Creating buttons to store inputted data.
Author |
Message |
bwong
|
Posted: Mon Apr 25, 2011 3:36 pm Post subject: Creating buttons to store inputted data. |
|
|
What is it you are trying to achieve?
Write a program that draw a shape with properties specified by the user. The program should provide a graphical user interface for user to select a shape, and a colour. For bonus mark, your program should let the user select the size as well. Users must select the properties by clicking the corresponding buttons in the right order. If the order is not correct, the program should output error messages and no shapes will be drawn.
What is the problem you are having?
I was able to format everything, and create the buttons. But, I am having an issue with storing their choices of shape and color in order to create a final product.
Describe what you have tried to solve this problem
I have searched online.... to no avail.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
import GUI
View.Set("graphics:360;400, noecho")
GUI.SetBackgroundColour (grey)
%Variable Declaration
var Star, Circle, Square, title, shape1, shape2, shape3, color1, color2, color3, color4, color5, size1, size2, size3 : int
%Procedures
procedure Donothing
end Donothing
procedure SquarePressed
GUI.Disable (shape1 )
GUI.Disable (shape2 )
GUI.Disable (shape3 )
Square := Window.Open ("title:Square, graphics:150;150")
drawbox (25, 25, 125, 125, black)
end SquarePressed
procedure ShowCircle
GUI.Disable (shape1 )
GUI.Disable (shape2 )
GUI.Disable (shape3 )
Circle := Window.Open (
end ShowCircle
procedure ShowStar
GUI.ShowWindow (Star )
end ShowStar
%Main Programme
title := GUI.CreateButton (100, 360, 160, "Shape-Maker", Donothing )
shape1 := GUI.CreateButton (20, 300, 80, "Square", SquarePressed )
shape2 := GUI.CreateButton (140, 300, 80, "Circle", ShowCircle )
shape3 := GUI.CreateButton (260, 300, 80, "Star", ShowStar )
color1 := GUI.CreateButton (40, 240, 40, "Red", Donothing )
%I get lost after this point. How would I be able to combine the choices of shape and colour together to form a final image?
loop
exit when GUI.ProcessEvent
end loop
|
Please specify what version of Turing you are using
Turing 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
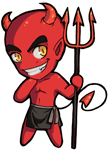
|
Posted: Mon Apr 25, 2011 3:46 pm Post subject: RE:Creating buttons to store inputted data. |
|
|
you could store various information in variables. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
bwong
|
Posted: Mon Apr 25, 2011 3:55 pm Post subject: Re: Creating buttons to store inputted data. |
|
|
Yessir, I understand that. I know the text based version, like:
Turing: |
var name : string
put "What's your name?"
get name
put "Hi, your name is ", name,"!"
|
But how would I do that for my situation right now?
so that, if the user pressed "Square" and "Red", the final product would be a red square? |
|
|
|
|
 |
Tony
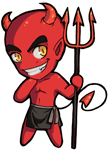
|
Posted: Mon Apr 25, 2011 4:15 pm Post subject: RE:Creating buttons to store inputted data. |
|
|
you can also assign values yourself
code: |
var name : string
name := "bwong"
put "Hello ", name
|
At what point in the code do you know that a user has pressed a particular button? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
bwong
|
Posted: Mon Apr 25, 2011 4:19 pm Post subject: Re: Creating buttons to store inputted data. |
|
|
Tony wrote:
At what point in the code do you know that a user has pressed a particular button?
I don't know. I'm not very versed in Turing, but this will be a significant part of my mark, so I have been working all day on this |
|
|
|
|
 |
Tony
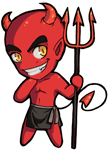
|
Posted: Mon Apr 25, 2011 6:07 pm Post subject: RE:Creating buttons to store inputted data. |
|
|
Read the documentation for GUI.CreateButton; You seem to have the code that does all the right things, but perhaps it's just not yet fully understood. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
bwong
|
Posted: Mon Apr 25, 2011 8:06 pm Post subject: Re: RE:Creating buttons to store inputted data. |
|
|
Tony @ Mon Apr 25, 2011 6:07 pm wrote: Read the documentation for GUI.CreateButton; You seem to have the code that does all the right things, but perhaps it's just not yet fully understood.
