I need some help with a project.
Author |
Message |
Nemo296
|
Posted: Sat Apr 04, 2009 11:23 pm Post subject: I need some help with a project. |
|
|
Well, I'm just wondering if its possible to add a OR statement into a if statement
Here's an example.
if GAMES = "Points" or "points" then
Please Help me =D |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dusk Eagle

|
Posted: Sat Apr 04, 2009 11:33 pm Post subject: Re: I need some help with a project. |
|
|
Your statements must be written like this:
code: | if %boolean% or %boolean% |
What that means is you must do something like the following:
code: | if points = 5 or points = 7 |
However, it looks like what you want to do is check for capitalization. This can be accomplished much better through the use of Str.Upper() or Str.Lower().
Turing: |
var input : string
get input
if Str.Lower(input ) = "hello world" then
%code
end if
|
|
|
|
|
|
 |
Tony
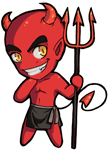
|
Posted: Sat Apr 04, 2009 11:47 pm Post subject: RE:I need some help with a project. |
|
|
there's an example in documentation of using an or -- if. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nemo296
|
Posted: Sun Apr 05, 2009 1:15 am Post subject: Re: I need some help with a project. |
|
|
Dusk Eagle @ Sat Apr 04, 2009 11:33 pm wrote: Your statements must be written like this:
code: | if %boolean% or %boolean% |
What that means is you must do something like the following:
code: | if points = 5 or points = 7 |
However, it looks like what you want to do is check for capitalization. This can be accomplished much better through the use of Str.Upper() or Str.Lower().
Turing: |
var input : string
get input
if Str.Lower(input ) = "hello world" then
%code
end if
|
Thanks that helped me, I got it working. |
|
|
|
|
 |
Nemo296
|
Posted: Sun Apr 05, 2009 2:45 am Post subject: RE:I need some help with a project. |
|
|
Ok, So i fixed that issue. Now I have a new issue.
My Elsif statements don't go past the second one
if BLA = "grrr" then
%CODE
elsif BLA = "Grrr2" then
%CODE2
elsif BLA = "Grrr3" then
%CODE THAT DOESNT WORK
elsif BLA = "Grrr4" then
%CODE THAT DOESNT WORK
end if
Things in bold are what needs fixing |
|
|
|
|
 |
Tony
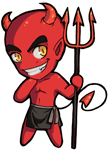
|
Posted: Sun Apr 05, 2009 3:03 am Post subject: RE:I need some help with a project. |
|
|
what is the value of variable BLA when the bolded code doesn't work? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nemo296
|
Posted: Sun Apr 05, 2009 7:43 am Post subject: RE:I need some help with a project. |
|
|
This is my code.
Turing: |
if Str.Lower (VOID ) = "items" then
put "Please enter the item you wish to have."
put "Void Armour, Dragon weapon set, BGS or Ice arrows."
get VOID1
if Str.Lower (VOID1 ) = "void armour" then
AMP := 150 - POINTS
A := 150
AMOUNT := POINTS / 150
GAMES := AMP / 2
elsif
Str.Lower (VOID1 ) = "bgs" then
AMP := 100 - POINTS
A := 100
AMOUNT := POINTS / 100
GAMES := AMP / 2
elsif
Str.Lower (VOID1 ) = "ice arrows" or Str.Lower (VOID1 ) = "ice arrow" then
AMP := 1 - POINTS
A := 1
AMOUNT := POINTS* 15
GAMES := 0
end if
end if
|
It says all the variables don't have a value when i make VOID1 ice arrow or ice arrows. |
|
|
|
|
 |
TheGuardian001
|
Posted: Sun Apr 05, 2009 12:02 pm Post subject: Re: I need some help with a project. |
|
|
if you want your strings to have a space in them (like in ice arrows) you must either surround them in quotes when inputting them, or use
by default, Turing stops reading input after a space. adding :* will stop this from happening. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Nemo296
|
Posted: Sun Apr 05, 2009 12:19 pm Post subject: RE:I need some help with a project. |
|
|
Omfg, thank you so much =D
It worked. |
|
|
|
|
 |
Nemo296
|
Posted: Sun Apr 05, 2009 4:36 pm Post subject: RE:I need some help with a project. |
|
|
Ok, one more thing. I thank everyone who's helped me.
