Need help making a Truth Table using Turing
Author |
Message |
Bucket
|
Posted: Fri Mar 18, 2011 9:57 pm Post subject: Need help making a Truth Table using Turing |
|
|
What is it you are trying to achieve?
I'm trying to create a truth table that uses 5 variables and the AND, OR, NOT, XOR expressions.
What is the problem you are having?
Confused about what to write.
Describe what you have tried to solve this problem
I tried using the if, then, and or statements but I can't seem to get it right.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
setscreen ("text")
var a : boolean
var b : boolean
var c : boolean
var d : boolean
var e : boolean
put " A" : 10, " B" : 10, " C" : 10, " D" : 10, " E" : 10, " Output" : 10
for countera : 0 .. 1
for coutnerb : 0 .. 1
for counterc : 0 .. 1
for counterd : 0 .. 1
for countere : 0 .. 1
This is all I have which isn 't really anything. x.x
|
Please specify what version of Turing you are using
Currently using version 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Zren

|
Posted: Fri Mar 18, 2011 11:14 pm Post subject: RE:Need help making a Truth Table using Turing |
|
|
How would you do just one case here?
A and B or C not D xor E = ?
We here have 5 variables? or is it just that? We have 5 boolean variables, but we also have 4 operators that are variable as well. All in all, 9 variables.
Lets just focus on a situation of 2 booleans, and one operator.
You can't exactly pass operators as a variable though, as there is no operator data type that could be used to store in a variable.
Instead, we'll use constants to represent them.
const op_or := 0
const op_and := 1
You could use an enum (enumerated type) to simplify this, however it becomes more complex and annoying in turing with enums and loops.
Then you could use a case selection (or a bunch of ifs) to actually get the result of what that operator did to the booleans. If I were me, I'd put this in it's own function, as this code will be used alot.
Turing: |
case (op1):
label op_or:
put x or y
end case
|
After that, you'll be stacking up those for loops for each value of a, b, and the operator.
Then you'll have a two variable truth table!
For more than two variables, you have to assume that you first need to know the result of the first two, then compare that result to the third, then compare that ... and so on.
fn(fn(a, op1, b), op2, c) |
|
|
|
|
 |
Tony
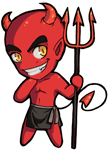
|
Posted: Fri Mar 18, 2011 11:18 pm Post subject: RE:Need help making a Truth Table using Turing |
|
|
Quote: AND, OR, NOT, XOR
All of those operators work on two arguments, not five. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Prince Pwn
|
Posted: Sun Mar 20, 2011 1:06 am Post subject: RE:Need help making a Truth Table using Turing |
|
|
First off it looks like he is looking for help with his homework. It doesn't seem like he knows about cases or labels yet so let's not jump too far ahead
Start off by ending your end fors. Then inside your nested for loop, use if statments comparing the for loop variables using the expressions and, or, not, xor.
An if statement looks as follows:
code: |
if 5 < x then
put "1 is less than your variable"
end if
|
Place that block into a for loop that counts from 1 to 10 and look at the results. Then you should understand how a for loop works.
Now apply the for loop and if statements with what you know about truth tables and it should become clear to you on how to go about solving the problem at hand. |
|
|
|
|
 |
|
|