Author |
Message |
dragun
|
Posted: Mon Mar 08, 2004 3:08 pm Post subject: vowel program help |
|
|
Hi, I was wondering if any of you can help me with this program that has been making me go crazy!
Anyways heres what i need to do:
Write a program that asks a user to enter his/her full name (first,middle, and last name) The program should inform the user of how many vowels her/his name contains. Each vowel counts as one and the "y" counts as 1/2> It should also infrom the user what percent of his/her name is made up of vowels.
Thanks for any help given. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
AsianSensation
|
Posted: Mon Mar 08, 2004 3:34 pm Post subject: (No subject) |
|
|
run a loop and use index function to search for either AEIOU. When you encountered it, add one to your counter. do an if case for Y, and add only 1/2. Percentage is just the counter divided by the total letter. |
|
|
|
|
 |
guruguru

|
Posted: Mon Mar 08, 2004 6:27 pm Post subject: (No subject) |
|
|
Here's a simple, fast way of doing it... It only checks for lower case but you can easily change that. (I have lots of spare time lol...)
code: |
var name : string
var number_vowels : real := 0
var vowel_percentage : int
put "What is your name? " ..
get name : *
for counter : 1 .. length (name)
if name (counter) = "a" or name (counter) = "e" or name (counter) = "i" or name (counter) = "o" or name (counter) = "u" then
number_vowels += 1
elsif name (counter) = "y" then
number_vowels += .5
end if
end for
vowel_percentage := round (number_vowels / length (name) * 100)
put skip, "Your name contains ", number_vowels, " vowels."
put "That is ", vowel_percentage, "% of your name."
|
(Thanks Tony ) |
|
|
|
|
 |
Tony
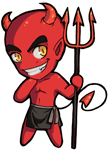
|
|
|
|
 |
dragun
|
Posted: Mon Mar 08, 2004 7:28 pm Post subject: thanks |
|
|
Hey thanks for all your help that seemed to work out great.. i wasn't quite sure how to do the index thing but thanks for the help |
|
|
|
|
 |
AsianSensation
|
Posted: Mon Mar 08, 2004 8:58 pm Post subject: (No subject) |
|
|
here's another way, it's considered more "programming-ish"
instead of using all those or statements, make a string variable with value "AEIOUaeiou" and then run index each letter of the inputted word with the vowel string variable. This saves the need to put all that or statements. This is especially useful if you are checking to see if the letter of the words belong to some group. |
|
|
|
|
 |
dragun
|
Posted: Tue Mar 23, 2004 3:21 pm Post subject: still confused |
|
|
hey, I still can't figure out how to use the index function... Also is there a way to calcualte the charcters in the Full name without counting the spaces? |
|
|
|
|
 |
sport

|
Posted: Tue Mar 23, 2004 3:43 pm Post subject: (No subject) |
|
|
Here is a way to count the name without spaces
code: |
var fullname, alpha, alpha1 : string
alpha := "abcdefghijklmnopqrstuvwxyz"
alpha1 := "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
get fullname : *
var count : int := 0
for i : 1 .. length (fullname)
if index (alpha, fullname (i .. i)) > 0 or index (alpha, fullname (i .. i)) > 0 then
count += 1
end if
end for
put count |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
dragun
|
Posted: Tue Mar 23, 2004 3:47 pm Post subject: thanks |
|
|
Thanks that helped now if someone can help me understand this vowel index function |
|
|
|
|
 |
Jodo Yodo
|
Posted: Tue Mar 23, 2004 5:13 pm Post subject: (No subject) |
|
|
When you use index, you're basically saying "Is this string inside this other string?" If it's true, index will return "1"
Thus, you can say:
if index ("a", "super")>0 then
bla bla bla
When this happens, it checks to see if "a" is in "super", and if it is, it returns "1". In this case, there are no "a"s in "super", so it only returns as 0. |
|
|
|
|
 |
Jodo Yodo
|
Posted: Tue Mar 23, 2004 5:15 pm Post subject: (No subject) |
|
|
Therefore, you can do this:
*assume other stuff and variables done*
get words
vowels:= "AEIOUaeiou"
if index ("AEIOUaeiou", words) >0 then
end if
(Words is the stuff that the user inputs, and vowelcounter counts the number of vowels)
In this case, the phrase "AEIOUaeiou" does not necessarily have to appear in this order. In fact, if only ONE of the letters in "AEIOUaeiou" is present within variables "words", then it returns "1" |
|
|
|
|
 |
Jodo Yodo
|
Posted: Tue Mar 23, 2004 5:16 pm Post subject: (No subject) |
|
|
index is pretty complicated, the whole string manipulation thing is tough. In fact, it has been a while since I did the string chapter, but I'm pretty sure I'm right.
I need bits. SUPER JUICY! |
|
|
|
|
 |
dragun
|
Posted: Tue Mar 23, 2004 5:57 pm Post subject: so if.. |
|
|
so if there is say 3 "a"'s in a word the counter will output 3?
Also if thats doesn't happen how do i add a value to the index? As i stated in the first message of this thread.......? |
|
|
|
|
 |
Jodo Yodo
|
Posted: Tue Mar 23, 2004 5:59 pm Post subject: (No subject) |
|
|
What you can do is go through each letter individually. You do this.
get word
lengthword:=length (word)
for counter:1..lengthword
if index ("AEIOUaeiou", word (counter))>0 then
wordcounter:=wordcounter+1
end if
end for |
|
|
|
|
 |
nadim
|
Posted: Tue Mar 23, 2004 6:19 pm Post subject: Hey I am also doing the same program...... |
|
|
I think that you need need something like - 3 for the spaces |
|
|
|
|
 |
|