Access array without a for loop?
Author |
Message |
amz_best
|
Posted: Sat Sep 27, 2014 3:38 pm Post subject: Access array without a for loop? |
|
|
What is it you are trying to achieve?
Access an array without a for loop
What is the problem you are having?
idk how 2.
Describe what you have tried to solve this problem
tried not using a for loop. didnt work.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
nothing relevant, i just need the basic idea
Turing: |
<Add your code here>
|
Please specify what version of Turing you are using
latest version of open turing |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
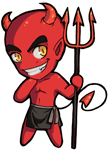
|
Posted: Sat Sep 27, 2014 5:52 pm Post subject: Re: Access array without a for loop? |
|
|
amz_best @ Sat Sep 27, 2014 3:38 pm wrote:
tried not using a for loop. didnt work.
Lets see how you've tried it. While at it, post an example that _does_ use a loop as well -- this can be used to figure out parts that are necessary, and what can be taken out. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nathan4102
|
Posted: Sat Sep 27, 2014 7:08 pm Post subject: RE:Access array without a for loop? |
|
|
If you want to iterate through the entirety of an array, you need a for loop.
If you want to access a specific index of an array, you can get away with no for loop.
Whether in a for loop or not in a for loop, the array-access code syntax is identical. |
|
|
|
|
 |
Raknarg

|
Posted: Sun Sep 28, 2014 11:06 pm Post subject: RE:Access array without a for loop? |
|
|
@nathan technically you don't need a for loop, per se... |
|
|
|
|
 |
Tony
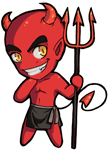
|
|
|
|
 |
Raknarg

|
Posted: Sun Sep 28, 2014 11:42 pm Post subject: RE:Access array without a for loop? |
|
|
How would you do it with recursion? |
|
|
|
|
 |
Tony
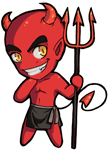
|
Posted: Mon Sep 29, 2014 3:05 am Post subject: RE:Access array without a for loop? |
|
|
writing loops using recursive calls
Quote:
$ irb
2.1.2 :001 > def go(arr)
2.1.2 :002?> if arr.any?
2.1.2 :003?> puts "item #{arr.first}"
2.1.2 :004?> go(arr[1..-1])
2.1.2 :005?> end
2.1.2 :006?> end
=> :go
2.1.2 :007 > go([1,2,3])
item 1
item 2
item 3
=> nil
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
amz_best
|
Posted: Mon Sep 29, 2014 6:47 pm Post subject: RE:Access array without a for loop? |
|
|
can someone explain to me what this recursion idea is? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
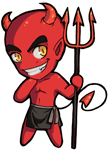
|
|
|
|
 |
wtd
|
Posted: Sat Oct 04, 2014 9:56 pm Post subject: RE:Access array without a for loop? |
|
|
When you loop in a program, what you're really doing, however the code may express it, is setting an initial state, checking for a condition, performing some set of operations, then doing it all again.
We can do this with a for loop in C.
code: | int i;
for (I = 0; I < 5; I++) {
printf("%d\n", I);
} |
And this will print the follow to stdout.
But, a function can also create an initial state, it can test for a condition, and it can process a whole series of operations.
code: | void my_function(int i) {
if (i < 5) {
printf("%d\n", i);
}
} |
And if we call the function again it can also repeat this.
code: | void my_function(int i) {
if (i < 5) {
printf("%d\n", i);
my_function(i + 1);
}
} |
Such that the following will replicate the original for loop's effects.
|
|
|
|
|
 |
wtd
|
Posted: Sat Oct 04, 2014 9:58 pm Post subject: RE:Access array without a for loop? |
|
|
Oh, oops... thought this was in C help. Ah well, concept is the same. |
|
|
|
|
 |
Dreadnought
|
Posted: Sat Oct 04, 2014 10:10 pm Post subject: Re: Access array without a for loop? |
|
|
Here's the translation into Turing.
wtd wrote:
When you loop in a program, what you're really doing, however the code may express it, is setting an initial state, checking for a condition, performing some set of operations, then doing it all again.
We can do this with a for loop in C [Turing].
Turing: | for i : 0 .. 4
put i
end for |
And this will print the following to stdout [the run window in Turing].
But, a function[a function that does not return a value is a procedure in Turing] can also create an initial state, it can test for a condition, and it can process a whole series of operations.
Turing: | procedure my_procedure (i : int)
if (i < 5) then
put i
end if
end my_procedure |
And if we call the function again it can also repeat this.
Turing: | procedure my_procedure (i : int)
if (i < 5) then
put i
my_procedure (i + 1)
end if
end my_procedure |
Such that the following will replicate the original for loop's effects.
|
|
|
|
|
 |
|
|