Hangman program
Author |
Message |
Miko99
|
Posted: Fri Apr 11, 2003 9:20 pm Post subject: Hangman program |
|
|
How would i write a hangman program that asks you what word will the other user be guessing. Each time the user guesses a correct letter it will say "Yes there are 4 E's!" or "Yes there are 2 W's!" and then shows the letters and in thr right position. i really need help with this! i havn'ty even got a clue where to start! all help is appreciated. THANKS!!!! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
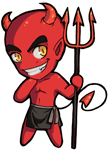
|
Posted: Fri Apr 11, 2003 9:36 pm Post subject: (No subject) |
|
|
well you use standart input/output for the word the user will be guessing.
code: |
put "what word to guess?"
get secretword
|
then you make an array of boolean to keep track of letters that were guessed.
code: |
var letter:array 1..length(secretword) of boolean
|
and initialize them all to false since none of the letters were guessed yet
then start a loop where user guesses letters
compare guessed letter with all the letters in the word
code: |
if userguess = secretword(1) %well ( i) since it will be in the loop
|
if the guess is right, mark your boolean array as true and let user know he guessed right
you can draw the letter in the correct spot using Font.Draw
there're tutorials covering everything mentioned above in the tutorials section |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Miko99
|
Posted: Sat Apr 12, 2003 12:45 pm Post subject: (No subject) |
|
|
I need more help. I dont understand how to make the boolean false. I am still lost.....PLEASE I NEED HELP!! LoL |
|
|
|
|
 |
Dan
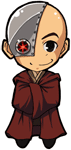
|
Posted: Sat Apr 12, 2003 2:54 pm Post subject: (No subject) |
|
|
letter(1) := false
that will make the 1st one equal to false... |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Miko99
|
Posted: Sat Apr 12, 2003 9:17 pm Post subject: (No subject) |
|
|
code:
var secretword, guess, wordguess, ans : string
put "Enter the word to Guess!"
get secretword
cls
var letter : array 1 .. length (secretword) of boolean
put "Enter a letter!"
get guess
loop
if (guess (1) := false) then
put "Sorry there are no ", guess (1), " 's"
else
put "Yes there is at least 1 ", guess (1)
end if
put "would you like to guess the word now?"
get ans
if (ans = "yes") then
put "Type in what you think the word is in Lower Case letters"
get wordguess
else
put "Ok then guess your next word!"
end if
exit when wordguess = secretword
end loop
Whats wrong with this? |
|
|
|
|
 |
Tony
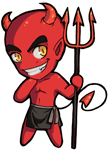
|
Posted: Sat Apr 12, 2003 9:53 pm Post subject: (No subject) |
|
|
variable guess is a string, not array. You dont need (1) after it. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Martin

|
Posted: Sat Apr 12, 2003 11:58 pm Post subject: (No subject) |
|
|
Here's how I would do it.
code: |
%The message to be guessed by the user
var strMessage : string
%The user's guess, they can only guess one letter at a time, so the variable is a string (1)
% ...that is, it is a string that can only hold 1 character rather than 255.
var strGuess : string (1)
loop
%Clear the screen
cls
%Put the cursor in the top left corner of the screen
locate (1, 1)
%Prompt the user for their guess
put "Please enter your guess"
%Getch sets the value of strGuess to the first key that the user presses
getch (strGuess)
%Now, find out if strGuess is part of strMessage
%Go through the entire message
for i : 1 .. length (strMessage)
%Read this as: If the i'th character of strMessage is the same as strGuess then...
%How this works is as follows:
%Pretend that strMessage = 'hangman' and the player guessed 'a'
%First, i = 1 so it checks the first character of strMessage [strMessage(1)]
%it then does the comparison: does 'h' = 'a'
if strMessage (i) = strGuess then
%I'll leave this part up to you
else %The i'th character is not equal to the user's guess
%Don't do anything, just check the next character until all comparisons are done
end if
end for
end loop
|
Keep in mind, this is just a shell for finding matches, it's up to you to decide what to do next.
If you don't understand, don't be afraid to ask (PM or otherwise) |
|
|
|
|
 |
Miko99
|
Posted: Sun Apr 13, 2003 11:25 am Post subject: (No subject) |
|
|
Ok, i am getting an error saying that the " ! " is an illegal Character. Is this true? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Asok

|
Posted: Sun Apr 13, 2003 1:01 pm Post subject: (No subject) |
|
|
heh miko, you seem to have the problem of not sharing your code, we cannot help you if you're not completely specific, if there is an error in your code then we need to SEE your code. |
|
|
|
|
 |
Miko99
|
Posted: Sun Apr 13, 2003 6:16 pm Post subject: (No subject) |
|
|
All thanks to Darkness, heres what i got:
Code:
var strMessage, ans, wordguess : string
var strGuess : string (1)
put "Enter the word!"
get strMessage
loop
cls
locate (1, 1)
put "Please enter your guess"
getch (strGuess)
delay (500)
for i : 1 .. length (strMessage)
if strMessage (i) = strGuess then
put "Yes! letter number ", i, " is a ", strGuess
delay (800)
else
put "No, letter number ", i, " is not a ", strGuess
delay (800)
end if
end for
put "would you like to guess the word yet?"
get ans
exit when ans = "yes"
end loop
put "Enter your guess for the word!!!!"
get wordguess
cls
if (wordguess = strMessage) then
put "Correct!!! the word was ", strMessage
else
put "HAHAHAAHAHAHA!!! YOU SUCK!!!!! THE WORD WAS ", strMessage,
" HAHAAHAHA!!!!"
end if
Now how would i modify that so that each time a letter is guessed correctly, it is displayed to the screen? |
|
|
|
|
 |
Blade
|
Posted: Sun Apr 13, 2003 6:46 pm Post subject: (No subject) |
|
|
you could make an array of which ones you've guessed... like what was said ealier... and if that letter is guessed, display it at the top of the screen...
ex:
code: | var guessed:array 1..length(word) of boolean
for i:1..length(word)
if(guessed(i) = true)then
put word(i) ..
end if |
this isnt supossed to work, its an example of what you could do
so basicly what you would do, is set each letter guessed to false and when hte player guesses a right one, its set to true and displayed at the top, but of course you'll have to organize it so its displayed correctly...
organize it by checking each letter, and if it the letter matches up.. then display it at a certian point using locate(x,y)
code: | var guessed:array 1..length(word) of boolean
for i:1..length(word)
locate(i,1)
if(guessed(i) = true)then
put word(i) ..
end if |
i think i explained it right...  |
|
|
|
|
 |
|
|