Author |
Message |
ProgrammingFun

|
Posted: Sat Jan 08, 2011 1:09 pm Post subject: Loop Fail |
|
|
I can't think of any way to do this in a more efficient way (perhaps using for-loops)...so I posted to ask, is there a better way to do this?
Java: |
checkBoard[0][0] = gameBoard[x-1][y-1];
checkBoard[0][1] = gameBoard[x][y-1];
checkBoard[0][2] = gameBoard[x+1][y-1];
checkBoard[1][0] = gameBoard[x-1][y];
checkBoard[1][1] = gameBoard[x][y];
checkBoard[1][2] = gameBoard[x+1][y];
checkBoard[2][0] = gameBoard[x-1][y+1];
checkBoard[2][1] = gameBoard[x][y+1];
checkBoard[2][2] = gameBoard[x+1][y+1];
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
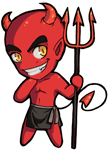
|
Posted: Sat Jan 08, 2011 1:15 pm Post subject: RE:Loop Fail |
|
|
If by "more efficient" you mean "less lines of code", then yes. Two for-loops and a single assignment statement.
Edit: strictly speaking, you can do this with a single for-loop, at the cost of readability. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Sat Jan 08, 2011 1:17 pm Post subject: RE:Loop Fail |
|
|
A nested for loop should do the trick.
code: |
for x_ : 0-2 {
for y : 0-2 {
checkerBoard[x_][y] = gameBoard[x+y-1][x+x_-1]
}
}
|
|
|
|
|
|
 |
ProgrammingFun

|
Posted: Sat Jan 08, 2011 2:23 pm Post subject: RE:Loop Fail |
|
|
Thanks for the help. I used the following method:
Java: |
for (int a = 0; a < 3; a++ )
{
for (int b = 0; b < 3; b++ )
{
checkBoard [a ][b ] = gameBoard [y + b - 1][x + a - 1];
System. out. print (checkBoard [a ][b ] + " ");
}
}
|
|
|
|
|
|
 |
ProgrammingFun

|
Posted: Sat Jan 08, 2011 5:02 pm Post subject: RE:Loop Fail |
|
|
An unrelated question:
If nowin is a boolean will:
if (nowin) mean the same thing as if (nowin == true)
if (!nowin) mean the same thing as if (nowin == false)
Thanks for the help! |
|
|
|
|
 |
2goto1

|
Posted: Sat Jan 08, 2011 5:24 pm Post subject: RE:Loop Fail |
|
|
Yes and yes. Try spitting out each to your console to confirm |
|
|
|
|
 |
ProgrammingFun

|
Posted: Sat Jan 08, 2011 5:52 pm Post subject: Re: RE:Loop Fail |
|
|
2goto1 @ Sat Jan 08, 2011 5:24 pm wrote: Yes and yes. Try spitting out each to your console to confirm OK, thanks for the help... |
|
|
|
|
 |
copthesaint

|
Posted: Mon Jan 10, 2011 3:31 am Post subject: Re: Loop Fail |
|
|
Tony wrote:
If by "more efficient" you mean "less lines of code", then yes. Two for-loops and a single assignment statement.
Edit: strictly speaking, you can do this with a single for-loop, at the cost of readability.
Sooo...
Does this work for yea then SAVES 1 LINE!!! *not counting brackets*
Java: | for (int i = 0; i < 9; i++ ){
heckBoard [((i- (i% 3))/ 3)][(i% 3)] = gameBoard [y + (i% 3) - 1][x + ((i- (i% 3))/ 3) - 1];
System. out. print (checkBoard [a ][(i% 3)] + " ");
} |
Lol I dont want to know how complex it would be to minipulate the values for a 3 dimensional array doing this... lol |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Mon Jan 10, 2011 10:45 am Post subject: RE:Loop Fail |
|
|
Incidentally, always choose readability over number of lines of code. In fact, choose readability over anything except a real requirement. |
|
|
|
|
 |
Tony
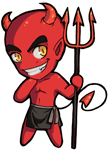
|
|
|
|
 |
copthesaint

|
Posted: Tue Jan 11, 2011 10:28 pm Post subject: RE:Loop Fail |
|
|
Lol, that is really great tony... , Also DemonWasp, whats the fun in readability jk |
|
|
|
|
 |
|