My Newbish Game
Author |
Message |
PHP God
|
Posted: Mon Apr 21, 2003 6:07 pm Post subject: My Newbish Game |
|
|
I'm making a game where the user is prompted to select a character then select some starting coordinates and use the WSAD keys to move it around. Here is my code thus far (note it is not complete)
code: |
% The Moving Program
%%%%%%%%%%%%%%%%%%%%%%%
% Variables %
%%%%%%%%%%%%%%%%%%%%%%%
var moveChar:string (1)
var dirSel:string (1)
var winStatus:int:=Window.Open ("fullscreen")
var yesOrNo:string (1)
var xStart:int
var yStart:int
var anyKey:string (1)
%%%%%%%%%%%%%%%%%%%%%%%
% Procedures %
%%%%%%%%%%%%%%%%%%%%%%%
procedure moveUp
if xStart=1
then sound (1000,100)
elsif xStart=maxrow
then sound (1000,100)
end if
xStart:= xStart+1
end moveUp
%procedure moveDown
%procedure moveLeft
%procedure moveRight
%%%%%%%%%%%%%%%%%%%%%%%
% Program %
%%%%%%%%%%%%%%%%%%%%%%%
loop
put "There are ",maxrow," rows on this screen."
put "There are ",maxcol," columns on this screen."
put ""
put "You can chose a character and move it anywhere in these boundaries with this program."
put ""
put "Select You Character :: "..
getch (moveChar)
cls
loop
put "You have chosen to use ",moveChar,"."
put ""
put "Is this correct? (Y/N) :: "..
getch (yesOrNo)
if yesOrNo = "y" then
exit
elsif yesOrNo = "n" then
exit
else put ""
put "Invalid Input"
end if
cls
end loop
if yesOrNo = "y"
then exit
end if
cls
end loop
loop
cls
put "Choose the starting coordinates for your character"
put ""
loop
put "Choose an x value between 1 and ",maxrow,"."
get xStart
if xStart > maxrow then
put "Value is too high"
put ""
elsif xStart < 1 then
put "Value is too low"
put ""
else exit
end if
end loop
loop
put ""
put "Choose a y value between 1 and ",maxcol,"."
get yStart
if yStart > maxcol then
put "Value is too high"
put ""
elsif yStart < 1 then
put "Value is too low"
put ""
else exit
end if
end loop
exit
end loop
setscreen ("nocursor")
cls
put ""
put " CONTROLS"
put " ==============="
put " W - Move Up"
put " S - Move Down"
put " A - Move Left"
put " D - Move Right"
put ""
put " X - Quit"
put ""
put " Press Any Key To Start The Game..."
getch (anyKey)
cls
loop
cls
locate (xStart,yStart)
put moveChar
getch (dirSel)
if dirSel = "w"
then moveUp
elsif dirSel = "s"
then moveDown
elsif dirSel = "a"
then moveLeft
elsif dirSel = "d"
then moveRight
elsif dirSel = "x"
then exit
end loop
|
If you will examine the code at the very bottom
code: |
if dirSel = "w"
then moveUp
elsif dirSel = "s"
then moveDown
elsif dirSel = "a"
then moveLeft
elsif dirSel = "d"
then moveRight
|
You will see I have used if statements and depending on input, I am trying to call a procedure. when I try to run my program, it says that moveUp and all the others are not declared, well. At the top I declared moveUp and I'm stuck.
How do you call a procedure after a THEN statement? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
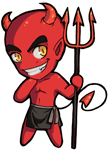
|
Posted: Mon Apr 21, 2003 6:13 pm Post subject: (No subject) |
|
|
you'd have to read a tutorial on procedures and fuctions, it might help out with examples.
a quick guess is that procedures need to have parameters, even if you dont pass any values to it. Such as
code: |
procedure something()
put "blah"
end something
|
notice that () in the procedure name. You might need to have it. Check tutorial for examples, its the best way to find errors - compare to working code |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Catalyst

|
Posted: Mon Apr 21, 2003 6:47 pm Post subject: (No subject) |
|
|
no, you dont need to do that in turing (ur prob thinking of c++)
you can have something like
code: |
procedure move
put "Hello"
end move
|
ne way the the only errors i get r concerning the other move procs not moveUp
also turing may have caught this but
code: | procedure moveUp
if xStart=1
then sound (1000,100)
elsif xStart=maxrow
then sound (1000,100)
end if |
is never ended
it shoudl be more like
code: |
procedure moveUp
if xStart=1
then sound (1000,100)
elsif xStart=maxrow
then sound (1000,100)
end if
end moveUp
|
[/code] |
|
|
|
|
 |
Tony
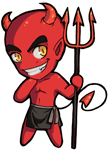
|
Posted: Mon Apr 21, 2003 7:01 pm Post subject: (No subject) |
|
|
ah yes... since you commented other procedures out, turing doesnt know what to call.
also it seems you dont have "end if" in there |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Prince
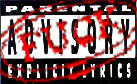
|
Posted: Mon Apr 21, 2003 7:07 pm Post subject: (No subject) |
|
|
its cus u hav the procedures moveDown, moveLeft and moveRight as comments (wit the % in front)... plus ders no code for it to b run in the first place  |
|
|
|
|
 |
Prince
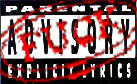
|
Posted: Mon Apr 21, 2003 7:08 pm Post subject: (No subject) |
|
|
nuts, u wer too quick for me tony ... lol |
|
|
|
|
 |
PHP God
|
Posted: Mon Apr 21, 2003 8:23 pm Post subject: (No subject) |
|
|
I know some are comments but moveUp isn't so it should work. my question was just in case you are illiterate, how do i call a procedure after a then statement? |
|
|
|
|
 |
Tony
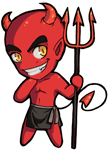
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Prince
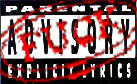
|
Posted: Mon Apr 21, 2003 9:29 pm Post subject: (No subject) |
|
|
think of it this way... if it worked wit one procedure (moveUp) wouldnt it click that doin the same thing would work wit all other procedures
*note* : its times like this i agree wit tony's sig |
|
|
|
|
 |
PHP God
|
Posted: Thu Apr 24, 2003 10:39 am Post subject: DUH!!! |
|
|
look
heres an example.
I have a bunch of ifs/elsifs
if this = that
then run
if black = white
then jump[/b
end if
notice the big red bolded ones? yeah, they are procedure name's we'll say, the procedures are all written and it doesn't matter what they say cuz thats not the point.
notice how i put the procedure name after [b]then?
well it won't run the procedur evn if the if's imply it should. |
|
|
|
|
 |
|
|