Turing GUI help needed
Author |
Message |
compnerd101
|
Posted: Thu Dec 29, 2011 9:32 pm Post subject: Turing GUI help needed |
|
|
I am creating a program that makes rectangles on the page at random locations when a button labeled "Draw Rectangle is pressed"
The following is my code
_________________________________________________________________________________
import GUI
procedure rectangle(startX:int, startY:int, endX:int, endY:int, color:int)
put "Please enter how far to the right you wisk to start the rectangle:" .. get startX
put "Please enter how far from the botttom you wish to start the rectangle:" .. get startY
put "Please enter the width of the rectangle to draw:".. get endx
put "Please enter the height of the rectangle to draw:".. get endY
put "Please enter the color of the rectangle (0-255 are valid options):".. get color
end rectangle
put "Press the draw button to create a rectangle of your choice."
GUI.CreateButton(30,15,5,"Draw Rectangle", rectangle)
___________________________________________________________________________
When i try to run the code i get the following errors:
-In the procedure "rectangle", on each line when i ask for user input, i get the error, "illegal get item"
I've tried for hours to fix, thinking that it was a matter of syntax, but to no avail.
-In the last line of code where there is the GUI.Createbutton, i get the error, "Arguement is of wrong type", with the rectangle reference highlighted
To try and solve this ive tried to make the switch around the variable types in the rectangle proc, but again, it didnt work |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
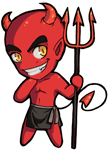
|
Posted: Thu Dec 29, 2011 9:38 pm Post subject: RE:Turing GUI help needed |
|
|
A hint for you:
you are calling procedure "rectangle", but the one declared (and thus expected) is "rectangle(int,int,int,int,int)" |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
compnerd101
|
Posted: Thu Dec 29, 2011 11:35 pm Post subject: Re: RE:Turing GUI help needed |
|
|
Tony @ Thu Dec 29, 2011 9:38 pm wrote: A hint for you:
you are calling procedure "rectangle", but the one declared (and thus expected) is "rectangle(int,int,int,int,int)"
i tried fixing by adding the space, but again, to no avail.and even if that were the case, wouldn't there be an error at the end procedure line, since the compiler would expect the statement to be "end rectangle(int,int,int,int,int)", instead of just "end rectangle"? |
|
|
|
|
 |
Tony
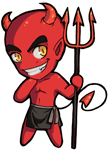
|
Posted: Thu Dec 29, 2011 11:50 pm Post subject: RE:Turing GUI help needed |
|
|
I was talking about
code: |
GUI.CreateButton(30,15,5,"Draw Rectangle", rectangle)
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Dreadnought
|
Posted: Fri Dec 30, 2011 12:07 am Post subject: Re: Turing GUI help needed |
|
|
When we declare a procedure like this
Turing: | procedure MyProcedure (param1, param2, param3, param4 : int)
% MyProcedure stuff
end MyProcedure |
We are creating a procedure that expects 4 parameters (integers in this case). Parameters are values that the procedure requires in order to run.
If we wanted to call this procedure we would do so in this way:
Turing: | MyProcedure (arg1, arg2, arg3, arg4) |
We have given the procedure four arguments to satisfy the procedure's parameters. (These arguments must all be integers in this case)
These arguments can now be used as constant values in the body of the procedure. Since they are constants we cannot change their value in any way.
If we wanted to use them as variables we would declare our procedure like so:
Turing: | procedure MyProcedure (var param1, param2, param3, param4 : int)
% MyProcedure stuff
end MyProcedure |
But this is not what you want.
To add to Tony's hint, remember that get changes the value of the variable it is given.
Also, you can declare variables within a procedure in the same way that you would outside of a procedure, just remember that they will only exist within the procedure they were created in. |
|
|
|
|
 |
compnerd101
|
Posted: Fri Dec 30, 2011 12:49 am Post subject: RE:Turing GUI help needed |
|
|
i got most of the program working. dreadnought, thanks for telling me i could declare variables in proc's same as out of. thats what was messing me up. here is an updated version of my code:
_____________________________________
import GUI
procedure Rectangle
var startX, startY, endX, endY, colors : int
put "Please enter how far to the right you wish to start the rectangle:"
get startX
put "Please enter how far from the botttom you wish to start the rectangle:"
get startY
put "Please enter the width of the rectangle to draw:"
get endX
put "Please enter the height of the rectangle to draw:"
get endY
put "Please enter the color of the rectangle (0-255 are valid options):"
get colors
Draw.Box(startX,startY,endX,endY, colors)
GUI.Refresh
end Rectangle
put "Press the draw button to create a rectangle of your choice."
var drawRec : int := GUI.CreateButton (50, 15, 5, "Draw Rectangle", Rectangle)
var Quit:int:= GUI.CreateButton (200, 15,5, "Quit", GUI.Quit)
loop
exit when GUI.ProcessEvent
end loop
_____________________________________
now, another question, if i may: what purpose does the last 3 lines of code serve? i just copy pasted it from the holtsoft doc because it says it is common to use it to get widgets working. |
|
|
|
|
 |
Tony
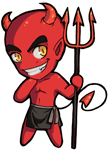
|
Posted: Fri Dec 30, 2011 1:25 am Post subject: RE:Turing GUI help needed |
|
|
without it, the buttons are just drawings that do nothing (since no button code is running). You have only a single thread, so your program goes into that "infinite loop" (until quit), and control is given to the button code.
Another interesting way to look at it, is if you replace it with
code: |
put "starting up GUI"
for i: 1 .. 10000
if GUI.ProcessEvent then
put "Quit button was pressed"
end
end
put "GUI no longer works"
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
compnerd101
|
Posted: Fri Dec 30, 2011 1:55 am Post subject: RE:Turing GUI help needed |
|
|
ok. i realize this is becoming more of a question thread then anything else, but is there a "cls when" type of command in turing, where statements dissapeer when a certain condition is met? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
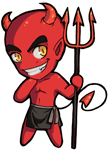
|
Posted: Fri Dec 30, 2011 2:06 am Post subject: RE:Turing GUI help needed |
|
|
code: |
if <condition> then
% do whatever you want
% e.g. -- cls
end if
|
keep in mind that you can't just throw random pieces of "if-this-than-that" code into a pile, and expect anything to work. There's a linear control flow -- one statement at a time. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
compnerd101
|
Posted: Fri Dec 30, 2011 2:08 am Post subject: RE:Turing GUI help needed |
|
|
ok thank you. |
|
|
|
|
 |
|
|