loading pics into an array with a for loop
Author |
Message |
Insectoid

|
Posted: Thu Aug 07, 2008 4:12 pm Post subject: loading pics into an array with a for loop |
|
|
How can I load up pictures automatically into an array with a for loop? I have pictures named 'bar (1)', 'bar (2)', etc, and want to load them into an array 1..10 of int.
I tried this:
code: |
var bars : array 1..10 of int
for x: 1..10
bars (x) := Pic.FileNew ("bar ("x").bmp")
end for
|
which obviously doesn't work (I tried it for the small chance it would.)
I need a way to sub in the picture's number for 'x'.
Help?
~Insectoid
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
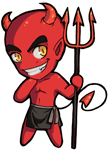
|
Posted: Thu Aug 07, 2008 4:23 pm Post subject: RE:loading pics into an array with a for loop |
|
|
code: |
"bar (" + intstr(x) + ").bmp"
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Thu Aug 07, 2008 11:31 pm Post subject: RE:loading pics into an array with a for loop |
|
|
There are a number of standard patterns used for inserting the values of variables into a string object (usually for output, though they have other uses, as you've shown).
First, there's concatenation, which is essentially "joining sequentially". This is what Tony suggested, and goes like
code: | string A = "there are" + posts + " posts on this forum"
// (A contains "there are 23989 posts on this forum") |
Different languages use different operators. In Turing, you can use + in all cases, but you can also use commas in put and write statements. C++ uses << operators for their IO streams, Java uses +, and (I think it was PHP) uses &.
Second, there's substitution, which is a more common pattern in languages like C. You'd see something like:
[syntax=C]
printf ( "There are %d posts on this forum", posts );
[/syntax]
In this case, there are special identifiers (%d here) that are replaced by the other arguments to the function (the variable posts). The form of the identifier dictates the output type (decimal-formatted integer, for %d).
Long explanation for a short solution: just do what Tony said.
|
|
|
|
|
 |
Insectoid

|
Posted: Fri Aug 08, 2008 8:05 am Post subject: RE:loading pics into an array with a for loop |
|
|
Well.... I didn't think of that....(Stupid stupid stupid me)
It's so obvious (now).
|
|
|
|
|
 |
Insectoid

|
Posted: Fri Aug 08, 2008 11:37 pm Post subject: Re: loading pics into an array with a for loop |
|
|
Okay, so Turing is being a bit stupid now. It won't let me load any more pictures. Is there a maximum amount of pics that can be loaded at once into Turing? I have 13 right now and need 15. I tried loading different pics, changing the name & file type, but it won't work! The code is exactly the same as the ones that DID work, and the picture is in the same folder, and it really is pissing me right off. Help?
|
|
|
|
|
 |
Tony
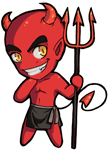
|
Posted: Fri Aug 08, 2008 11:45 pm Post subject: Re: loading pics into an array with a for loop |
|
|
insectoid @ Fri Aug 08, 2008 11:37 pm wrote: Is there a maximum amount of pics that can be loaded at once into Turing?
Yes -- 1000. Sounds like this isn't the case, unless your picture loading for-loop is inside another loop.
Try loading pictures one at a time, just to make sure that you got the name of it correct, and that the file is accessible.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Sat Aug 09, 2008 12:05 am Post subject: RE:loading pics into an array with a for loop |
|
|
the first 13 pics work fine, it's just the last 2 I did the next day that won't.
is 1000 the max number of images you can have loaded at one time or if you swap the picture stored in a variable will that increase the count? (something is odd about that sentence...hmm...)
|
|
|
|
|
 |
Tony
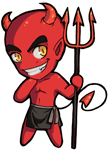
|
Posted: Sat Aug 09, 2008 12:11 am Post subject: RE:loading pics into an array with a for loop |
|
|
If you were to load the last 2, without the first 13, does that work? It sounds like there's some small detail that you might be missing.
The 1000 limit is the number of times you can call Pic.New before having to call Pic.Free.
If you notice, the variables that "hold" the pictures are simple int variables, and they hold an integer ID that references the picture, loaded elsewhere. That elsewhere can have a max of 1000.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
gitoxa

|
Posted: Sat Aug 09, 2008 12:46 am Post subject: RE:loading pics into an array with a for loop |
|
|
Simple test. Create a new file, make a for loop to assign a single picture to each element of your array. First try up to 1000, then try 1001.
You can have up to 1000. The problem lies elsewhere in your program. Either like Tony said, perhaps your initializing loop is inside another loop. Or perhaps you're just using the pictures elsewhere, and have hit the limit already.
|
|
|
|
|
 |
Insectoid

|
Posted: Sat Aug 09, 2008 9:24 am Post subject: Re: loading pics into an array with a for loop |
|
|
Actually, the limit shouldn't be a problem, because the pictures in question are loaded at the start with my global variables, before the for loop, which is not in a loop. The for loop is the first thing the program executes after initiating global variables.
I tried putting Pic.Free before re-initiating the variable to a different picture, but that still didn't work.
Anyways, I'll post my project, maybe you guys can find the problem.
EDIT: I made a new test file,
code: |
var picture := Pic.FileNew ("mario_fall.bmp")
Pic.Draw (picture, 0, 0, picCopy)
|
This one also would not load the picture, but when I switched mario_fall with mario_left, it worked fine. Perhaps Turing just doesn't like the picture?
Description: |
|
 Download |
Filename: |
unnamed game.zip |
Filesize: |
24.2 KB |
Downloaded: |
101 Time(s) |
|
|
|
|
|
 |
Insectoid

|
Posted: Sat Aug 09, 2008 9:54 am Post subject: RE:loading pics into an array with a for loop |
|
|
Okay, I found the problem. It was the way I took the pictures off the sprite sheet and converted them from GIF to BMP. With the first 3 pictures off the sheet, I copy & pasted them into a new window in Paint, then they automatically converted to BMP. With the pictures that didn't work, I copied them, the selected File/New in Paint and pasted them there, then converted to BMP. Somehow it makes a difference.
|
|
|
|
|
 |
|
|