Collision Detection Help
Author |
Message |
ajones88
|
Posted: Mon Nov 23, 2009 6:41 pm Post subject: Collision Detection Help |
|
|
What is it you are trying to achieve?
I am creatign a tag game involving 2 ovals, when one oval touches another, the other person should become it.
What is the problem you are having?
Turing is not detecting a collision
Describe what you have tried to solve this problem
Tried whatdotcolor, didnt work because the red ovalw as always on top so it couldnt detect when the blue one collided, so i am trying with a simple collision detection.
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
Turing: |
setscreen ("graphics")
var x := 150
var y := 100
var x1 := 450
var y1 := 300
var keys, key : array char of boolean
var Red := 1
var Blue := 0
var r := 10
var distance := ((x1 - x ) * (x1 - x )) + ((y1 - y ) * (y1 - y )) ** 0. 5
colorback (black)
color (brightred)
cls
loop
if Red = 1 then
put "Red is It"
else
color (brightblue)
put "Blue is It"
color (brightred)
end if
Input.KeyDown (keys )
drawfilloval (x, y, 10, 10, brightred)
if keys (KEY_UP_ARROW) and y + 10 < maxy then
y + = 1
end if
if keys (KEY_DOWN_ARROW) and y - 10 > 0 then
y - = 1
end if
if keys (KEY_LEFT_ARROW) and x - 10 > 0 then
x - = 1
end if
if keys (KEY_RIGHT_ARROW) and x + 10 < maxx then
x + = 1
end if
drawfilloval (x, y, r, r, brightred)
Input.KeyDown (key )
drawfilloval (x1, y1, r, r, brightblue)
if key ('w') and y1 + 10 < maxy then
y1 + = 1
end if
if key ('s') and y1 - 10 > 0 then
y1 - = 1
end if
if key ('a') and x1 + 10 > 0 then
x1 - = 1
end if
if key ('d') and x1 - 10 < maxx then
x1 + = 1
end if
drawfilloval (x1, y1, 10, 10, brightblue)
View.Update
delay (5)
cls
if distance < r + r and Red = 1 then
Red := 0
elsif distance < r + r and Red = 0 then
Red := 1
end if
end loop
|
Please specify what version of Turing you are using
Turing V. 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
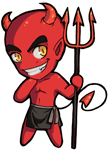
|
Posted: Mon Nov 23, 2009 7:19 pm Post subject: RE:Collision Detection Help |
|
|
You've got the right idea, but try running this code
code: |
put "distance is: ", distance
if distance < r + r and Red = 1 then
Red := 0
elsif distance < r + r and Red = 0 then
Red := 1
end if
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ajones88
|
Posted: Mon Nov 23, 2009 7:49 pm Post subject: Re: Collision Detection Help |
|
|
Got it Had to put the equation for distance after the movement.
Turing: |
setscreen ("graphics")
var x := 150
var y := 100
var x1 := 400
var y1 := 300
var keys, key : array char of boolean
var Red := 1
var Blue := 0
var r := 10
colorback (black)
color (brightred)
cls
loop
if Red = 1 then
put "Red is It"
else
color (brightblue)
put "Blue is It"
color (brightred)
end if
Input.KeyDown (keys )
drawfilloval (x, y, 10, 10, brightred)
if keys (KEY_UP_ARROW) and y + 10 < maxy then
y + = 1
end if
if keys (KEY_DOWN_ARROW) and y - 10 > 0 then
y - = 1
end if
if keys (KEY_LEFT_ARROW) and x - 10 > 0 then
x - = 1
end if
if keys (KEY_RIGHT_ARROW) and x + 10 < maxx then
x + = 1
end if
drawfilloval (x, y, r, r, brightred)
Input.KeyDown (key )
drawfilloval (x1, y1, r, r, brightblue)
if key ('w') and y1 + 10 < maxy then
y1 + = 1
end if
if key ('s') and y1 - 10 > 0 then
y1 - = 1
end if
if key ('a') and x1 + 10 > 0 then
x1 - = 1
end if
if key ('d') and x1 - 10 < maxx then
x1 + = 1
end if
drawfilloval (x1, y1, 10, 10, brightblue)
View.Update
delay (5)
cls
var distance := ((x1 - x ) * (x1 - x )) + ((y1 - y ) * (y1 - y )) ** 0. 5
put "distance is: ",distance
if distance < r + r and Red = 1 then
Red := 0
elsif distance < r + r and Red = 0 then
Red := 1
end if
end loop
|
|
|
|
|
|
 |
ajones88
|
Posted: Mon Nov 23, 2009 8:00 pm Post subject: Re: Collision Detection Help |
|
|
Also any help with a better detection for the diagonal radius would be apreciated. |
|
|
|
|
 |
Tony
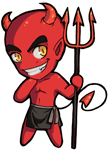
|
Posted: Mon Nov 23, 2009 8:09 pm Post subject: RE:Collision Detection Help |
|
|
code: |
loop
var distance := ...
end loop
|
You are creating a new variable in every iteration of the loop.
