help with Net module
Author |
Message |
loonyli
|
Posted: Tue Mar 09, 2004 10:24 pm Post subject: help with Net module |
|
|
i'm trying to write a program that communicates with 3 other computers via TCP/IP using the Net module. this is fine with 1 connection between 2 computers. however, the trouble arises when i try to open more than 1 connection (1 each to the rest of the computers). it appears that the client program (the one that uses the Net.OpenConnection) does not want to connect with the host computer. the port numbers and the stream numbers should be set in a way that there should not be any conflicts between connections. is it due to errors in my code, or is it due to the limitations of the Turing language that causes this incapability in establishing multiple connections in the same program? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
recneps

|
Posted: Wed Mar 10, 2004 1:31 pm Post subject: (No subject) |
|
|
Limits of turing language, it can only connect 2, so what you gotta do is have the sever take a connection, then the port number goes up by one, then when the server sends/gets data, it checks all the streams (one stream per port) Understand? i believe there is a chat prog in source or submissioin that uses this method. |
|
|
|
|
 |
Tony
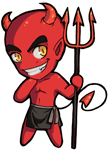
|
Posted: Wed Mar 10, 2004 7:23 pm Post subject: (No subject) |
|
|
that's not the limit of turing... port can host only 1 connection at a time
the idea here is managing multiple streams on different ports and organizing server/client setup/ info relay |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
loonyli
|
Posted: Wed Mar 10, 2004 9:26 pm Post subject: (No subject) |
|
|
i've tried using different ports and different streams for the 3 separate connections. maybe there's something wrong in my coding. is it possible to have the server "listen" on 3 different ports simultaneously (w/ difference processes)? and is there a way for the client program to test each connection to see if it is used or not? (i.e. client tests ports 10000, 10001, 10002 in order until it finds an unused port that the server is listening to)
and the example chat program sets up only 1 connection between 2 computers. |
|
|
|
|
 |
jonos

|
Posted: Wed Mar 10, 2004 9:29 pm Post subject: (No subject) |
|
|
if you post your code, then we may be able to know what is the problem. and id suggest not using processes, well firstly because tony will say something against them and thats not good cause hes boss, and secondly i think it will scramble up things if you are sending them across the net (im pretty sure i saw that in a program once.) |
|
|
|
|
 |
Tony
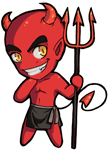
|
Posted: Wed Mar 10, 2004 9:44 pm Post subject: (No subject) |
|
|
haha don't use processes
main reason being is that since processes radomly pick which procedure to actually execute, you'll end up listening to just 1/3 ports at a time, randomly and chances are that noone will be able to connect.
the idea here is to listen to a single connection port and once connection is established to listen to the next available port.
Then to check for messages, you just forloop through all of the connections, checking if there're any characters/lines to read |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
loonyli
|
Posted: Thu Mar 11, 2004 3:04 pm Post subject: (No subject) |
|
|
code: | %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% This is the server code
%%%%%%%%%%%%%%%%%%%%%%%%%
var netStream : array 1 .. 3 of int
var netAddress : array 1 .. 3 of string
const netPort : array 1 .. 3 of int := init (10000, 10001, 10002)
put "Waiting for Connections..."
for i : 1 .. 3
netStream (i) := Net.WaitForConnection (netPort (i), netAddress (i))
put "Connected to ", netAddress (i), " via port ", netPort (i)
end for
put "All connections established."
%%%%%%%%%%%%%%%%%%%%%%
%this is the client code
%%%%%%%%%%%%%%%%%%%%%%%%%%
var netStream : int
var netAddress : string
const netPort : array 1 .. 3 of int := init (10000, 10001, 10002)
put "Enter address to connect to: " ..
get netAddress
for i : 1 .. 3
netStream := Net.OpenConnection (netAddress, netPort (i))
if netStream > 0 then
put "Connected to ", netAddress, " via port ", netPort (i)
exit % exit program when connected
else
put "Failed to connect to server via port ", netPort (i)
end if
if i not= 3 then
put "Trying to connect via port ", netPort (i + 1)
end if
end for
if netStream <= 0 then
put "Failed to connect to ", netAddress
end if
Time.Delay (1000)
Text.Cls
put "Press any key to exit.\nTime elapsed: "
% This loops prevents the connections from closing
loop
Text.Locate (2, 15)
put Time.Elapsed div 1000, " seconds"
exit when hasch
end loop |
This is my connection codes so far. Plz suggest changes. thx |
|
|
|
|
 |
Dan
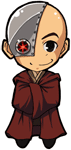
|
Posted: Thu Mar 11, 2004 10:05 pm Post subject: (No subject) |
|
|
sory i cant test your code right now b/c my network is not working gr8 right now but i do have some posable sugestions:
with the code the way it is now it has to w8 for 3 poleop to conect b4 it statras b/c the Net comand you are using is a blocking type one witch means the code stops runing till some one conects.
not what i whould do is have it w8 for just 1 person to conect and then have a processes runing that simpley checks for new poleop conecting and adds 1 to the port number and the total number of poleop.
alougth tony side not to use processes, this is one of the times where they work well if you uses them right witch is the key thing. if you do it with processes you will have to consiedr some tings like the posbality that vars could be cahging at any time and how that could mess your progame up. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
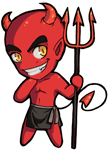
|
Posted: Fri Mar 12, 2004 12:10 am Post subject: (No subject) |
|
|
Hacker Dan wrote:
alougth tony side not to use processes, this is one of the times where they work well if you uses them right witch is the key thing.
umm... yeah sometimes you have to use processes (such as background music or in this case it might actually be a good idea to listen for new connections in a process) but in other cases there're ways of going around them.
since you don't want to have randomness in the execution of your code, a better way would be to execute handling procedures from a loop
code: |
loop
check_new_connections
check_new_messages
check_user_input
send_messages
end loop
|
having those processes execute in order would add greater stability to the program then having all 4 running simuntaniously randomly picking 1 line at a time to execute |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Dan
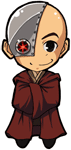
|
Posted: Fri Mar 12, 2004 11:55 am Post subject: (No subject) |
|
|
probly is that "check_new_connections " stops the loop in till there is a new connection. unless there is a non-blocking fuction that returns a boolean that there is a new contion or not. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
recneps

|
Posted: Fri Mar 12, 2004 2:24 pm Post subject: (No subject) |
|
|
or, make a procedure that checks for connection on one port, then have a for loop in a process that uses that procedure, that way its done in set order, right? |
|
|
|
|
 |
loonyli
|
Posted: Sat Mar 13, 2004 4:38 pm Post subject: (No subject) |
|
|
i tried using procedures and processes (separately), but it still doesn't seem to work. the client "thinks" it has connected and gets stuck the loop that checks if there is a line to read; meanwhile, the server is "stuck" at the Net.WaitForConnection line. i ran the prgm with the "allocation object" window open, and it said that prgm is trying to open a connection on the same port. can someone plz verify that? thx |
|
|
|
|
 |
|
|