Directory Scanning
Author |
Message |
Prince Pwn
|
Posted: Sun Nov 05, 2006 9:54 am Post subject: Directory Scanning |
|
|
I'm attempting to make a program that will scan similar to an anti-spyware or something. I'm testing it on my 128MB USB key so it won't take for ever. Here is my code so far:
code: |
var dirName : string
var dir := "E:/"
var stream := Dir.Open (dir)
loop
for i : 1 .. stream
dirName := Dir.Get (stream)
if Dir.Exists (dirName) then
put dir
dir := dir + dirName
stream := Dir.Open (dir)
end if
end for
end loop
|
It gives me an error when it finds a dot which means back a directory or something... I want it to go through all my folders successfully and put them down the screen. Then eventually files (which shouldn't be hard). So can anyone help me see every folder without getting errors? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Expirant
|
Posted: Sun Nov 05, 2006 2:49 pm Post subject: (No subject) |
|
|
I think Turing puts a dot and then two dots at the beginning of the folder so you know it's the beginning (or something like that). I would just do something along the lines of: code: | if dir not= "." and dir not= ".." then... |
I think that's what you were asking. I don't have Turing on this machine so I can't exactly test your code...but I think some sort of if statement is in hand. |
|
|
|
|
 |
Dan
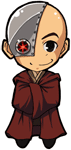
|
Posted: Sun Nov 05, 2006 2:52 pm Post subject: (No subject) |
|
|
You could simpley put in a if to check for a dir called "." or ".." and ingorner it. Also i whould recomend using a recursive aproch rather then using for loops if you whont to check very file on a computer or device.
I whould help more but i am geting the fealing this is going to be used for somthing unethical.... |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Cervantes

|
Posted: Sun Nov 05, 2006 2:53 pm Post subject: (No subject) |
|
|
You're doing a few things wrong here. First, you're trying to loop from 1 up to `stream'. I ask you, "What's the value of `stream'?" The value of stream is just some integer. It's not random, but it might seem like it. If the stream for C:\Windows is 1001, it doesn't mean the stream for C:\Program Files is 1002, or whatever. So looping from 1 up to `stream' just doesn't make sense.
Now, a single period, ".", means current directory. Two periods, "..", means parent directory.
So, how might we go about writing this program? The truth is that writing this with loop constructs is very difficult. I don't even know how to begin. Fortunately, there's a much easier way to do it. It involves recursion. The idea is that you write a function that computes the directory tree for a single folder. If you find a folder within the folder you're traversing, then call your function on that. Don't worry, eventually the program will trace back to the original folder, assuming you don't have folders that have an infinite number of entries or a folder within a folder within a folder ... forever.
This is actually a classic example of using recursion. It's a very good exercise for you. It shouldn't be too hard, assuming you understand the basic idea of recursion (read a bit of the recursion tutorial) and can work with subroutines (functions/procedures) well. For this reason, I leave the code to you. I will provide, as an example, my tree program, written in Ruby. It recursively lists the entries in a directory and does some ASCII art to show the structure. |
|
|
|
|
 |
Prince Pwn
|
Posted: Sat Nov 17, 2007 10:33 pm Post subject: Re: Directory Scanning |
|
|
I know about functions and recursion, but I need to be able to get access to a folder within a folder, and I can't seem to find a way to tell if a folder exists within a folder. eg:
I can see all the music files within "My Music" but I don't know how to see each of the folders I have within "My Music". |
|
|
|
|
 |
Mazer

