String Manipulation Problem
Author |
Message |
highly
|
Posted: Sat Nov 28, 2009 4:16 am Post subject: String Manipulation Problem |
|
|
I'm trying to achieve an effect where the output is
Highly
HighlyHighly
HighlyHighlyHighly
HighlyHighlyHighlyHighly
HighlyHighlyHighlyHighlyHighly
HighlyHighlyHighlyHighly
HighlyHighlyHighly
HighlyHighly
Highly
HighlyHighly
HighlyHighlyHighly
HighlyHighlyHighlyHighly
etc, and will repeat this pattern for as long as I determine
My problem is that when I try to use -= when pertaining to a string, I get an error. there must be a way to remove text from the end of a string, but I do not know it, and it wasn't covered in the string manipulation tutorial.
I'm not new to programming, just new to turing.
I'm, using turing 4.1.1a
here's my code,
Turing: |
var i : int
var n : string
var p : string
var mx : int
var pc : int
i := 0
n := "Highly"
p := "Highly"
mx := 100
pc := 0
loop
i += 1
put n
if pc <= 0 then
loop
pc += 1
n += p
exit when pc := 5
end loop
end if
if pc >= 5 then
loop
pc -= 1
n := -p
exit when pc := 0
end loop
end if
exit when i = mx
end loop
put "HIGHLY"
|
the problem occurs around "n := -p ", and "n -= p" doens't work either. Both n and p are strings.
any help is much appreciated |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Zren

|
Posted: Sat Nov 28, 2009 5:45 am Post subject: Re: String Manipulation Problem |
|
|
To clip the string you have to create a substring with the range of the beginning to X characters from the end.
Turing: |
var str := "holy mother of jeeeeus"
put length (str )
put str (4) %Chracter at 4
put str (1 .. 3) %Substring of range 1-3
put str (1 .. length (str )) %Substring of range 1-End of string
put str (1 .. length (str ) - 5) %Substring with the last 5 characters clipped off
|
What other languages do you know btw? |
|
|
|
|
 |
highly
|
Posted: Sat Nov 28, 2009 6:20 am Post subject: Re: String Manipulation Problem |
|
|
Zren @ Sat Nov 28, 2009 5:45 am wrote: To clip the string you have to create a substring with the range of the beginning to X characters from the end.
Turing: |
var str := "holy mother of jeeeeus"
put length (str )
put str (4) %Chracter at 4
put str (1 .. 3) %Substring of range 1-3
put str (1 .. length (str )) %Substring of range 1-End of string
put str (1 .. length (str ) - 5) %Substring with the last 5 characters clipped off
|
What other languages do you know btw?
Well, I'm most well-versed in AS2, and have dabbled in java, ahk, etc. Nothing besides as2 has really done anything for me lol
besides that I've meddled in various application scripting languages (mIRC scripting language, lua briefly a loooong time ago for cortex command, etc) but have only recently gotten into it at any depth.
But I love turing. I'd remembered reading about it in a book about the history of Artificial Intelligence, and recently ran into a game using it. Very fun language, if I do say so myself.
EDIT: Finished the code, was rather simple, Thanks to you Zren
Turing: |
var i : int
var n : string
var p : string
var mx : int
var pc : int
i := 0
n := "penis"
p := "penis"
mx := 100
pc := 0
loop
i + = 1
if pc <= 0 then
loop
pc + = 1
n + = p
put n
exit when pc = 5
end loop
end if
if pc >= 5 then
loop
pc - = 1
n := n (1 .. length (n ) - 5)
put n
exit when pc = 0
end loop
end if
exit when i = mx
end loop
put "PENIS"
|
|
|
|
|
|
 |
Zren

|
Posted: Sat Nov 28, 2009 9:07 am Post subject: Re: String Manipulation Problem |
|
|
You'll learn to hate and love Turing at the same time. It's simplity rubs off at the really annoying limitations sometimes. But you just can't get rid of it.
Btw, since you finished it that way, I might as well point out a reall usefull function in Turing syntax.
put "Dude"..
put "What"
Will output DudeWhat on the same line. So you don't need to modify a string. You could write it through a for loop. To be more specific, the "____".. at the end of a put statement will allow the next put to be placed on the same line.
Turing: | var str := "Zing"
var c := 1
var inc := 1
loop
for i : 1 .. c
put str, "" ..
end for
put ""
c += inc
if c <= 1 or c >= 5 then
inc *= -1
end if
end loop
|
|
|
|
|
|
 |
Tony
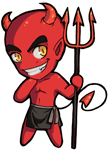
|
Posted: Sat Nov 28, 2009 2:38 pm Post subject: Re: String Manipulation Problem |
|
|
and then you realize that Turing has a build in function repeat
code: |
var str := "Zing"
var c := 1
var inc := 1
loop
put repeat(str, c)
c += inc
if c <= 1 or c >= 5 then
inc *= -1
end if
end loop
|
This also reminds me of this magic |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
highly
|
Posted: Sat Nov 28, 2009 2:45 pm Post subject: Re: String Manipulation Problem |
|
|
Tony @ Sat Nov 28, 2009 2:38 pm wrote: and then you realize that Turing has a build in function repeat
code: |
var str := "Zing"
var c := 1
var inc := 1
loop
put repeat(str, c)
c += inc
if c <= 1 or c >= 5 then
inc *= -1
end if
end loop
|
This also reminds me of this magic
Lmao! Something tells me you're somewhat of a turing guru? |
|
|
|
|
 |
|
|