I get this error when I try to run the stand alone program.
Bad object File Header in "project.exe" . (2)
Heres my FULL program.
Turing: |
var VOID, VOID1, STAT, B : string
var NUMBER, POINTS, AMP, A, LEVEL, EXP : int
var AMOUNT, GAMES, EXPNEEDED : real
loop
put "Please enter how many points you have"
get POINTS
put "Enter what you want"
put "Items or Exp"
get VOID
if Str.Lower (VOID ) = "items" then
put "Please enter the item you wish to have."
put "Void Armour, Dragon weapon set, BGS or Ice arrows."
get VOID1 : *
if Str.Lower (VOID1 ) = "void armour" then
AMP := 150 - POINTS
A := 150
AMOUNT := POINTS / 150
GAMES := AMP / 2
elsif
Str.Lower (VOID1 ) = "bgs" then
AMP := 100 - POINTS
A := 100
AMOUNT := POINTS / 100
GAMES := AMP / 2
elsif
Str.Lower (VOID1 ) = "ice arrows" or Str.Lower (VOID1 ) = "ice arrow" then
AMP := 1 - POINTS
A := 1
AMOUNT := POINTS * 15
GAMES := 0
elsif
Str.Lower (VOID1 ) = "dragon weapon" or Str.Lower (VOID1 ) = "dragon weapons" then
AMP := 5 - POINTS
A := 5
AMOUNT := POINTS
GAMES := AMP / 2
end if
put "You have ", POINTS, " Points and you want to buy ", VOID1, "."
if AMOUNT >= 1 then
put "You have enough for ", AMOUNT, " ", VOID1
else
put "You need ", AMP, " to get a ", VOID1
put "Or you need to play ", GAMES, " games for that item."
end if
end if
if Str.Lower (VOID ) = "exp" then
put "Please enter the stat you want to get up"
put "Please use, Attack-Strength-Defence-Range-Mage-Prayer."
put "No short forms please"
get STAT : *
if Str.Lower (STAT ) = "strength" then
put "What is your Strength exp?"
get EXP
elsif Str.Lower (STAT ) = "attack" then
put "What is your Attack exp?"
get EXP
elsif Str.Lower (STAT ) = "defence" then
put "What is your Defence exp?"
get EXP
elsif Str.Lower (STAT ) = "mage" then
put "What is your Magic exp?"
get EXP
elsif Str.Lower (STAT ) = "range" then
put "What is your Range exp?"
get EXP
elsif Str.Lower (STAT ) = "prayer" then
put "What is your Prayer exp?"
get EXP
end if
put "Please enter the exp you want, 0 - 13,034,431"
get LEVEL
var GAMEEXP : int := 12
EXPNEEDED := (((LEVEL - EXP ) / GAMEEXP ) - POINTS ) / 2
put "You need to play ", EXPNEEDED, " games to get ", LEVEL - EXP, " exp."
put "Or you need ", ((LEVEL - EXP ) / GAMEEXP ) - POINTS, " points."
end if
put "Do you want to use the calculator again?"
get B
exit when Str.Lower (B ) = "n"
end loop
cls
put "Please close this window"
put "Thanks for using Nemo2966's Pest control calculator"
put "Please PM me if there are any errors"
|
|
|
|
|
|
 |
TheGuardian001
|
Posted: Sun Apr 05, 2009 6:08 pm Post subject: Re: I need some help with a project. |
|
|
The bad object header error means that you are using the version of Turing in which compiling is broken. Downloading a slightly older version (I think the one linked to at the top of the compsci.ca page works) and recompiling should fix this problem. |
|
|
|
|
 |
Tallguy

|
Posted: Mon Apr 06, 2009 12:46 pm Post subject: RE:I need some help with a project. |
|
|
i'm just very curious wat is the point of this code? |
|
|
|
|
 |
blankout
|
Posted: Mon Apr 06, 2009 3:04 pm Post subject: Re: I need some help with a project. |
|
|
hmmm, i asked this same question about a week ago, how funny |
|
|
|
|
 |
|
|