Needing some tips
Author |
Message |
ItsCristian69
|
Posted: Thu Dec 13, 2007 4:57 pm Post subject: Needing some tips |
|
|
Hello everyone, I am currently using turing and for a school project, I must make a quiz/game show. I want to remake 'who wants to be a millionaire'.
My question is, what commands will i have to know? I know the basics, but if anyone could tell me how to do randomizing, arrays (i just heard about it) and maybe mouse overs, and in what ways they should be added to the program, i would really appreciate it.
Thank you in advance. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
HeavenAgain

|
Posted: Thu Dec 13, 2007 5:06 pm Post subject: RE:Needing some tips |
|
|
array always confuse me when i started programming, if you just started, i dont suggest you to jump into array right away, so what i'll suggest is:
loops
randomize
if statements
procedures/functions
(graphics) this will be more work, and could be confusing
(read files) for your questions, again, this is not necessary
and to do random, its pretty easy, there are two ways to do it
randint (variable_name, min, max)
var randomNumber : int := Rand.Int (min, max)
and of course you'll need if statements after this |
|
|
|
|
 |
Tony
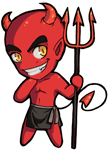
|
Posted: Thu Dec 13, 2007 5:07 pm Post subject: RE:Needing some tips |
|
|
it should be possible to put a game together with just the basics. "knowing commands" is not the right approach. "understanding commands" allows you to do new things in new ways.
It's not like you can just throw a bunch of blocks of code together and expect a game to come out.
You should probably look over The Turing Walkthrough in the Tutorials Section. It has links to tutorials of all the important concepts. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nick

|
Posted: Thu Dec 13, 2007 5:09 pm Post subject: RE:Needing some tips |
|
|
the turing help feature (hit F10 in the editor window) is great!
for what you need is Mouse.Where, Rand.Int, and I find they don't give enough information for a begginer to learn arrays...
so heres a breif discription of my own:
arrays are regular varibles with mulitple values
a sample code code: | var pic1,pic2,pic3:int |
can be replaced with code: | var pic:array 1..3 of int |
to call the newly foremed varible you use the following
code: | put pic(1)
put pic(2)
put pic(3) |
It's as simple as that  |
|
|
|
|
 |
ItsCristian69
|
Posted: Thu Dec 13, 2007 5:11 pm Post subject: Re: Needing some tips |
|
|
Thanks guys! I will get right to it.
By the way, i have taken pictures from the tv show and photoshopped them so they appear blank, i want to use them as a template.
I am sort of a beginner at Turing, but i know the basics. I am just debating now whether i should do mouse overs for the user's input, or they type in the answer.
What do you guys think?
Thanks. |
|
|
|
|
 |
HeavenAgain

|
Posted: Thu Dec 13, 2007 5:13 pm Post subject: RE:Needing some tips |
|
|
of course, the less typing the better less error could happen and way better presenting the game
and wow, you are serious, even took pictures! im sure you couldve found some pictures from google  |
|
|
|
|
 |
Nick

|
Posted: Thu Dec 13, 2007 5:13 pm Post subject: RE:Needing some tips |
|
|
well roll overs will stop the user from screwing up the program (example typing a string instead of a int)
also it stops the user from mistyping something
not to mention it a) makes the program looks nicer
b) makes YOU look smarter  |
|
|
|
|
 |
ItsCristian69
|
Posted: Thu Dec 13, 2007 5:15 pm Post subject: Re: Needing some tips |
|
|
I think I have a basic ideas of arrays now... but you assigned the value of an integer to pic 1 .. 3, do all of them have the same value? what makes them different? and why would i use arrays?
Yes i do want the program to make me look smarter than everyone else in the class
Thanks for the feedback everyone, i really appreciate it!  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Nick

|
Posted: Thu Dec 13, 2007 5:20 pm Post subject: RE:Needing some tips |
|
|
well the great things about arrays are: (man whats with me and lists today?)
a) they DONT hold the same value (EX. pic(1) can contain the main character's picture whereas pic(2) could contain an enemy picture)
b) they make the code much more efficeint
EX. instead of code: | pic1:=Pic.New(yadda yadda)
pic2:=Pic.New(yadda yadda)
pic3:=Pic.New(yadda yadda) |
you can simply type code: | for i:1..3
pic(i) := Pic.FileNew("picNum("+intstr(i)+").bmp")
end for |
now imagine the above with 100 or even 1000 varibles! |
|
|
|
|
 |
HeavenAgain

|
Posted: Thu Dec 13, 2007 5:20 pm Post subject: RE:Needing some tips |
|
|
arrays are block of memory in the computer, and they can be assigned to any type, BUT it must be the same data type, and plus it is a FIXED size, which will not be changed after declaration
and you treat each element of array as a variable, they can hold the same information or differnt, its really up to you
you use array because they are cool and because the elements are easy to modify and theres thousands of algorithm for it, so yea, its cool |
|
|
|
|
 |
ItsCristian69
|
Posted: Thu Dec 13, 2007 5:23 pm Post subject: Re: Needing some tips |
|
|
Sounds more complicated than I thought , but ill try it out.
Ill get started on the program! thanks guys! |
|
|
|
|
 |
Clayton

|
Posted: Thu Dec 13, 2007 5:29 pm Post subject: RE:Needing some tips |
|
|
HeavenAgain wrote: arrays are block of memory in the computer, and they can be assigned to any type, BUT it must be the same data type, and plus it is a FIXED size, which will not be changed after declaration
Don't leave flexible arrays out of this party. |
|
|
|
|
 |
Nick

|
Posted: Thu Dec 13, 2007 5:32 pm Post subject: RE:Needing some tips |
|
|
yes yes flexible arrays those are the next best thing to vectors which sadly turing does not have
heres how to declare a flexible array its not much diffrent
code: | var pic:flexible array 1..3 of int |
but the added benefit of having the array flexible is reallocationg the upper demension... or in english increasing the size
(this is correct right?)
this will allow you to now use pic(4) since you created a new block of data on top of the origanal 3 |
|
|
|
|
 |
HeavenAgain

|
Posted: Thu Dec 13, 2007 5:33 pm Post subject: RE:Needing some tips |
|
|
yes... but we'll just pretend its not there, since he is already confused
but yea, flexible arrays are more advanced than the normal array (which is good enough for your case) |
|
|
|
|
 |
|
|