Turing Procedure Problems
Author |
Message |
timmy otoole
|
Posted: Wed May 02, 2012 10:25 am Post subject: Turing Procedure Problems |
|
|
Hi, I'm having problems with two things in this lottery program I'm making for class. First, I keep getting an error message in my sorting procedure that "the variable has no value." Code(beneath Winningnums in actual program):
for j : 1 .. 5
for i : 1 .. 5
if nums (i) < nums (i + 1) then
temp := nums (i)
nums (i) := nums (i + 1)
nums (i + 1) := temp
end if
end for
end for
Second, the program says procedure lotto can only be declared at the "program,module or monitor level." Also, although it's probably obvious, I'm new to programming.
PROGRAM
type box :
record
x, y, sx, sy, col : int
end record
var font3 : int := Font.New ("arial:20:bold,italic")
var font4 : int := Font.New ("arial:12:bold,italic")
var x : int
var font : int := Font.New ("arial:16:bold,italic")
var font1 : int := Font.New ("arial:12:bold,italic")
var font2 : int := Font.New ("arial:16:bold,italic")
var money : int := 30
var moneys : string
var curX, curY : int := 75
var boxes : array 1 .. 42 of box
var numbers : array 1 .. 6 of int
var z, numChosen : int
numChosen := 0
var Winnings : array 0 .. 6 of int := init
(0, 5, 10, 100, 500, 1000, 10000)
var num : array 1 .. 6 of int
curX := maxx div 2 - 100
type car :
record
x : int
y : int
speed : int
end record
var cars : array 1 .. 2 of car
var y : int := maxy - 125
for i : 1 .. 2
cars (i).x := 0
cars (i).y := y
cars (1).speed := 5
cars (2).speed := 4
y -= 125
end for
setscreen ("offscreenonly")
function between (y, minpos, maxpos : int) : boolean
if y < maxpos and y > minpos then
result true
end if
result false
end between
function Move (x : int, xory : boolean, var myCar : car) : boolean
if xory then
if whatdotcolour (myCar.x - x, myCar.y) not= gray then %iSuperRages
myCar.x -= x
result true
end if
elsif whatdotcolor (myCar.x, myCar.y - x) not= gray and myCar.y > 0 and myCar.y < maxy then % iRaged
myCar.y -= x
result true
end if
result false
end Move
function ptinrect (x, y, x1, y1, x2, y2 : int) : boolean
result (x > x1) and (x < x2) and (y > y1) and (y < y2)
end ptinrect
procedure resetLotteryValues
for i : 1 .. 42
boxes (i).x := curX
boxes (i).y := curY
boxes (i).sx := curX + 20
boxes (i).sy := curY + 20
boxes (i).col := black
curX += 25
if curX > maxx div 2 + 50 then
curX := maxx div 2 - 100
curY += 25
end if
end for
end resetLotteryValues
procedure drawLottery
for i : 1 .. 42
drawbox (boxes (i).x, boxes (i).y, boxes (i).sx, boxes (i).sy, boxes (i).col)
Font.Draw (intstr (i), boxes (i).x + 5, boxes (i).y + 5, Font.New ("Arial:8"), boxes(i).col)
end for
end drawLottery
function grid (random : boolean) : array 1 .. 6 of int
View.Set ("offscreenonly")
resetLotteryValues
drawLottery
View.Update
if not random then
loop
Mouse.Where (x, y, z)
if z not= 0 then
cls
for i : 1 .. 42
if ptinrect (x, y, boxes (i).x, boxes (i).y, boxes (i).sx, boxes (i).sy) and boxes (i).col not= 43 then
boxes (i).col := 43
numChosen += 1
if numChosen <= 6 then
numbers (numChosen) := i
end if
end if
end for
drawLottery
View.Update
end if
exit when numChosen >= 6
end loop
else
for i : 1 .. 6
loop
var randNum : int := Rand.Int (1, 42)
if boxes (randNum).col not= 43 then
