How to define boundaries for text.
Author |
Message |
Timothy Willard
|
Posted: Thu Jun 20, 2013 2:27 pm Post subject: How to define boundaries for text. |
|
|
What is it you are trying to achieve?
I want to create a text based game, where your inventory/equipment is consistently displayed on the right, and your health/ above.
What is the problem you are having?
I do not know how to limit the text for the game (i.e. You are in a open field") to just a section in the bottom left of the screen.
Describe what you have tried to solve this problem
I tried preventing it from going too far right by making sure that anything I "put" is less than 2/3 the max column size, and it worked (code shown below). However, I don't know how to get the text to always begin below (for example) x = 100. I know of locate and locatexy, but you need to do that for every single "put" command, and it doesn't make the text stack/cycle up like it normally would.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
proc formatText (displayText : string)
var substring : string
var oneThirdMaxCol : int := maxcol div 3
if length (displayText ) > (2 * oneThirdMaxCol ) then
substring := displayText (1 .. 2 * oneThirdMaxCol )
put substring
put displayText ((2 * oneThirdMaxCol ) + 1 .. length (displayText ))
put "Too big."
end if
|
(Please note that "Too big" is just for bugtest purposes)
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
evildaddy911
|
Posted: Thu Jun 20, 2013 2:48 pm Post subject: RE:How to define boundaries for text. |
|
|
well, if you know of locatexy, then the only thing i can suggest is Font.Draw (text, x, y, font, color).
You seem to understand how to wrap text, however, i hope you realize that doing it that way will sometimes split the text in the middle of the word |
|
|
|
|
 |
Tony
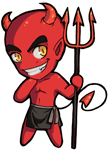
|
Posted: Thu Jun 20, 2013 2:56 pm Post subject: Re: How to define boundaries for text. |
|
|
Timothy Willard @ Thu Jun 20, 2013 2:27 pm wrote: but you need to do that for every single "put" command
That is the wrong approach anyway. Not because "I don't feel like pasting in some code all over the place", but because "pasting in some code all over the place" is simply a bad idea. What happens when you decide to adjust the UI to change "x" from 100 to 150?
You are obviously familiar with functions, so that's what you should do -- implement a function to handle the placing of the text.
Instead of
you'll use
code: |
yourAwesomeFunction("some text")
|
and the latter will contain a single spot in the codebase that will keep track of amount of text, locatexy coordinates, etc. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Timothy Willard
|
Posted: Thu Jun 20, 2013 3:41 pm Post subject: Re: How to define boundaries for text. |
|
|
Actually, I started using a function of my own just before you posted, and I have it finished here.
Turing: | proc shiftText
for i : 1 .. 69
previousText (i ) := previousText (i + 1)
end for
end shiftText
proc customPut (userInput : string)
cls
shiftText
previousText (70) := userInput
for i : 1 .. 70
locate (4 + i, 1)
put previousText (i )
end for |
It works, but rather than nicely scrolling the text up like standard, it 'blinks' up. I don't know of any way of moving the text up beyond just rewriting it.
Edit: I found that the blinking is caused by using cls. The problem is that if I remove that, the old text scrolls up using standard Turing code, and I don't get my space above my text. Is there a way to disable that "scrolling" in Turing? |
|
|
|
|
 |
andrew.
|
Posted: Fri Jun 21, 2013 9:12 am Post subject: RE:How to define boundaries for text. |
|
|
I haven't done Turing in a long time, but to eliminate the flashing on the screen for each screen update, you have to "double-buffer" the screen.
What this means is that you basically draw everything, then display it to the screen rather than having Turing draw everything as it goes through your code.
To do this, you have to tell Turing that you want it to double buffer by calling the View.Set method with "offscreenonly" as a parameter.
Then when you want Turing to draw everything to the screen, you call View.Update (I believe).
It should look something like this (my syntax may be wrong):
|
|
|
|
|
 |
Raknarg

|
Posted: Fri Jun 21, 2013 6:18 pm Post subject: RE:How to define boundaries for text. |
|
|
To add, for a small speed boost, use View.UpdateArea (0, 0, maxx, maxy) instead of View.Update |
|
|
|
|
 |
|
|