Alright, I read the entire document twice, throughly, but, it doesn't exactly help me.
I believe it would be correct to reword my plead for assistance:
I would like to create a program which creates user-defined shapes. The user will be able to chose (1) a shape, (2) a colour, and (3) the size.
My approach to this issue is by creating a menu-like GUI. I have used some buttons, as you would see if you were to copy and paste the code (below) into turing.
I was able to get all the buttons to appear, but was unable to use the buttons to create a final image. I would like it to be kind of like this (below), except in a visual form, where the user can click on a box, or button instead of typing in a letter:
Turing: |
put "Please choose a shape: "
put "A. Box"
put "B. Circle"
put "C. Star"
get shape
|
Here is an updated code... Nothing special, just with all the wanted buttons there
Turing: |
import GUI
View.Set("graphics:360;400, noecho")
GUI.SetBackgroundColour (grey)
%Variable Declaration
var Star, Circle, Square, title, shape1, shape2, shape3, color1, color2, color3, color4, size1, size2, size3 : int
%Procedures
procedure Donothing
end Donothing
procedure SquarePressed
GUI.Disable (shape1 )
GUI.Disable (shape2 )
GUI.Disable (shape3 )
Square := Window.Open ("title:Square, graphics:150;150")
drawbox (25, 25, 125, 125, black)
end SquarePressed
procedure ShowCircle
GUI.Disable (shape1 )
GUI.Disable (shape2 )
GUI.Disable (shape3 )
Circle := Window.Open ("title:Circle, graphics:150;150")
drawoval (75, 75, 35, 35, black)
end ShowCircle
procedure ShowStar
GUI.ShowWindow (Star )
end ShowStar
%Main Programme
title := GUI.CreateButton (0100, 360, 160, "Shape-Maker", Donothing )
shape1 := GUI.CreateButton (020, 300, 080, "Square", SquarePressed )
shape2 := GUI.CreateButton (140, 300, 080, "Circle", ShowCircle )
shape3 := GUI.CreateButton (260, 300, 080, "Star", ShowStar )
color1 := GUI.CreateButton (020, 240, 060, "Red", Donothing )
color2 := GUI.CreateButton (100, 240, 060, "Orange", Donothing )
color3 := GUI.CreateButton (200, 240, 060, "Green", Donothing )
color4 := GUI.CreateButton (280, 240, 060, "Blue", Donothing )
size1 := GUI.CreateButton (020, 180, 080, "Small", Donothing )
size2 := GUI.CreateButton (140, 180, 080, "Medium", Donothing )
size3 := GUI.CreateButton (260, 180, 080, "Large", Donothing )
loop
exit when GUI.ProcessEvent
end loop
|
|
|
|
|
|
 |
Tony
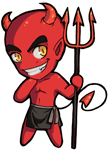
|
Posted: Mon Apr 25, 2011 9:09 pm Post subject: RE:Creating buttons to store inputted data. |
|
|
Alright, lets walk through this. The documentation starts out with
Quote:
The button widget is used to implement a textual button. When you click on a button, the button's action procedure is called.
You sort of understand that well enough to link to SquarePressed in
code: |
shape1 :=GUI.CreateButton (020, 300, 080, "Square", SquarePressed)
|
and to actually implement that procedure.
So:
- button linked to a procedure
- button pressed -> procedure runs
- that procedure has no other entry points
- when the procedure runs, we could assume that the corresponding button has been pressed
Now you just need to "remember" (meaning record information somewhere, typically variables), that you were in that state. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
bwong
|
Posted: Tue Apr 26, 2011 12:11 pm Post subject: Re: RE:Creating buttons to store inputted data. |
|
|
Tony @ Mon Apr 25, 2011 9:09 pm wrote: Alright, lets walk through this. The documentation starts out with
So:
- button linked to a procedure
- button pressed -> procedure runs
- that procedure has no other entry points
- when the procedure runs, we could assume that the corresponding button has been pressed
Now you just need to "remember" (meaning record information somewhere, typically variables), that you were in that state.
