Survey/Multiple choice
Author |
Message |
AiR
|
Posted: Sat Mar 27, 2004 7:20 pm Post subject: Survey/Multiple choice |
|
|
For school I have to make a Multiple choice program which asks 5 questions 3 possible answers for each. It is suppose to be a simple program, and it's my first time writing programs with turing.
Would you guys have any tips on that?
I have tried using for count: 1..5. The only real part I am stuck on is that the program has to tell the user that if he doesn't enter a valid answer the program would tell him to enter A, B or C.
For that I tried:
if answer not= "a" and answer not= "b" and answer not= "c" then
put "This is an invalid answer please enter either A, B or C."
If I use the "If" statement I have to copy and paste it after every question and it ain't as effecient as it can be. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Paul
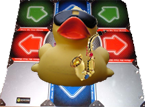
|
Posted: Sat Mar 27, 2004 7:32 pm Post subject: (No subject) |
|
|
you COULD do one procedure that checks if this a or b or c, then call it everytime you ask for input. |
|
|
|
|
 |
AsianSensation
|
Posted: Sat Mar 27, 2004 7:41 pm Post subject: (No subject) |
|
|
you can use a string variable to keep track of all your answers.
code: | var choices := "abc"
if index (choices, answer) = 0 then
put "you have not made a valid selection, please try again"
end if
|
index function seems to me to be the easiest. But since this is your first time programming with turing, I would suggest learning about index first. There is a tutorial on this site, in the tutorial section. |
|
|
|
|
 |
jonos

|
Posted: Sat Mar 27, 2004 8:30 pm Post subject: (No subject) |
|
|
you could create a procedure that acts like a function or something.
so:
code: |
proc checkAnswer (var userAnswer : int, var rightAnswer : int)
if userAnswer = rightAnswer then
put "you are right"
end if
end checkAnswer
var answer : int
put "what is the answer"
get answer
checkAnswer(answer, b)
|
i haven't checked that or anything or tested it, but it should be a good blueprint |
|
|
|
|
 |
AiR
|
Posted: Sun Mar 28, 2004 12:20 am Post subject: (No subject) |
|
|
Well I have tried both of your examples one said you haven't declared B and the other is that variable has no value. |
|
|
|
|
 |
AiR
|
Posted: Sun Mar 28, 2004 1:11 am Post subject: (No subject) |
|
|
Well I've got it:
var answer: string
loop
put " "
put "question1"
get answer
if answer = "a" then
put "You are correct"
elsif answer = "b" or answer = "c"
then put "You are incorrect, the answer is a."
elsif answer > "a" or answer > "b" or answer > "c" then
put "That is an invalid answer please enter either a, b, or c."
end if
put " "
put "question2"
get answer
if answer = "b" then
put "You are correct"
elsif answer = "a" or answer = "c"
then put "You are incorrect, the answer is b."
elsif answer > "a" or answer > "b" or answer > "c" then
put "That is an invalid answer please enter either a, b, or c."
end if
end loop
It works fine, but there is only one problem when the answer is invalid it does not ask the same question again instead it continues the cycle and it just moves on. Also I'll need to keep track of the score. If he only gets one right I would tell him in the end he had gotten 1 out of 2. |
|
|
|
|
 |
jonos

|
Posted: Sun Mar 28, 2004 1:38 am Post subject: (No subject) |
|
|
no you still had to declare variables and stuff, and i think i made a mistake though, i didn't try it out:
code: |
proc checkAnswer (var userAnswer : string, rightAnswer : string)
if userAnswer = rightAnswer then
put "you are right"
end if
end checkAnswer
var answer : string
put "what is the answer"
get answer
checkAnswer(answer, "b")
|
if you are just beginning then it may be a little tough to understand. what it does is itmakes a function/procedure that allows you to check if the answer is right, so if the answer is "c" then you would put:
checkAnswer(answer, c)
if the answer was d then:
checkAnswer(answer,b)
there was some problems before because i hadn't checked it, and i haven't really used those before ive only really seen other people do them. if you need help understanding it just say so in a post and i will try to help you or someone else will.
as to your problem:
for the score: just make a variable that is initialized as 2 and then if they get the question wrong, just take one from that variable:
var score: int := 2
if "question is wrong" then
score := score -1
end if
as to your other problem concerning it not repeating, you need to make 2 separate loops that your questions are inside, and if they get the question right, then exit the loop, but if it is wrong, the ndon't exist the loop. |
|
|
|
|
 |
Delos

|
Posted: Sun Mar 28, 2004 11:16 am Post subject: (No subject) |
|
|
Umm...guys!
Quote:
and it's my first time writing programs with turing
Ease off the procs and fcns and stuff! Mr/Ms AiR will get to that in due time. For now, concentrate on the basics...that's what intro CompSci classes are (supposed to be) about.
Ok, here's a bit of modified code to solve your problem:
code: |
var answer : string
loop
% Outside loop. Not really necassary unless you want to
% repeat the questioning.
loop
% 1st nested loop.
put " "
put "question1"
get answer
if answer = "a" then
put "You are correct"
exit
% Exit this loop, i.e. go on to the next question
% if the answer entered is correct...
elsif answer = "b" or answer = "c"
then
put "You are incorrect, the answer is a."
exit
% ...or the character entered is valid but
% incorrect.
elsif answer > "a" or answer > "b" or answer > "c" then
put "That is an invalid answer please enter either a, b, or c."
% Don't exit if an invalid answer is entered.
end if
end loop
loop
put " "
put "question2"
get answer
if answer = "b" then
put "You are correct"
exit
elsif answer = "a" or answer = "c"
then
put "You are incorrect, the answer is b."
exit
elsif answer > "a" or answer > "b" or answer > "c" then
put "That is an invalid answer please enter either a, b, or c."
end if
end loop
% Over here, you could put some sort of exit condition...
% ...if a certain score is reached/the User decides not to
% 'Try Again'...your call.
end loop
|
I'd like you to take note of how I used the [code] tags to make it look all nice and pretty upon this here Forum. Also, note how within Turing, I pressed F2 so as to indent my work.
Also take special note of the commenting...for not only does it explain things, but it is a habit teachers want you to get into. On your own, it's your decision whether to use it or not, but for teachers (and thus that much covetted mark), commenting is a must.
If you still are mildly interested in procedures etc, there're some good Tutorials available. But since this is your first bash w/ Turing (possibly programming too), you can leave that for a later date. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
AiR
|
Posted: Sun Mar 28, 2004 11:18 pm Post subject: (No subject) |
|
|
I appreciate all the help you guys have provided me with. The program has no problem now..
The only thing that's bugging me is that my coding may be ineffecient and lose marks for it. haha thanks for noticing that I'm new to the turing language and gave it to me lightly Delos.
Also for the score I guess I can use this right?
var score: int := 2
if "question is wrong" then
score := score -1 |
|
|
|
|
 |
|
|