boxes (randNum).col := 43
numbers (i) := randNum
exit
end if
end loop
drawLottery
View.Update
Time.Delay(500)
cls
end for
end if
View.Set ("nooffscreenonly")
result numbers
end grid
var Input : int := 1 % times
loop
var ch : array char of boolean
var worked : boolean
Input.KeyDown (ch)
if ch (KEY_LEFT_ARROW) then
worked := Move
(cars (1).speed, true, cars (1))
elsif ch (KEY_RIGHT_ARROW) then
worked := Move
(cars (1).speed * -1, true, cars (1))
elsif ch (KEY_UP_ARROW) then
worked := Move
(cars (1).speed * -1, false, cars (1))
elsif ch (KEY_DOWN_ARROW) then
worked := Move
(cars (1).speed, false, cars (1))
end if
if not Move (cars (2).speed * -1, true, cars (2)) then
if cars (2).x > 350 and cars (2).x < 400 then
worked := Move (cars (2).speed, false, cars (2))
else
worked := Move (cars (2).speed * -1, false, cars (2))
end if
end if
%cars (2).x += cars (2).speed
procedure lotto
Draw.FillBox (80, 120, 205, 220, green)
Draw.FillBox (250, 120, 375, 220, green)
Draw.FillBox (420, 120, 545, 220, green)
Font.Draw ("Welcome to Ryan Hodges's Lottery!", 100, 320, font3, black)
Font.Draw ("Play", 460, 170, font4, black)
Font.Draw ("Exit", 290, 170, font4, black)
Font.Draw ("Info", 120, 170, font4, black)
end lotto
loop
Mouse.Where(x,y,z)
if z not=0 then
if ptinrect(x,y,80,120,205,220) then
Draw.Fill (maxx, maxy, red, red)
Font.Draw ("These are the rules:", 20, 350, font3, black)
Font.Draw ("1.Control your car with the arrow keys, win the race to choose your numbers", 20, 310, font4, black)
Font.Draw ("2.If the computer wins, it chooses your numbers for you.", 20, 270, font4, black)
Font.Draw ("3.Choose six lottery numbers from 1 to 42.", 20, 230, font4, black)
Font.Draw ("4.You start with $10. Each ticket costs $5.", 20, 190, font4, black)
Font.Draw ("5.Get matches to earn money! One match=$5, Two=$20, Three=$100,Four=$500", 20, 150, font4, black)
Font.Draw ("Five=$1000,Six=$10000.", 40, 130, font4, black)
delay(5000)
cls
lotto
elsif ptinrect(x,y,250,120,375,220) then
cls
elsif ptinrect(x,y,420,120,545,220) then
cls
Font.Draw("3",maxx div 2,maxy div 2, font, yellow)
delay(1000)
cls
Font.Draw("2",maxx div 2,maxy div 2,font, yellow)
delay(1000)
cls
Font.Draw("1",maxx div 2,maxy div 2, font,yellow)
delay(1000)
cls
Font.Draw("GO!", maxx div 2,maxy div 2,font,yellow)
cls
Draw.FillBox (400, 220, 370, 400, gray)
Draw.FillBox (400, 0, 370, 150, gray)
Draw.FillBox (200, 50, 220, 350, gray)
Draw.FillBox (550, 50, 570, 350, gray)
Draw.FillBox (90, 0, 110, 170, gray)
Draw.FillBox (90, 200, 110, 400, gray)
Draw.FillBox (0, 400, maxx, 390, gray)
Draw.FillBox (0, 0, maxx, 10, gray)
for i : 1 .. 2
Draw.FillOval (cars (i).x, cars (i).y, 2, 2, blue)
end for
View.Update
exit when
cars (1).x > maxx or
cars (2).x > maxx
delay (25)
end if
cls
setscreen ("nooffscreenonly")
var nums, winningNums : array 1 .. 6 of int
cls
if cars (1).x > maxx then
% cls
Font.Draw ("You Won! Pick Your Numbers(6)", 170, 300, font2, black)
nums := grid (false)
% for i : 1 .. 6
%
% loop
% locatexy (300, 200)
% get nums (i)
% var complete : boolean := true
% for j : 1 .. i - 1
% if nums (j) = nums (i) then
% complete := false
% end if
% end for
% exit when (nums (i) <= 42 or nums (i) >= 1) and complete
% put "That needs to be between 1 and 42, please reenter."