Also, it your ovals are circles, then the sum of their radii will always be the same, regardless of the angle. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ajones88
|
Posted: Mon Nov 23, 2009 8:22 pm Post subject: Re: RE:Collision Detection Help |
|
|
Tony @ Mon Nov 23, 2009 8:09 pm wrote: code: |
loop
var distance := ...
end loop
|
You are creating a new variable in every iteration of the loop.
Also, it your ovals are circles, then the sum of their radii will always be the same, regardless of the angle.
but if 2 "corner" edges are touching, the origin of the circles are farther apart |
|
|
|
|
 |
Tony
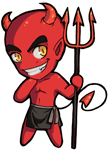
|
Posted: Mon Nov 23, 2009 8:31 pm Post subject: RE:Collision Detection Help |
|
|
If I'm guessing at what you are talking about correctly, then you are talking about the case when distance equals to the sum of radii. In such a case, you should adjust your if conditions accordingly. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ajones88
|
Posted: Mon Nov 23, 2009 9:49 pm Post subject: Re: RE:Collision Detection Help |
|
|
Tony @ Mon Nov 23, 2009 8:31 pm wrote: If I'm guessing at what you are talking about correctly, then you are talking about the case when distance equals to the sum of radii. In such a case, you should adjust your if conditions accordingly.
how would i go about adjusting that. Ive played with it for a bit, but i cant seem to get it. Also, is there some sort of delay i can put in so it wont keep switching who is it while they are touching, as sometimes the same person emerges it. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
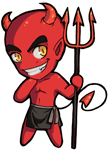
|
Posted: Mon Nov 23, 2009 10:01 pm Post subject: RE:Collision Detection Help |
|
|
how would you check if one number is less than or equals to another number?
As for delays -- yes. You might want to wait for some time, see -- timemodule. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ajones88
|
Posted: Mon Nov 23, 2009 10:12 pm Post subject: RE:Collision Detection Help |
|
|
I want to be able to move, but dont want the value of red to change for ex. 1 second since it last changed. Correct me if i am wrong, but as far as i can tell, none of those do that.
Thanks |
|
|
|
|
 |
Tony
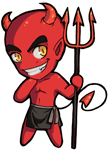
|
Posted: Tue Nov 24, 2009 12:26 am Post subject: RE:Collision Detection Help |
|
|
The only methods that are blocking are delay and DelaySinceLast. The rest return a value to you right away. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ajones88
|
Posted: Tue Nov 24, 2009 5:43 pm Post subject: Re: Collision Detection Help |
|
|
Got it had to add a check that is reset when the distance is greater than the 2 radii
Turing: |
setscreen ("graphics")
var x := 150
var y := 100
var x1 := 400
var y1 := 300
var keys, key : array char of boolean
var Red := 1
var Blue := 0
var r := 10
var check := 0
colorback (black)
color (brightred)
cls
loop
if Red = 1 then
put "Red is It"
else
color (brightblue)
put "Blue is It"
color (brightred)
end if
Input.KeyDown (keys )
drawfilloval (x, y, 10, 10, brightred)
if keys (KEY_UP_ARROW) and y + 10 < maxy then
y + = 1
end if
if keys (KEY_DOWN_ARROW) and y - 10 > 0 then
y - = 1
end if
if keys (KEY_LEFT_ARROW) and x - 10 > 0 then
x - = 1
end if
if keys (KEY_RIGHT_ARROW) and x + 10 < maxx then
x + = 1
end if
if Red = 1 then
drawfilloval (x, y, 11, 11, yellow)
end if
drawfilloval (x, y, r, r, brightred)
Input.KeyDown (key )
drawfilloval (x1, y1, r, r, brightblue)
if key ('w') and y1 + 10 < maxy then
y1 + = 1
end if
if key ('s') and y1 - 10 > 0 then
y1 - = 1
end if
if key ('a') and x1 + 10 > 0 then
x1 - = 1
end if
if key ('d') and x1 - 10 < maxx then
x1 + = 1
end if
if Red = 0 then
drawfilloval (x1, y1, 12, 12, yellow)
end if
drawfilloval (x1, y1, 10, 10, brightblue)
View.Update
delay (5)
cls
var distance := ((x1 - x ) * (x1 - x )) + ((y1 - y ) * (y1 - y )) ** 0. 5
if distance < 11 + 11 and Red = 1 and check = 0 then
Red := 0
check := 1
elsif distance < 11 + 11 and Red = 0 and check = 0 then
Red := 1
check := 1
end if
if distance > 11+ 11 then
check:= 0
end if
end loop
|
|
|
|
|
|
 |
|
|