Babylonian method of Square roots
Author |
Message |
Thuged_Out_G
|
Posted: Mon Oct 27, 2003 10:39 pm Post subject: Babylonian method of Square roots |
|
|
the babylonia method for calculating square roots involves iteration. Suppose you wish to find the square root of 5. The babylonian method starts with a wild card guess which we will call x. You then use the iteration rule where the next x equals 0.5(x+n/x). For example, if we start with x=10, then the next x would be 0.5(10+5/10) or 5.25. We use that new value for x to determine the next value. This continues until x converges on a specific value. As the difference in x values becomes very small you now have an approximation for the square root of 5. The formula for any positive square root n is x0.5(x+n/x). Write a function that approximates the square root of a number using the babylonian method. You should stop iterations when the value doesnt change to 2 decimal places.
i have to have this function done for comp sci class....can anyone help?
thanks |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
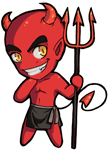
|
|
|
|
 |
Thuged_Out_G
|
Posted: Mon Oct 27, 2003 10:54 pm Post subject: (No subject) |
|
|
lol
i would, but i have to hand this is...its the final proggy for the functions unit lol |
|
|
|
|
 |
Dan
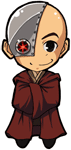
|
Posted: Mon Oct 27, 2003 10:57 pm Post subject: (No subject) |
|
|
well if x is a guess you whould probly whont to set it to a number graeter then the one you are finding the square root for. so set x to somting like: num to find square root of + 1. then just do thous math staments in your post in a loop that stops when the number is the the rigth range. you will most likey need to use real variables for this beacuse you need the demalses.
EDIT: dang tony and his fast posting |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Tony
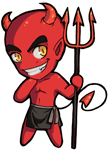
|
Posted: Mon Oct 27, 2003 10:59 pm Post subject: (No subject) |
|
|
well the function seems to be messed up...
actually you can try to trim the value to keep on going
like
code: |
put strreal(realstr(1.23456789011111111,0))
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Thuged_Out_G
|
Posted: Mon Oct 27, 2003 11:01 pm Post subject: (No subject) |
|
|
code: |
var winID:int
winID:=Window.Open("position:top,center,graphics:640;640")
function babylon(n:real):real
var i,x,x1,x2,x3:real
loop
i:=Rand.Int(5,10)
x:=0.5*(i + n/ i)
x1:=0.5*(x + n / x)
x2:=0.5*(x1 + n / x1)
x3:=0.5*(x2 + n / x2)
result x3
end loop
end babylon
var n:real
for root:1..1000000
put "Enter in the number(0 to exit): "..
get n
put babylon(n)
if n=0 then
loop
Window.Close(winID)
end loop
end if
end for
|
thats what i have so far, and it works....but it just gets less accurate the higher the number you put...it seems towork for values up to 10,000[/code] |
|
|
|
|
 |
Tony
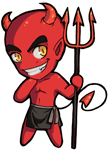
|
Posted: Mon Oct 27, 2003 11:08 pm Post subject: (No subject) |
|
|
that's because for values of 10,000, you need to calculate new value more then 3 times (meaning have x4).
and why is there a loop in function? It NEVER gets to endloop because of result |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|