (non-specific) any ideas??... |
|
|
|
|
 |
|
|