For loop a string? (Super noob at Turing, Gr.10 Prog)
Author |
Message |
jinjin
|
Posted: Sun Mar 16, 2008 12:27 pm Post subject: For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Sorry if this is a stupid question but really stuck on this as part of my Gr.10 programming class. The program's function is that it must ask the user for a word and then ask how many times to print it. Then, it should loop the inputted word by the inputted number of times using a for loop. The problem is that I don't know how to for loop a string by an integer. I wrote it in normal loop first:
code: |
var word : string
var times, c : real
put "Please enter a word."
get word
put "How many times do you want to print this word?"
get times
c := 1
loop
put word
exit when c = times
c := c + 1
end loop
|
and this above code works but when I try using for loop ...
code: |
var word : string
var times : int
put "Please enter a word"
get word
put "How many times do you want to print this word?"
get times
for c : word .. times
put c
end for
|
... I get the error " 'for' range bounds must be both integers, chars or elements of the same type..."
However, I can't make them both integers in this case so I am not sure what to do.
Any help is appreciated, thanks
Regards,
jinjin |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Mackie

|
Posted: Sun Mar 16, 2008 12:34 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Why are you going from word .. times? A for loop is a counter. Can you count from Foobar to 60? Think about how the syntax of the counter works.
code: | for i : fromThisNumber .. toThisOne |
So to count from zero you would put 0 in the first position, followed by the number you want to count to. |
|
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 12:47 pm Post subject: Re: For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Mackie wrote:
Why are you going from word .. times? A for loop is a counter. Can you count from Foobar to 60? Think about how the syntax of the counter works.
code: | for i : fromThisNumber .. toThisOne |
So to count from zero you would put 0 in the first position, followed by the number you want to count to.
Thanks for this advice. I am still slightly confused though; I understand now that you can't count from word to number so then it would have to be word multiplied by number. However, I have no idea how to have a word multiplied by a number using a for loop in Turing. |
|
|
|
|
 |
Mackie

|
Posted: Sun Mar 16, 2008 12:53 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Well, since the loop is looping, as in doing the same thing a number of times. All you need to do is put the string in the loop. Count from one to the total number of times you want it to display. |
|
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 1:04 pm Post subject: Re: For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Thanks for the quick reply but I'm not sure if I understand.
If I count from one to times...
code: |
var word : string
var times : int
put "Please enter a word"
get word
put "How many times do you want to print this word?"
get times
for c : 1 .. times
put c
end for
|
... I just get the number 1 to the inputted number for times. I'm not sure if this is what you meant  |
|
|
|
|
 |
Mackie

|
Posted: Sun Mar 16, 2008 1:09 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Try putting word, rather than c. |
|
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 1:14 pm Post subject: Re: For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Mackie wrote:
Try putting word, rather than c.
Ok, now I think Turing hates me.
With this
code: |
var word : string
var times : int
put "Please enter a word"
get word
put "How many times do you want to print this word?"
get times
for word : 1 .. times
put word
end for
|
It says "word has been previously declared." So, I remove the declaration and it says "word has not been declared." So, I just ended up in a neverending cycle aaahh. |
|
|
|
|
 |
Mackie

|
Posted: Sun Mar 16, 2008 1:18 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
The variable declared in the for loop, doesn't need to be used. All it does is tell you what number of loop you are on. Keep what you have there. Just change the 'word' right after the 'for' to something else. See what happens. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
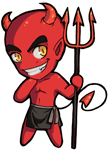
|
Posted: Sun Mar 16, 2008 1:19 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
you removed the declaration from the wrong place. Try removing the _other_ declaration of "word" (refer to your previous code). |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 1:27 pm Post subject: Re: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Mackie @ Sun Mar 16, 2008 1:18 pm wrote: The variable declared in the for loop, doesn't need to be used. All it does is tell you what number of loop you are on. Keep what you have there. Just change the 'word' right after the 'for' to something else. See what happens.
If I change the word right after for, it works but all it does is counts from #1 to the user inputted number. (i.e. I put the word elephant and say it to repeat 5 times, the output is "1 2 3 4 5")
Tony wrote:
you removed the declaration from the wrong place. Try removing the _other_ declaration of "word" (refer to your previous code).
I don't understand... what is the _other_ declaration? I thought the only declaration for word was "var : word"?
Mackie @ Sun Mar 16, 2008 1:18 pm wrote: The variable declared in the for loop, doesn't need to be used. All it does is tell you what number of loop you are on. Keep what you have there. Just change the 'word' right after the 'for' to something else. See what happens.
If I change the word right after for, it works but all it does is counts from #1 to the user inputted number. (i.e. I put the word elephant and say it to repeat 5 times, the output is "1 2 3 4 5")
Tony wrote:
you removed the declaration from the wrong place. Try removing the _other_ declaration of "word" (refer to your previous code).
I don't understand... what is the _other_ declaration? I thought the only declaration for word was "var : word"?
edit: my next reply might take a while, I'll be back in ~10 minutes |
|
|
|
|
 |
Mackie

|
Posted: Sun Mar 16, 2008 1:35 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Ok try changing the variable after 'for' to like 'c' or something. Now 'put word' don't 'put c'! |
|
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 2:11 pm Post subject: Re: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Mackie @ Sun Mar 16, 2008 1:35 pm wrote: Ok try changing the variable after 'for' to like 'c' or something. Now 'put word' don't 'put c'!
MACKIE, YOU ARE A GENIUS! 'put word' was the answer to the problem! Thanks
Final working program for anyone else's reference
code: |
%% Credits to Mackie
var word : string
var times : int
put "Please enter a word."
get word
put "How many times do you want to print this word?"
get times
for c : 1 .. times
put word
end for
|
|
|
|
|
|
 |
Tony
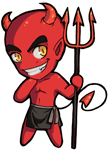
|
Posted: Sun Mar 16, 2008 2:52 pm Post subject: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
@Mackie -- you are just giving out solutions without any explanation.
@jinjin -- there are multiple ways of declaring a variable. The conventional use is, as you've stated, var variable_name : type
Though for-loops require a variable counter, so it creates one for you from just a name
code: |
for variable_name : start .. finish
put variable_name
end for
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
jinjin
|
Posted: Sun Mar 16, 2008 3:42 pm Post subject: Re: RE:For loop a string? (Super noob at Turing, Gr.10 Prog) |
|
|
Tony @ Sun Mar 16, 2008 2:52 pm wrote:
Though for-loops require a variable counter, so it creates one for you from just a name
code: |
for variable_name : start .. finish
put variable_name
end for
|
Thanks for clarifying that Tony  |
|
|
|
|
 |
|
|