Author |
Message |
rcmp1234
|
Posted: Thu May 01, 2008 8:19 pm Post subject: the "Getch" procedure |
|
|
Turing: |
procedure inputletter
getch (reply )
if reply = "e" then
menu
end if
end inputletter
|
*Note that menu is a procedure and has been declared before*
Here I have a part of my program that asks the user to press a key. If the user presses e then he will be taken back to the menu. The problem is if he presses another letter the program will still keep on executing. Is there any way I can have the program wait for the user to press e (and does not continue executing if he presses another key)?
I was thinking of putting it in a loop but you cant put a procedure in a loop
ANd yes, I am a newbie in Turing
Thanks! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
The_Bean

|
Posted: Thu May 01, 2008 8:37 pm Post subject: Re: the "Getch" procedure |
|
|
Turing: |
procedure inputletter
getch (reply )
if reply = "e" then
menu
else
doSomethingElse
end if
end inputletter
|
I just added in the "else" which means if it reply= anything but "e" then it does something else(what ever you might want it to be).
But if you want the program to totally terminate if they put anything but "e", then work exit, return, or quit in there some how (depending on what the rest of your program is doing). |
|
|
|
|
 |
TheGuardian001
|
Posted: Fri May 02, 2008 4:06 pm Post subject: Re: the "Getch" procedure |
|
|
you could even use The_Bean's method to have the procedure call itself if they don't enter "e" |
|
|
|
|
 |
Nick

|
Posted: Fri May 02, 2008 4:08 pm Post subject: RE:the "Getch" procedure |
|
|
or a loop inside the procedure
Turing: | procedure inputletter
loop
getch (reply )
if reply = "e" then
menu
return
end if
end loop
end inputletter |
|
|
|
|
|
 |
Tony
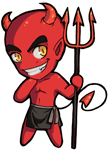
|
Posted: Fri May 02, 2008 4:20 pm Post subject: RE:the "Getch" procedure |
|
|
or call procedure after the loop
Turing: |
procedure inputletter
loop
getch (reply )
exit when reply = "e"
end loop
menu
end inputletter
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
btiffin

|
Posted: Fri May 02, 2008 5:16 pm Post subject: Re: the "Getch" procedure |
|
|
These are all good answers so far.
Do a google on Structured Programming for more info, but basically you want to use a
DO
...
UNTIL
structure for that type of problem.
DO
get a key
UNTIL key equals "e"
Converting that to Turing will be left as an exercise.
In purist terms, a
LOOP
IF ... RETURN ENDIF
END LOOP is defined as a "hidden goto"; not horribly bad form, and quite acceptable now-a-days, but a DO ... UNTIL is just that little bit more structured, and usually more efficient, as there are no magical internal stack winds and unwinds or jumps going on behind the scenes.
Cheers |
|
|
|
|
 |
rcmp1234
|
Posted: Fri May 02, 2008 5:38 pm Post subject: RE:the "Getch" procedure |
|
|
Thanks everyone...that did the trick! |
|
|
|
|
 |
btiffin

|
Posted: Fri May 02, 2008 5:39 pm Post subject: Re: the "Getch" procedure |
|
|
My apologies to Tony. His code may well be the "converting this to Turing will be left as exercise" part. Excuse my lack of Turing syntax details.
Cheers |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
|