Author |
Message |
sparkz1
|
Posted: Thu Apr 28, 2011 4:42 pm Post subject: Find if its a odd or even number |
|
|
What is it you are trying to achieve?
<Writing a program in turing that gets a number from the user and finds out if it's an odd or even number(this is my first time on turing) <>>
What is the problem you are having?
<cant get it to work>
Describe what you have tried to solve this problem
<tried everything i am capable to>
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
<var number: int
put "Write a number to find out if it's Even/Odd numbers:" ..
get number
for i : 2 .. 50 by 2
put "This is a Even number"
else if
put "This is a Odd number"
end for
>
Turing: |
<var number: int
put "Write a number to find out if it's Even/Odd numbers:" ..
get number
for i : 2 .. 50 by 2
put "This is a Even number"
else if
put "This is a Odd number"
end for
>
|
Please specify what version of Turing you are using
<4.1.1> |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
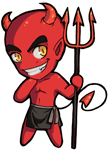
|
Posted: Thu Apr 28, 2011 4:51 pm Post subject: RE:Find if its a odd or even number |
|
|
It's one of those questions that asks you to implement a definition; so you should go and review the formal definition of what it means for a number to be odd or even. This might seem trivial, but it's a good practice.
After that, try out a few numbers on a piece of paper.
Is 42 even or odd? How did you get the answer?
Is 43 even or odd? How did you get the answer?
The "How did you get the answer?" part is what you need to write the code for, but that's simply a programatic encoding of a process that you already should be able to perform and describe in some other way. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Thu Apr 28, 2011 4:51 pm Post subject: RE:Find if its a odd or even number |
|
|
You have an else if (with no condition), but no if. 'for i: 2..50 by 2' will output all even numbers from 2 to 50.
You'll want to look into the mod operator. Also, fix that if statement.
EDIT: You win this time, Tony. |
|
|
|
|
 |
tyuo9980
|
Posted: Thu Apr 28, 2011 5:18 pm Post subject: Re: Find if its a odd or even number |
|
|
When you program you should be thinking about the basics, as if you were teaching someone who has no idea on the topic.
Now how would you make the computer interpret if a number is odd or even? What are the properties of those numbers? After you have that figured out, the answer should be pretty simple.
For example, the properties of a prime number is that it can only be divided by 1 and itself without a remainder, so a basic (and inefficient) way to figure out those numbers would be to see if they could be divided without a remainder.
'mod' is a function to calculate the remainder of two numbers.
var input : int
get input
for x : 2 .. input
if input mod x = 0 then
put "the number is a prime"
end if
end for |
|
|
|
|
 |
sparkz1
|
Posted: Fri Apr 29, 2011 6:03 pm Post subject: RE:Find if its a odd or even number |
|
|
var number: int
put "Write a number to find out if it's Even/Odd numbers:" ..
get number
if number mod 2=0 then
put "even"
else
put "odd"
end if
Thanks guys, really appreciate it.
1 more question: how do i make it so that its only from 2 .. 50? I added "for i : 2 .. 50" but it still will get the answer for higher numbers |
|
|
|
|
 |
Raknarg

|
Posted: Fri Apr 29, 2011 6:27 pm Post subject: RE:Find if its a odd or even number |
|
|
Turing: |
var number: int
put "Write a number to find out if it's Even/Odd numbers:" ..
get number
if number <= 2 and number >= 50 then
if number mod 2=0 then
put "even"
else
put "odd"
end if
end if
|
There's better ways of doing it, but this way the code doesn't run if you put in an invalid number. |
|
|
|
|
 |
Raknarg

|
Posted: Fri Apr 29, 2011 6:29 pm Post subject: Re: Find if its a odd or even number |
|
|
Actually, I should just show you a better way...
Turing: |
var number: int
loop
put "Write a number to find out if it's Even/Odd numbers:" ..
get number
exit when number >= 2 and number <= 50
end loop
if number mod 2=0 then
put "even"
else
put "odd"
end if
|
That way it'll run forever until it gets a valid number  |
|
|
|
|
 |
Tony
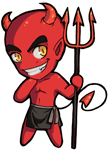
|
Posted: Fri Apr 29, 2011 6:37 pm Post subject: RE:Find if its a odd or even number |
|
|
@Raknarg -- it might be hard to answer trivial questions without supplying the full answers, but the latter would be preferred. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Raknarg

|
Posted: Fri Apr 29, 2011 6:43 pm Post subject: RE:Find if its a odd or even number |
|
|
Sorry Tony, I had a different teaching experience than you probs did. I found that I learned more when my teacher showed me how to do the question properly than if I tried to create the solution myself. Not that I didn't try, but I'd rather learn how to do it better than worse.
But fine. I'll restrain myself. |
|
|
|
|
 |
mirhagk
|
Posted: Fri Apr 29, 2011 6:53 pm Post subject: RE:Find if its a odd or even number |
|
|
it's really a difficult situation in computer sciences, you can show them code, and people learn better sometimes, since they aren't stressing and struggling, but alot of times people just copy and paste. Unless you know the person I'd suggest simply attempting to lead in the right direction as much as you can, but never supply a full solution |
|
|
|
|
 |
Tony
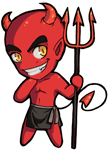
|
Posted: Fri Apr 29, 2011 6:57 pm Post subject: RE:Find if its a odd or even number |
|
|
I think a good compromise would be to provide proper examples to related, but not exact, problems. Linking to documentation with [ tdoc ] tags is always a good idea.
In the context of this particular question, perhaps an example of a loop that validates numbers above 42?
I'll grant you that all of this is subjective, but I feel that it's more important to learn how to approach and solve problems, rather than memorizing how to solve one particular type of a problem (even if that is done really well). Having just read a full solution, it might be too tempting to simply copy it, or maybe just retype it. It takes away that spark you get with "oh, I've just got this myself", which I personally found to be a major driving force for me in this field. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
mirhagk
|
Posted: Fri Apr 29, 2011 7:02 pm Post subject: RE:Find if its a odd or even number |
|
|
It's true, like when you write a particularly nifty function, or find some super neat optimization and you sit back be like "I'm awesome" lol.
But yeah linking to some code that made sure a number was in a range seems appropriate (I'm sure there are at least a 100 of that one line written in various forms for programs on this forum) |
|
|
|
|
 |
|