|
Posted: Sat Nov 17, 2007 11:29 pm Post subject: RE:Directory Scanning |
|
|
You have to concatenate. "My Music" isn't a directory, but "C:/Documents and Settings/username/My Documents/My Music" (or whatever) is.
What exactly was your error? the "." and ".." isn't a Turing thing, that's pretty standard operating system shit. "." refers to the current directory, and ".." is the parent directory. |
|
|
|
|
 |
Prince Pwn
|
Posted: Thu Nov 29, 2007 11:57 am Post subject: Re: Directory Scanning |
|
|
OK, this is what I have so far:
Turing: |
var defaultFont : int := Font.New ("Times:12")
var musicDirectory : string := "C:/Documents and Settings/" + Sys.GetUserName + "/My Documents/My Music/"
var mouseX, mouseY, button : int
if Dir.Exists (musicDirectory ) then
%put "Found Music Folder"
else
put Error.LastMsg
end if
var stream : int := Dir.Open (musicDirectory )
var counter : int := 0
var fileName : string
View.Set ("text")
for i : 0 .. stream
fileName := Dir.Get (stream )
exit when fileName = ""
if Dir.Exists (fileName ) then
%Dir.Open (fileName)
end if
if fileName (length (fileName )) = '3' then
put fileName
end if
end for
Input.Pause
quit
var winID : int := Window.Open ("graphics;offscreenonly,position:300,300")
loop
Mouse.Where (mouseX, mouseY, button )
Draw.FillBox (0, 0, maxx, maxy, black)
Font.Draw ("Current Directory: ", 20, maxy - 30, defaultFont, white)
Font.Draw (musicDirectory, 135, maxy - 30, defaultFont, yellow)
View.Update
cls
exit when hasch
end loop
Window.Close (defWinID )
|
It lists all the music in the My Music folder. I have several sub folders within My Music, about 50. So how would I go about finding these 50 sub folders? |
|
|
|
|
 |
Mazer

|
Posted: Thu Nov 29, 2007 12:14 pm Post subject: RE:Directory Scanning |
|
|
Recursion. You have a function that goes through the contents of a directory and printing out the filename of everything that ends with a '3' (I'm sure it'll work, but this isn't a great way to find MP3s). What happens when you find a directory? Just call that function you have that goes through directories and... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Prince Pwn
|
Posted: Fri Nov 30, 2007 9:05 pm Post subject: RE:Directory Scanning |
|
|
The thing is, the program cannot see a directory. The scanning I do can see . which is current, and .. which is back a directory I believe. I cannot see folders within a folder whatsoever. Maybe it's impossible to "scan" for a folder in Turing, or there's a way that I have not discovered yet.
PS I know the program finds anything that ends with a '3', I can easily change that to finding specifically a . then 'm', 'p', '3', but that isn't as important as finding a folder. |
|
|
|
|
 |
neufelni
|
Posted: Fri Nov 30, 2007 9:33 pm Post subject: Re: Directory Scanning |
|
|
I agree with everyone else. Definitely easiest with recursion. For my grade 12 final CompSci project I made a simple media player, which scanned the My Music folder and found all the mp3 files. |
|
|
|
|
 |
Mazer

|
Posted: Fri Nov 30, 2007 10:43 pm Post subject: RE:Directory Scanning |
|
|
It's not impossible, I've done the very same thing years ago. When you come across "." and ".." just skip them. If you can't seem to find a folder then write a program to list all contents of a single directory and try running that on something you know for sure to have subdirectories.
EDIT: Hey remember that time when I said
Quote: You have to concatenate. "My Music" isn't a directory, but "C:/Documents and Settings/username/My Documents/My Music" (or whatever) is. ?
Seriously. |
|
|
|
|
 |
Nick

|
Posted: Fri Nov 30, 2007 10:48 pm Post subject: RE:Directory Scanning |
|
|
this bit of code was taken directly from turing help
Turing: | % The "DirectoryListing" program.
setscreen ("text")
var streamNumber : int
var fileName : string
streamNumber := Dir.Open (".")
assert streamNumber > 0
loop
fileName := Dir.Get (streamNumber )
exit when fileName = ""
put fileName
end loop
Dir.Close (streamNumber ) |
|
|
|
|
|
 |
Prince Pwn
|
Posted: Fri Nov 30, 2007 11:41 pm Post subject: RE:Directory Scanning |
|
|
Thanks momp! Using that I can see the folders within a folder. I'll report back if I have any troubles. |
|
|
|
|
 |
|
|