Help With Turing Hangman
Author |
Message |
wolfy
|
Posted: Sat Dec 07, 2013 12:34 am Post subject: Help With Turing Hangman |
|
|
Hello! I am making Hangman for a project and I am almost finished, I just have one problem. How exactly would I make it so when the user guesses a letter, and it is in the word, it displays the letter in the proper spot and adds to it each time they guess a letter. I already have an index check for if the guessed letter is in the actual word, now I just need to display where it would go. My program uses a file called "Words" that it searches for a random word to use for the game.
Turing: |
% The names of my two variables needed for this
% Word is the word
% Guess is the guessed letter
var word, guess : string
% Check that letter is in word
if index (word, guess ) > 0 then
Font.Draw (guess, 10, 100, menuFont, green)
rightLetters := rightLetters + guess
delay (1500)
Draw.FillBox (5, 80, 55, 125, black)
end if
|
If anybody could help me write the code to do this that would be a very big help, and if you may need more code to see what I would need to do, let me know. Thanks! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
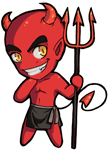
|
Posted: Sat Dec 07, 2013 1:18 am Post subject: RE:Help With Turing Hangman |
|
|
Quote:
Font.Draw (guess, 10, 100, menuFont, green)
You can try drawing the guessed letter at a position other than 10,100.
Read up on index, it will tell you where the first occurrence of the match is. You can figure out the position from there. (Also, think about the cases where the same letter appears more than once in the word). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
evildaddy911
|
Posted: Sat Dec 07, 2013 9:25 am Post subject: Re: Help With Turing Hangman |
|
|
quick question: do you know arrays? because they can make this a bit easier. if so, highlight the rest of the paragraph to read (it will confuse you a lot of you dont know arrays: think of strings as an array of single characters. you can extract single characters out of a string the same way you reference a single element of an array of integers: Int (x) gives you 2, Str (x) gives you "b"
id suggest using locate/put within a for-loop instead of Font.Draw, because then you dont have to worry about messy text overlapping and giving some strange characters.
@tony: he already is using index in the program, i would assume he already understands how to use it |
|
|
|
|
 |
Raknarg

|
Posted: Sat Dec 07, 2013 10:24 am Post subject: RE:Help With Turing Hangman |
|
|
I don't see why you would need arrays for hangman, except for the words |
|
|
|
|
 |
evildaddy911
|
Posted: Sat Dec 07, 2013 11:25 am Post subject: RE:Help With Turing Hangman |
|
|
@Ragnarg: no, not really. i was suggesting learning arrays for 2 reasons:
1) string manipulation (in turing) treats strings as arrays, so you should learn arrays. since index() only returns the first occurrence of a string pattern, if you have multiple A's in the word, for example, you should use a for loop and check every character against the guessed character. the most basic form of string manipulation.
2) im in the habit of using the basic game structure of
code: |
loop
get_input
process_input
draw_stuff
update_screen
end loop
|
which isnt bad, but would mean that you need a boolean array of "guessed" characters for redrawing purposes |
|
|
|
|
 |
Raknarg

|
Posted: Sat Dec 07, 2013 12:53 pm Post subject: RE:Help With Turing Hangman |
|
|
Fair enough. But I think that goes under more specifically string manipulation, not necessarily arrays. You can so more with strings in Turing than you can with arrays. Also, I think every language treats strings as arrays (array of characters I guess).
What? No, you can just use a string of all your guessed characters. If your letter isnt there, add it. If its there, then you cant use that word, just using index() |
|
|
|
|
 |
wolfy
|
Posted: Sat Dec 07, 2013 2:20 pm Post subject: RE:Help With Turing Hangman |
|
|
Ok thanks guys. So first off:
I do know how to use index's as I have them a few times in my code. Also I just need to make the code actually locate the proper position in the word, for example in cat. If they guess "a" i want the program to know to draw _a_.
Secondly:
I do know how to use arrays, and I have a few in my program. But they are only for things like words and letters. |
|
|
|
|
 |
evildaddy911
|
Posted: Sat Dec 07, 2013 4:36 pm Post subject: Re: Help With Turing Hangman |
|
|
If your new to string manipulation, check out this tutorial first, then come back here.
------
ok, so the basic way is to use index(). that will give you the FIRST occurrence of 'a', so you take every character in output_string before, but not including, the index, add the letter, then add everything after it (again, not including the index)
so, lets use "string" as the word and "i" as the guess. index should return 4. take the characters from 1 to 3, add 'i', then throw on characters 5 to the end. now all you have to do is output output_string (using Font.Draw or put)
something to think about: what if the word is "manipulation" and guess is "i"? what should happen? what actually happens? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wolfy
|
Posted: Sat Dec 07, 2013 7:02 pm Post subject: RE:Help With Turing Hangman |
|
|
I am more of a visual learner, is there any way you could give me a code example? Like I said above my variable for the word is word, and guess is guess. |
|
|
|
|
 |
Raknarg

|
Posted: Sat Dec 07, 2013 9:28 pm Post subject: RE:Help With Turing Hangman |
|
|
This is his example portrayed in code. It's not fully complete, but you can do that yourself. There's an easier way to do this, but this works as well.
Turing: |
proc updateHiddenWord (guess : string)
for i : 1 .. length(word ) % we look through every letter in word
if guess = word (i ) then % We found a match!
var stringBeforeI : string := hiddenWord (before_i ) % The string which is the hidden word, every letter before the index i
var stringAfterI : string := hiddenWord (after_i ) % The string which is the hidden word, every letter after the index i
hiddenWord := stringBeforeI + word (i ) + stringAfterI % We add the beginning of the hidden word, the letter we found and the end of the word
end if
end for
end updateHiddenWord
|
|
|
|
|
|
 |
wolfy
|
Posted: Sun Dec 08, 2013 11:42 pm Post subject: RE:Help With Turing Hangman |
|
|
Where does the before_i and after_i come from? |
|
|
|
|
 |
Raknarg

|
Posted: Mon Dec 09, 2013 1:17 am Post subject: RE:Help With Turing Hangman |
|
|
We're assuming you have some string hiddenWOrd that is the same length as your word, but with hidden characters.
Eg word = "hello", therefore hiddenWord = "-----" or something.
Now lets say the player guesses 'e'. So, we want hiddenWord to show 'e' now. So we go through the loop, and chance upon an 'e'. We want the rest of the string to be maintained, so we store everything that was before the 'e' and after. For instance, your hiddenWord was '----o'. Now you'll have two strings: '-' and '--o'. Throw the 'e' in the middle: '-e--o'. That is what that program would be doing. |
|
|
|
|
 |
|
|