But how would I do that last part?
EDIT: I felt the need to use a less advanced code, since I am not so versed in Turing. Hence I have this now:
Turing: |
setscreen ("graphics:600;425")
%Variable Declaration
var shapechoice : string
var colorchoice, sizechoice : int
var x, y : int
var font : int
var click : int
var shape : int
font := Font.New ("comic-sans:12")
%Main Programme
loop
shapechoice := ""
colorchoice := 0
sizechoice := 0
%Formating
loop
Draw.Text ("What shape do you want? Your Shape Is:", 000, 400, font, black)
Draw.Box (005, 320, 065, 380, black)
Draw.Star (080, 320, 150, 380, black)
Draw.MapleLeaf (160, 320, 225, 380, black)
Draw.Text ("What colour do you want? Your Colour Is:", 000, 305, font, black)
Draw.FillBox (005, 280, 065, 300, 012)
Draw.FillBox (080, 280, 145, 300, 079)
Draw.FillBox (160, 280, 225, 300, 009)
Draw.Text ("What size would you like? Your Size Is:", 000, 260, font, black)
Draw.Oval (030, 220, 015, 015, 016)
Draw.Oval (105, 220, 025, 025, 016)
Draw.Oval (195, 220, 035, 035, 016)
%The user will now choose their settings
mousewhere (x, y, click )
if click = 1 then
if x >= 005 and x <= 065 and y >= 320 and y <= 380
then
Draw.Box (375, 330, 415, 370, black)
shapechoice := "Box"
elsif x >= 080 and x <= 150 and y >= 320 and y <= 380
then
Draw.Star (375, 330, 415, 370, black)
shapechoice := "Star"
elsif x >= 160 and x <= 225 and y >= 320 and y <= 380
then
Draw.MapleLeaf (375, 330, 415, 370, black)
shapechoice := "Maple Leaf"
end if
if x >= 005 and x <= 065 and y >= 280 and y <= 300
then
Draw.FillBox (375, 280, 415, 300, 012)
colorchoice := 012
elsif x >= 080 and x <= 145 and y >= 280 and y <= 300
then
Draw.FillBox (375, 280, 415, 300, 075)
colorchoice := 075
elsif x >= 160 and x <= 225 and y >= 280 and y <= 300
then
Draw.FillBox (375, 280, 415, 300, 009)
colorchoice := 009
end if
if x >= 015 and x <= 045 and y >= 205 and y <= 235
then
Draw.Text ("Small", 375, 230, font, black)
sizechoice := 015
elsif x >= 80 and x <= 130 and y >= 195 and y <= 245
then
Draw.Text ("Medium", 375, 230, font, black)
sizechoice := 025
elsif x >= 160 and x <= 230 and y >= 185 and y <= 255
then
Draw.Text ("Large", 375, 230, font, black)
sizechoice := 035
end if
end if
exit when shapechoice not= "" and colorchoice not= 0 and sizechoice not= 0
end loop
%Programme gets stuck at this loop...
if shapechoice not= "" and colorchoice not= 0 and sizechoice not= 0 then
if shapechoice = "Box"
then
Draw.FillBox (100 + sizechoice, 100 + sizechoice, 100 + sizechoice, 100 + sizechoice, colorchoice )
elsif shapechoice = "Star"
then
Draw.FillStar (100 + sizechoice, 100 + sizechoice, 100 + sizechoice, 100 + sizechoice, colorchoice )
elsif shapechoice = "Maple Leaf"
then
Draw.FillMapleLeaf (100 + sizechoice, 100 + sizechoice, 100 + sizechoice, 100 + sizechoice, colorchoice )
end if
end if
end loop
|
|
|
|
|
|
 |
Tony
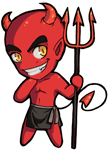
|
Posted: Wed Apr 27, 2011 2:33 am Post subject: RE:Creating buttons to store inputted data. |
|
|
Here's a trivial example that should give you an idea
code: |
var was_visited : boolean := false
procedure some_procedure
was_visited := true
end some_procedure
put "has procedure been visited? ", was_visited
some_procedure
put "has procedure been visited? ", was_visited
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|