Is bad to have a variable decalaration inside a loop?
Author |
Message |
riveryu

|
Posted: Fri Jun 06, 2008 5:15 pm Post subject: Is bad to have a variable decalaration inside a loop? |
|
|
Question is mentioned in title. I noticed it from the tutorial on the perfect "following"
Turing: |
View.Set ("offscreenonly")
type Position :
record
x, y : real
end record
const ENEMY_MOVEMENT_SPEED := 1 %% This is the same as the projection length (Same as |Direction|)
var enemy : Position := init (200, 200)
loop
var mouseX, mouseY, mouseButtonState : int
Mouse.Where (mouseX, mouseY, mouseButtonState )
var distance := Math.Distance (mouseX, mouseY, enemy.x, enemy.y )
var deltaX := mouseX - enemy.x
var deltaY := mouseY - enemy.y
%% Here we plug into the equations
var xDir := (deltaX * ENEMY_MOVEMENT_SPEED ) / distance
var yDir := (deltaY * ENEMY_MOVEMENT_SPEED ) / distance
enemy.x + = xDir
enemy.y + = yDir
Draw.FillOval (round (enemy.x ), round (enemy.y ), 5, 5, black)
View.Update ()
Time.Delay (10)
cls ()
end loop |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
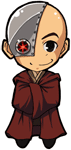
|
Posted: Fri Jun 06, 2008 6:28 pm Post subject: RE:Is bad to have a variable decalaration inside a loop? |
|
|
Well if you declare a varaible in a loop it will noraml have scope just in that loop and can not be accessed out side of it. Also that variable will be reset every interation of the loop.
So in my option if the varibale is only used in the loop and needs to be changed every time the loop goes around it is a good thing to have it in the loop.
However depending on the langue and the aviablity and quality of grabage collectors it might not be if that alocated memeory is hanging around affter it goeses out of scope. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
rdrake

|
Posted: Fri Jun 06, 2008 9:01 pm Post subject: RE:Is bad to have a variable decalaration inside a loop? |
|
|
Like Dan says, it depends. If it's not going to be used anywhere but inside the loop it's best to stick the variable declaration inside there. This is known as encapsulation; you are hiding unnecessary information from other parts of your program. |
|
|
|
|
 |
r691175002
|
Posted: Fri Jun 06, 2008 9:25 pm Post subject: Re: Is bad to have a variable decalaration inside a loop? |
|
|
It really depends, I generally suggest keeping it outside the loop, just because in some of the languages I use the performance lost to redeclaring the same variables each iteration is substantial. If you are dealing with classes that can be reused the performance difference is incredible.
Something like this:
code: | var position:Point = new Point();
for (var i:int = 0; i < 1000; i++) {
position.x = 100;
position.y = 100;
doSomething(position);
andSomethingElse(position);
} |
Will run orders of magnitude faster than the following:
code: | for (var i:int = 0; i < 1000; i++) {
var position:Point = new Point(100,100);
doSomething(position);
andSomethingElse(position);
} |
I prefer having them inside the loop, but reality sometimes gets in the way. |
|
|
|
|
 |
jeffgreco13

|
Posted: Mon Jun 09, 2008 9:03 am Post subject: Re: Is bad to have a variable decalaration inside a loop? |
|
|
r6911's got the best idea in my opinion. While both methods work, you're better off declaring it outside of the loop and just resetting the values.
r691175002 wrote:
Something like this:
code: | var position:Point = new Point();
for (var i:int = 0; i < 1000; i++) {
position.x = 100;
position.y = 100;
doSomething(position);
andSomethingElse(position);
} |
Optimization is key, maybe not in Turing because optimal programming is using a completely different language. But, you should still make your program as efficient as possible. Calling 'var' requests the processor to create a new place in the memory where your value will be held. Calling it over and over in a loop requests a lot more work than just setting the values back to a set value at the end of the loop (or beginning). |
|
|
|
|
 |
DemonWasp
|
Posted: Thu Jun 12, 2008 8:48 pm Post subject: RE:Is bad to have a variable decalaration inside a loop? |
|
|
This really, really depends. In smarter interpreters, the difference can actually be near-zero between the two, since it can detect that object is redeclared at the start of the loop, and just new the object in-place.
If there's no initialization there, then the compiler/interpreter can actually pull the variable declaration completely out of the loop in the code that actually gets executed.
In Turing you're probably better off writing the optimisation yourself. I wouldn't rely on the Turing runtime to crack a peanut for me. Tell it exactly what you want from it, and it generally does a decent job delivering. |
|
|
|
|
 |
riveryu

|
Posted: Thu Jun 12, 2008 9:49 pm Post subject: RE:Is bad to have a variable decalaration inside a loop? |
|
|
I think i'll keep it outside most of the time. Thanks for answering guys. |
|
|
|
|
 |
|
|