Making an animation walk fluidly
Author |
Message |
Aange10

|
Posted: Wed Oct 12, 2011 8:47 pm Post subject: Making an animation walk fluidly |
|
|
What is it you are trying to achieve?
Squares are for losers! I'm going to implement a person (stick man) into my game! ... Are there any basic ways to go about drawling him? I know that A: if i make his legs move every frame then he's going to be moving 26 times a second (35 ms delay), so that won't look right. So I'm wondering, how do people normally go about doing this?
What is the problem you are having?
Well if my character's legs moved 26 times a second, it's barley noticeable. I could make 26 pictures and cycle through them to make him "walk" every second. But if I want him to go backwards as well that 52 photos. I know this can't be memory efficient in the long run; every mob in a game can't do this...
Describe what you have tried to solve this problem
Brainstorm, mostly. Asking some friends what they think as well
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
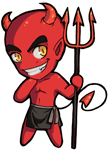
|
Posted: Wed Oct 12, 2011 9:59 pm Post subject: RE:Making an animation walk fluidly |
|
|
The term is "sprite sheet". Games do just cycle through the images that are assembled into animations. Search for some sample sheets do get an idea of what kind of character positions they cover -- you'll notice that walking is typically just a few frames. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
trishume

|
Posted: Thu Oct 13, 2011 7:12 am Post subject: Re: Making an animation walk fluidly |
|
|
You can use Time.Elapsed or some other timing function to only move the person's legs when you want to.
If you incorporate Time.Elapsed throughout your game it will run at the same speed always.
Turing's frame rate is not always 27fps it varies depending on how fast the computer is.
A common problem with games that don't time anything is that they are much faster/harder on fast computers. |
|
|
|
|
 |
Aange10

|
Posted: Thu Oct 13, 2011 7:19 am Post subject: Re: Making an animation walk fluidly |
|
|
trishume wrote:
You can use Time.Elapsed or some other timing function to only move the person's legs when you want to.
If you incorporate Time.Elapsed throughout your game it will run at the same speed always.
Turing's frame rate is not always 27fps it varies depending on how fast the computer is.
A common problem with games that don't time anything is that they are much faster/harder on fast computers.
Yes, Time.Elapsed could suffice. I may switch my method to that, if need be. However, the game can't go faster than 27 fps. That is because of the delay I have on the game, at the bottom of the loop. Considering the game is written in turing, most people can run 27 fps+ on it. However I do have a friend who tested it, he has a really bad computer and he was getting 23 fps.
If you know of a good way to please both 23 fpsers and 27 fpsers, then feel free to let me know. Do you think that the delay in time instead of in frames (how i have it) would help those with slow computers? |
|
|
|
|
 |
Insectoid

|
Posted: Thu Oct 13, 2011 9:50 am Post subject: RE:Making an animation walk fluidly |
|
|
Just don't ever use delay(). What I like to do is calculate the time elapsed since the previous frame, and move everything an appropriate distance for that time. In this way, everything moves in pixels-per-second rather than pixels-per-frame, so slow computers will run at the same 'speed, but it will be choppy, whereas high-end computers will run it very smoothly. This gets complicated really fast though. |
|
|
|
|
 |
Tony
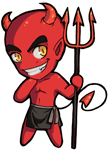
|
Posted: Thu Oct 13, 2011 10:00 am Post subject: Re: RE:Making an animation walk fluidly |
|
|
Insectoid @ Thu Oct 13, 2011 9:50 am wrote: This gets complicated really fast though.
That's been abstracted away into Time.DelaySinceLast |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Thu Oct 13, 2011 10:29 am Post subject: Re: RE:Making an animation walk fluidly |
|
|
Tony @ Thu Oct 13, 2011 10:00 am wrote: Insectoid @ Thu Oct 13, 2011 9:50 am wrote: This gets complicated really fast though.
That's been abstracted away into Time.DelaySinceLast
Not really. Time.DelaySinceLast() limits the framerate so you'll get the same fps on any machine (with the exception of very slow computers). I was suggesting simply drawing more frames instead of waiting. Limiting the framerate is (as far as I know) only useful for preventing screen tearing (also it's a lot easier to do 'cause you just change one line in the loop).
Maybe one day I'll get a super-fast computer of the future that does things way faster than computers now, and my games will run at 10,000 fps without changing a single line of code. |
|
|
|
|
 |
Tony
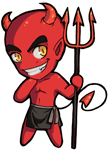
|
Posted: Thu Oct 13, 2011 1:10 pm Post subject: RE:Making an animation walk fluidly |
|
|
Well, right... DelaySinceLast slows down fast machines such that all have the same FPS. Depending on the application, you might get smoother animations with more FPS... but at the velocities below ~1px per (time delayed) frame both approaches would look identical (as drawing a frame in the same pixel position multiple times would not advance anything)
Some common FPS rates:
- standard tv: 30
- hd tv: 60 (and typical LCD computer monitors)
commercial video games tend to have an FPS somewhere in between. There are games that will compute more than 60 frames each second, though since the hardware will not be able to display that many frames that fast anyway, the benefit is negligible. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
mirhagk
|
Posted: Thu Oct 13, 2011 1:53 pm Post subject: RE:Making an animation walk fluidly |
|
|
Drawing should never occur more than 60 times a second, it's simply a waste, the human eye can't notice a difference. However the physics, movement etc could update more than that, but again, if the game is designed to work fine at 60 frames, having more frames won't make it work much better, and it will just eat CPU, overheating and taken time processing time away from other applications.
Realistically, unless a game has some advanced processing it can do with extra time (for instance loading another level, or spend more time on AI or something), it should relinquish the CPU after it's done, and wait until the next frame comes up. (and certain functions should not even be updated 60 times a second, for instance do AI need to have a 16ms repsonse time? Usually not, so you can update them less often, like 20 times a second or less) |
|
|
|
|
 |
Beastinonyou

|
Posted: Fri Oct 14, 2011 5:40 pm Post subject: Re: Making an animation walk fluidly |
|
|
I recently (very recently) asked the same question, and I suggest taking a look at these in the Documentation:
Pic.FileNewFrames and Sprite.Animate.
The two are used in the example for Sprite.Animate, and I believe that those should help... Helped me anyway. =P |
|
|
|
|
 |
Aange10

|
Posted: Fri Oct 14, 2011 5:56 pm Post subject: RE:Making an animation walk fluidly |
|
|
Yes I've been reading, beast! And thank you for the post, I'll definitely check those two out. If your wondering, i simply asked in a new thread because your knowledge of character animation seemed to be more than mine, so the answers you received deemed useless to me. (At least, for the current point in time) Hence, i made a new thread with a lower level question. Just notably the same topic (: |
|
|
|
|
 |
|
|