% end loop
% end for
cls
elsif cars (2).x > maxx then
% cls
Font.Draw ("You Lost The computer picks your numbers", 180, 260, font1, black)
nums := grid (true)
% for i : 1 .. 6
% loop
% nums (i) := Rand.Int (1, 42)
%
% var complete : boolean := true
% for j : 1 .. i - 1
% if nums (j) = nums (i) then
% complete := false
% end if
% end for
%
% exit when complete
% end loop
% end for
end if
for i : 1 .. 6
loop
winningNums (i) := Rand.Int (1, 42)
var complete : boolean := true
for j : 1 .. i - 1
if winningNums (j) = winningNums (i) then
complete := false
end if
end for
exit when complete
end loop
end for
var temp : int := 0
for j : 1 .. 5
for i : 1 .. 5
if winningNums (i) < winningNums (i + 1) then
temp := winningNums (i)
winningNums (i) := winningNums (i + 1)
winningNums (i + 1) := temp
end if
end for
end for
for j : 1 .. 5
for i : 1 .. 5
if nums (i) < nums (i + 1) then
temp := nums (i)
nums (i) := nums (i + 1)
nums (i + 1) := temp
end if
end for
end for
cls
drawfill(5, 5, 105, 105)
locatexy (270, 200)
for i : 1 .. 6
put nums (i), " " ..
end for
put " "
locatexy (270, 180)
for i : 1 .. 6
put winningNums (i), " " ..
end for
put ""
var matches : int := 0
for j : 1 .. 6
for i : 1 .. 6
if nums (i) = winningNums (j) then
matches += 1
end if
end for
end for
locatexy (270, maxy div 2 + 25)
if matches = 1 then
Font.Draw("You got 1 match.",270,maxy div 2+25, font,black) % Make this into Font.Draw
% And this
end if
money += Winnings (matches)
Font.Draw ("You have this much money:" + intstr (money), 170, 300, font, black)
end if
end loop
end loop |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dreadnought
|
Posted: Wed May 02, 2012 11:48 am Post subject: Re: Turing Procedure Problems |
|
|
You assign a value (or values) to nums in an if statement. What happens when the conditions of the if statement are not satisfied? |
|
|
|
|
 |
kane8290
|
Posted: Thu May 03, 2012 8:08 pm Post subject: RE:Turing Procedure Problems |
|
|
Well your first problem is that you have "%"'s in front of many pieces of code, so they will not be acted upon  |
|
|
|
|
 |
Raknarg

|
Posted: Fri May 04, 2012 10:08 am Post subject: RE:Turing Procedure Problems |
|
|
Sometimes people do that because they dont want to erase it, but also dont want it to be used right now |
|
|
|
|
 |
Tony
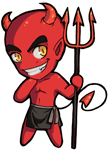
|
Posted: Fri May 04, 2012 12:24 pm Post subject: Re: RE:Turing Procedure Problems |
|
|
Raknarg @ Fri May 04, 2012 10:08 am wrote: Sometimes people do that because they dont want to erase it, but also dont want it to be used right now
that's what code versioning systems are for (e.g. Git) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Raknarg

|
Posted: Fri May 04, 2012 10:37 pm Post subject: RE:Turing Procedure Problems |
|
|
Yeah, but what if its just for a bit? Sometimes I do that, but then forget about the code I commented. |
|
|
|
|
 |
|
|