Game Keeps Replaying
Author |
Message |
darkage43
|
Posted: Wed Jun 15, 2011 6:25 pm Post subject: Game Keeps Replaying |
|
|
What is it you are trying to achieve?
Trying not to let the screen go back into playing the game.
What is the problem you are having?
Every time the screen shows you lose or you win the screen would then go back into playing the game. It only stops if I hit the quit button.
Describe what you have tried to solve this problem
I don't know what to do
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
import GUI
var winID : int
winID := Window.Open ("graphics:640;400")
%Setting Variables
var ballx, bally : int := 200 %Coordinates for the ball
var x, y : int := 1 %Speed and direction of the ball
var box : int := 170 %Y-coordinates of pong paddle
var chars : array char of boolean %Allows the usage of keys
var startTime : int := Time.Elapsed %Gets the current time so we know when the game started
var score : int
var xmouse, ymouse, button : int
var font := Font.New ("Impact:26")
var font2 := Font.New ("Arial:12")
var font3 := Font.New ("Sans Serif:40:bold")
var pic : int := Pic.FileNew ("ping-pong.jpg")
var pic2 : int := Pic.FileNew ("win.gif")
var pic3 : int := Pic.FileNew ("the_gameover.jpg")
%Title
Pic.Draw (pic, - 25, - 35, 0)
Font.Draw ("POWERPong", 30, 310, font3, 53)
Font.Draw ("POWERPong", 30, 305, font3, 54)
Font.Draw ("POWERPong", 30, 300, font3, 55)
procedure instruction
Font.Draw ("1. Use up and down arrow key to move", 30, 250, font2, black)
Font.Draw ("2. Last 60 seconds with POWERPong and you'll win!", 30, 200, font2, black)
Font.Draw ("3. Remember: The ball comes towards you much faster", 30, 150, font2, black)
Font.Draw ("4. Hold down the left mouse button to see instructions again in game", 30, 100, font2, black)
end instruction
%Game
procedure game
loop
View.Set ("offscreenonly") %Updates window offscreen which causes the animation
Input.KeyDown (chars ) %allows the use of the keyboard to control the paddle
mousewhere (xmouse, ymouse, button ) %Determines the location of the mouse
const BUTTON_X1 := 1280
const BUTTON_Y1 := 800
const BUTTON_X2 := 640
const BUTTON_Y2 := 400
drawbox (BUTTON_X1, BUTTON_Y1, BUTTON_X2, BUTTON_Y2, 15)
Draw.FillBox (0, 0, maxx, maxy, black) %Background colour
drawfillbox (10, box, 20, box + 95, red) %Pong paddle
drawfilloval (ballx, bally, 6, 6, white) %Pong ball
delay (3) %Slows down the speed of the ball
if chars (KEY_UP_ARROW) then %Paddle movement keys
box + = 2
elsif chars (KEY_DOWN_ARROW) then
box - = 2
end if
%Pong Ball
ballx := ballx + x %Causes the ball to move around the screen automatically
bally := bally + y
if bally >= 390 then %Creates a top boundary for the ball
y := - 1 %Causes the ball to bounce back against the border
elsif bally <= 10 then %Creates a bottom boundary for the ball
y := 1
elsif ballx >= 635 then %Creates a right boundary for the ball
x := - 3
end if
%Pong Paddle
if box >= 320 then %Creates a top boundary for the paddle
box := 320
elsif box <= 0 then %Creates a bottom boundary for the paddle
box := 0
end if
if ballx <= 30 and bally > box and bally < box + 95 then %Causes the ball to bounce off the paddle
x := 1
end if
%Score
locate (1, 70)
put "Time:"
locate (1, 78)
colourback (black)
colour (yellow)
score := Time.Elapsed %Gets the time each time the loop repeats
put (score - startTime ) div 1000 %Slows down the timer by 1 second
%Instruction
if button = 1 then
Font.Draw ("1. Use the up and down arrow key to move", 150, 225, font2, yellow)
Font.Draw ("2. Last 60 seconds with POWERPong and you'll win!", 150, 200, font2, yellow)
Font.Draw ("3. Remember: The ball comes towards you much faster", 150, 175, font2, yellow)
end if
%Win/Lose
if ballx <= 0 then
cls
Draw.FillBox (0, 0, maxx, maxy, 0)
Pic.Draw (pic3, 180, 65, 0)
var quitBtn : int := GUI.CreateButton (550, 15, 0, "Quit", GUI.Quit)
exit when GUI.ProcessEvent
elsif (score - startTime ) div 1000 = 60 then
cls
Draw.FillBox (0, 0, maxx, maxy, 0)
Pic.Draw (pic2, 150, 25, 0)
var quitBtn : int := GUI.CreateButton (550, 15, 0, "Quit", GUI.Quit)
exit when GUI.ProcessEvent
end if
View.Update %Updates window offscreen
end loop
end game
var c : int := GUI.CreateButton (450, 20, 0, "Instruction", instruction )
var b : int := GUI.CreateButton (385, 20, 0, "Start", game )
var quitBtn : int := GUI.CreateButton (550, 20, 0, "Quit", GUI.Quit)
loop
exit when GUI.ProcessEvent %Exits game once quit button is pressed
end loop
Window.Close (winID ) %Closes game window
|
Please specify what version of Turing you are using
4.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
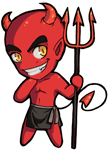
|
Posted: Wed Jun 15, 2011 6:37 pm Post subject: Re: Game Keeps Replaying |
|
|
darkage43 @ Wed Jun 15, 2011 6:25 pm wrote: It only stops if I hit the quit button.
Is there any other way to exit that loop? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ProgrammingFun

|
Posted: Wed Jun 15, 2011 6:39 pm Post subject: Re: Game Keeps Replaying |
|
|
You are not telling it to exit after displaying the message unless the button is pressed. |
|
|
|
|
 |
darkage43
|
Posted: Wed Jun 15, 2011 6:46 pm Post subject: RE:Game Keeps Replaying |
|
|
What I'm actually trying to do is when the game ends, the user still has the ability to click quit. I tried adding this to the program.
Turing: |
exit when ballx <=0 or (score - startTime) div 1000 = 60
|
However this stops the game, which means it doesn't show the you win, you lose, or the quit button. |
|
|
|
|
 |
darkage43
|
Posted: Wed Jun 15, 2011 7:50 pm Post subject: Re: Game Keeps Replaying |
|
|
ProgrammingFun @ Wed Jun 15, 2011 wrote: You are not telling it to exit after displaying the message unless the button is pressed.
How would I do that then? |
|
|
|
|
 |
ProgrammingFun

|
Posted: Wed Jun 15, 2011 7:54 pm Post subject: RE:Game Keeps Replaying |
|
|
If you want the person to only click "Quit" afterwards, you can add a long delay after exit when GUI.ProcessEvent in both cases here:
Turing: |
if ballx <= 0 then
cls
Draw.FillBox (0, 0, maxx, maxy, 0)
Pic.Draw (pic3, 180, 65, 0)
var quitBtn : int := GUI.CreateButton (550, 15, 0, "Quit", GUI.Quit)
exit when GUI.ProcessEvent
elsif (score - startTime ) div 1000 = 60 then
cls
Draw.FillBox (0, 0, maxx, maxy, 0)
Pic.Draw (pic2, 150, 25, 0)
var quitBtn : int := GUI.CreateButton (550, 15, 0, "Quit", GUI.Quit)
exit when GUI.ProcessEvent
end if
|
OR, you can declare a boolean variable at the beginning and run the game inside a while loop so that this variable is changed to exit the game when the user presses QUIT here ^. |
|
|
|
|
 |
darkage43
|
Posted: Wed Jun 15, 2011 8:11 pm Post subject: RE:Game Keeps Replaying |
|
|
When I add a long delay after GUI.ProcessEvent it also delays the time that it takes to appear the the button. |
|
|
|
|
 |
Tony
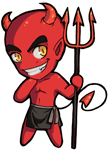
|
Posted: Wed Jun 15, 2011 8:15 pm Post subject: Re: RE:Game Keeps Replaying |
|
|
darkage43 @ Wed Jun 15, 2011 8:11 pm wrote: When I add a long delay after GUI.ProcessEvent it also delays the time that it takes to appear the the button.
darkage43 @ Wed Jun 15, 2011 6:25 pm wrote: View.Update %Updates window offscreen |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
darkage43
|
Posted: Wed Jun 15, 2011 8:25 pm Post subject: RE:Game Keeps Replaying |
|
|
So there is some kind of problem where I'm placing the View.Update? |
|
|
|
|
 |
Tony
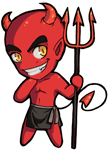
|
Posted: Wed Jun 15, 2011 8:58 pm Post subject: RE:Game Keeps Replaying |
|
|
What happens when you place a delay before View.Update? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ProgrammingFun

|
Posted: Wed Jun 15, 2011 9:27 pm Post subject: RE:Game Keeps Replaying |
|
|
Oh yes, my bad...as Tony said, place it after View.Update  |
|
|
|
|
 |
darkage43
|
Posted: Thu Jun 16, 2011 12:14 pm Post subject: RE:Game Keeps Replaying |
|
|
Oh, alright I got it now. Thanks for the help. |
|
|
|
|
 |
|
|