Collision detection issues...
Author |
Message |
Insectoid

|
Posted: Thu Apr 24, 2008 8:49 pm Post subject: Collision detection issues... |
|
|
I just learned today how to do character movement from a tutorial on this site somewhere and well, movement isn't usefull without collision detection.
However, My mini-project for fun seems to do something...wrong. The little red circle (controlled by arrow keys) is supposed to hit a black dot, which is then randomly placed somewhere else on the screen. What happens instead is that once the red dot crosses the x- axis of the black dot and subsequently crosses the y- axis, THEN it moves the black dot.
Here's my code:
Turing: |
var charx : int := 100
var chary : int := 100
var targetx : int
var targety : int
var num : array char of boolean
loop
targetx := Rand.Int (10, 630)
targety := Rand.Int (10, 390)
loop
exit when charx + 6 >= targetx + 6 and chary + 6 >= targety + 6
Draw.FillOval (targetx, targety, 5, 5, black)
Draw.Oval (charx, chary, 5, 5, brightred)
Input.KeyDown (num )
if num (KEY_UP_ARROW) and chary < 390 then
chary := chary + 5
end if
if num (KEY_DOWN_ARROW) and chary > 10 then
chary := chary - 5
end if
if num (KEY_LEFT_ARROW) and charx > 10 then
charx := charx - 5
end if
if num (KEY_RIGHT_ARROW) and charx < 630 then
charx := charx + 5
end if
delay (10)
cls
View.Update
end loop
end loop
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
btiffin

|
Posted: Thu Apr 24, 2008 9:59 pm Post subject: Re: Collision detection issues... |
|
|
Hi,
I don't know Turing and should probably leave this to some of the other compsci members, but;
Your charx targetx test is ANDed with the chary targety test. Would OR be more appropriate?
Cheers from the clueless old guy. |
|
|
|
|
 |
Tony
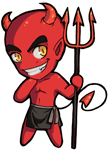
|
Posted: Thu Apr 24, 2008 10:24 pm Post subject: RE:Collision detection issues... |
|
|
@btiffin -- I'm sure that you could still read that code
@insectoid -- read your condition statement. You are checking if char is to the right and above the target. There's quite a lot of area in that box.
also: charx + 6 >= targetx + 6 is the same as
charx >= targetx. Less magic numbers makes more sense.
If you were to follow the tutorials to read up on the collision detection, you'd learn that collision occurs when two objects overlap. That is, the right-most point of A is to the right of the left-most point of B and the left-most point of A is to the left of the right-most point of B and similar set of conditions for the Y-axis.
That's a lot to check. It's also a square shape. A shortcut for two circles is that collision occurs when the distance between the centers is less than the sum of the radiuses.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
btiffin

|
Posted: Thu Apr 24, 2008 11:44 pm Post subject: Re: RE:Collision detection issues... |
|
|
Tony @ Thu Apr 24, 2008 10:24 pm wrote: @btiffin -- I'm sure that you could still read that code  Well Tony, I must say; hanging out here, I'm going to have to unfurl a Turing and take it for a spin. It seems to inspire. It sure doesn't hurt having smart people that seem to have this stuff down cold to bounce questions off either. So far I'm learning by osmosis.
Cheers |
|
|
|
|
 |
Insectoid

|
Posted: Fri Apr 25, 2008 7:42 am Post subject: RE:Collision detection issues... |
|
|
Thanks Tony. I did check the tutorial, but didn't find it helpful as it was...not very good, and painful to read (Dan has to work on his spelling). As I said, this is my first time going outside text and letters moving in a pre-determined route around the screen.
Being the kind of guy I am, I would now like to know what Math.Distance does. (I suppose it calculates the distance between the points you put in parantheses) |
|
|
|
|
 |
gitoxa

|
Posted: Fri Apr 25, 2008 5:15 pm Post subject: Re: Collision detection issues... |
|
|
Math.Distance finds the distance between two points (x1, y1) and (x2, y2) using the distance formula
distance = square root of( (x2 - x1)^2 + (y2 - y1)^2 ) |
|
|
|
|
 |
|
|