Advanced string manipulation: Help needed
Author |
Message |
echeese
|
Posted: Sat Nov 22, 2003 6:49 pm Post subject: Advanced string manipulation: Help needed |
|
|
Hello, all, I need commands for string manipulation. Please post all the ones you can think of. If there's one like PHP's explode, post it as well, or something that can get the x'th word from a string like
getword("This is a test, ok?", 3, " ") would return "test," |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
thoughtful

|
Posted: Sat Nov 22, 2003 6:58 pm Post subject: string manupulation |
|
|
you can use something like this
code: |
var temp : string := "this is a test"
put temp (1 .. 4)
put temp (11 .. *)
|
|
|
|
|
|
 |
echeese
|
Posted: Sat Nov 22, 2003 7:05 pm Post subject: (No subject) |
|
|
Yes, but what if you don't know the length of the word, or even how many words there are? |
|
|
|
|
 |
Tony
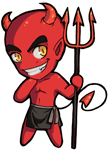
|
Posted: Sat Nov 22, 2003 9:03 pm Post subject: (No subject) |
|
|
to get the length of the string, you can use
code: |
put length("compsci")
|
use substrings to find spaces and break sentance into separate words.
you can ether use variableName(index) method or index() |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Andy
|
Posted: Sun Nov 23, 2003 3:37 pm Post subject: (No subject) |
|
|
to check the number of words:
code: |
var count:=0
for i:1..length(word)
if word(i)=" " then
count+=1
end if
end for
if word(*) not= " " then
count+=1
end if
put count
|
|
|
|
|
|
 |
Homer_simpson

|
Posted: Sun Nov 23, 2003 6:36 pm Post subject: (No subject) |
|
|
how bout a nice function for it?
code: | function returnword (s : string, separator : char, n : int) : string
var wi := 1
var s2 := ""
for i : 1 .. length (s)
if wi >= n then
if s (i) = separator then
result s2
end if
s2 += s (i)
end if
if s (i) = separator then
wi += 1
end if
end for
if s2 not= "" then
result s2
else
result "Couldn't find that many words!"
end if
end returnword
put returnword ("Spammers suck juicy cocks!", " ", 3)
|
|
|
|
|
|
 |
echeese
|
Posted: Tue Nov 25, 2003 8:28 am Post subject: (No subject) |
|
|
Thank you Homer... By the way, this is for my next Net project... It's a 3 letter word with the first letter I, last is C and it's full of R... Take a guess...
Argh!!! I'm glad I didn't submit this post... Is there a way using Net to read more than 255 chars? Server sends me a string > 255 chars |
|
|
|
|
 |
Tony
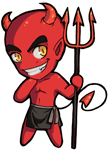
|
Posted: Tue Nov 25, 2003 11:16 am Post subject: (No subject) |
|
|
i belive homer wrote a net server app that accepts 255+ characters. You should take a look at that - its here |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
echeese
|
Posted: Wed Nov 26, 2003 11:13 am Post subject: (No subject) |
|
|
lol, that's my server, with Homer's addons I'm now working on a bot for IRC called cOOTie |
|
|
|
|
 |
Dan
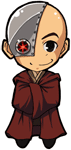
|
Posted: Wed Nov 26, 2003 12:35 pm Post subject: (No subject) |
|
|
echeese wrote: lol, that's my server, with Homer's addons  I'm now working on a bot for IRC called cOOTie
in turing?  |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
echeese
|
Posted: Mon Dec 01, 2003 11:15 am Post subject: (No subject) |
|
|
Hacker Dan: Yes. In Turing... I decided to actually make it a client. Check it out under submissions. |
|
|
|
|
 |
Dan
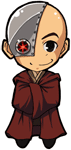
|
Posted: Tue Dec 02, 2003 5:35 pm Post subject: (No subject) |
|
|
wow, you shure got crazy ideas for turings net comands. why not check out c++ ? you get alot more power and fuctionaliy out of it and it has some nice netwrok related librablerys |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
DanShadow

|
Posted: Sun Dec 07, 2003 7:49 pm Post subject: .. |
|
|
Advanced String Manipulation...hmm, well if you want to do it the advanced way, use the almighty 'ord' command, and ascii codes.
This code works out if you want to be very detail specific to characters put in, cast sensitiveness, checking all letters in a word, checking words, etc.
[ I dont know why, but it cuts out the last two letters, ]
code: |
var words : array 1 .. 20 of string
var num_words, input_length : int := 0
var ch_temp : string (1) := ""
var str_temp, input : string := ""
var num_temp, num_temp2 : int := 1
for i : 1 .. 20
words (i) := " "
end for
color (12)
colorback (black)
put "Enter a few words to test this: " ..
color (white)
get input : *
loop
loop
ch_temp := input (num_temp)
num_temp := num_temp + 1
exit when ch_temp = " " or num_temp = length (input)
str_temp := str_temp + ch_temp
put str_temp, " " ..
end loop
words (num_temp2) := str_temp
str_temp := ""
num_temp2 := num_temp2 + 1
exit when num_temp = length (input)
end loop
loop
color (green)
colorback (black)
locate (1, 1)
put "What Word Would You Like to See in the 'Words' array? "
locate (2, 1)
put ""
locate (2, 1)
put "Word: " ..
get num_temp
if num_temp > 0 and num_temp <= 20 then
locate (4, 1)
put ""
locate (4, 1)
put "Word #" ..
color (white)
put num_temp, "" ..
color (green)
put " is: " ..
color (12)
put words (num_temp)
else
locate (4, 1)
put ""
locate (4, 1)
put num_temp, " isnt between 1-20, and therefore isnt in the 'Words' array."
end if
end loop
|
|
|
|
|
|
 |
|
|