Selecting objects.
Author |
Message |
metachief

|
Posted: Wed May 07, 2008 10:25 pm Post subject: Selecting objects. |
|
|
Hello, I am working on R.T.S. and I can't figure out how to select oneobject a a time. What I mean is: I have 3 circles on the screen and I want to have one change color by selecting it with the mouse. I want it to be something like selecting units in warcraft for examle. I'm not having trouble with the visual part. I need the concept. What variales would I have as arrays and where will I use a for loop statement? Do I need to use types for each unit? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
lordroba

|
Posted: Wed May 07, 2008 10:59 pm Post subject: Re: Selecting objects. |
|
|
An easy way to do it would be to set up an array with records
Turing: |
var cUnit : array 1 .. 100 of
record
x : int
y : int
selected : boolean
hp : int
%and so on...
end record
for i : 1 .. 100
cUnit (i).selected := false
end for
|
I would initially declare all units as non selected, and then when you click on a unit, (you'd figure this out by their position on the map) you'd change that unit's selected value to true. for example cUnit(23).selected:=true Then you could use if conditions with that. Say for example...
Turing: |
if cUnit(i).selected=true then
%make that unit appear highlighted on screen
|
|
|
|
|
|
 |
metachief

|
Posted: Thu May 08, 2008 9:46 am Post subject: RE:Selecting objects. |
|
|
Well, you din't quite answer my question, but you gave me a better way of selecting single units (I had that covered, but using records is probably better so i'll try that)
The thing that I was having troubble with was selecting multiple units at the same time. I mean highliting them. You know how in warcraft you can select many of them by clicking on the mouse and dragging it to make a square over them and if they are in that square then they are selected. Well that is what I'm trying to do.
How do I figure out weither they are in the area of the squareor not? |
|
|
|
|
 |
Tony
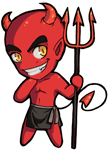
|
Posted: Thu May 08, 2008 10:09 am Post subject: Re: RE:Selecting objects. |
|
|
metachief @ Thu May 08, 2008 9:46 am wrote: How do I figure out weither they are in the area of the squareor not?
It's much easier to figure this out if you draw a rectangle on paper then hit random dots and ask yourself "is this inside?"
And no, "well it looks like it's inside" doesn't count. Try to work out a set of math rules that make the statement definitively true. (Things like "the point is to the left of this rectangle's side" or "the point is this far away from a vertex"). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
lordroba

|
Posted: Thu May 08, 2008 11:02 am Post subject: Re: Selecting objects. |
|
|
needless to say, I was somewhat bored...
I copied the Mouse.ButtonWait code from the help files and changed the lines to boxes.
Basically with this code, it first checks from which direction the box is drawn, and then it checks if the circles are within that box...
Turing: |
var cUnit : array 1 .. 10 of
record
x : int
y : int
selected : boolean
hp : int
%and so on...
end record
for i : 1 .. 10
cUnit (i ).selected := false
end for
cUnit (1).x := 110
cUnit (1).y := 110
cUnit (2).x := 120
cUnit (2).y := 20
cUnit (3).x := 30
cUnit (3).y := 130
for i : 1 .. 3
drawoval (cUnit (i ).x, cUnit (i ).y, 5, 5, 9)
end for
var x, y, btnNumber, btnUpDown, buttons : int
var nx, ny : int
loop
Mouse.ButtonWait ("down", x, y, btnNumber, btnUpDown )
nx := x
ny := y
loop
Draw.Box (x, y, nx, ny, 0) % Erase previous line
exit when Mouse.ButtonMoved ("up")
Mouse.Where (nx, ny, buttons )
for i : 1 .. 3
drawoval (cUnit (i ).x, cUnit (i ).y, 5, 5, 9)
end for
Draw.Box (x, y, nx, ny, 1) % Draw line to position
end loop
Mouse.ButtonWait ("up", nx, ny, btnNumber, btnUpDown )
Draw.Box (x, y, nx, ny, 2) % Draw line to final position
for i : 1 .. 3
if nx > x then
if cUnit (i ).x < nx and cUnit (i ).x > x then
if ny > y then
if cUnit (i ).y < ny and cUnit (i ).y > y then
cUnit (i ).selected := true
else
cUnit (i ).selected := false
end if
end if
if ny < y then
if cUnit (i ).y > ny and cUnit (i ).y < y then
cUnit (i ).selected := true
else
cUnit (i ).selected := false
end if
end if
else
cUnit (i ).selected := false
end if
elsif nx < x then
if cUnit (i ).x > nx and cUnit (i ).x < x then
if ny > y then
if cUnit (i ).y < ny and cUnit (i ).y > y then
cUnit (i ).selected := true
else
cUnit (i ).selected := false
end if
end if
if ny < y then
if cUnit (i ).y > ny and cUnit (i ).y < y then
cUnit (i ).selected := true
else
cUnit (i ).selected := false
end if
end if
else
cUnit (i ).selected := false
end if
end if
cls
for v : 1 .. 3
if cUnit (v ).selected = true then
drawfilloval (cUnit (v ).x, cUnit (v ).y, 5, 5, 9)
else
drawoval (cUnit (v ).x, cUnit (v ).y, 5, 5, 9)
end if
end for
end for
end loop
|
if you draw a box somewhere else, your units will be deselected |
|
|
|
|
 |
metachief

|
Posted: Thu May 08, 2008 2:51 pm Post subject: RE:Selecting objects. |
|
|
Cool, I never heard of Muse.ButtonWait. Althought you would probably agree that that's a lot of code just to select units. Thanks anyway, but I'm going to try to figure out a more clear and efficient way. Perhaps after experimenting with equations i'll get it. If I do I'll inform you as well. |
|
|
|
|
 |
|
|