Randomizing Array Values
Author |
Message |
Fozmik
|
Posted: Mon Jan 29, 2007 5:26 pm Post subject: Randomizing Array Values |
|
|
Basically, what I want to do is jumble the string values of one array into another array. This is easy enough, but I can't figure out a way to make the randomized array not contain duplicate values.
Here are the two arrays, the one containing the original values called "casearray" and the other which i want the strings to be randomized into called "randomcasearray". Also a for loop with a randint that randomly puts values into the other array 26 times.
How can I write this so that the program checks if each value has already been randomly entered into the second array, and does not add it into the array a second time?
code: |
var random : int
var randomcasearray : array 1 .. 26 of string
var casearray : array 1 .. 26 of string
casearray (1) := "0.01"
casearray (2) := "1"
casearray (3) := "5"
casearray (4) := "10"
casearray (5) := "25"
casearray (6) := "50"
casearray (7) := "75"
casearray (8) := "100"
casearray (9) := "200"
casearray (10) := "300"
casearray (11) := "400"
casearray (12) := "500"
casearray (13) := "750"
casearray (14) := "1000"
casearray (15) := "5000"
casearray (16) := "10000"
casearray (17) := "25000"
casearray (18) := "50000"
casearray (19) := "75000"
casearray (20) := "100000"
casearray (21) := "200000"
casearray (22) := "300000"
casearray (23) := "400000"
casearray (24) := "500000"
casearray (25) := "750000"
casearray (26) := "1000000"
for randomcase : 1 .. 26
randint (random, 1, 26)
randomcasearray (randomcase) := casearray (random)
end for
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
agnivohneb

|
Posted: Mon Jan 29, 2007 5:52 pm Post subject: Re: Randomizing Array Values |
|
|
You could make a boolean array of 1 to 26 (ill call it done in this example) predefine them all as false first.
This will be your array to tell if that number has been used already.
have a loop and in it get your random number.
if the done (random number) = false then do your switch program if not then continue the loop and get a new number.
if it dose do the program after make sure you turn done (randomcase) to true or you will have a problem when you exit.
to exit the loop after the if statement just call exit when done (randomcase) = true
this way may be slightly slow on a bad day because it can draw the same number a bunch of times. |
|
|
|
|
 |
Bored

|
Posted: Mon Jan 29, 2007 6:41 pm Post subject: Re: Randomizing Array Values |
|
|
agnivohneb wrote:
You could make a boolean array of 1 to 26 (ill call it done in this example) predefine them all as false first.
This will be your array to tell if that number has been used already.
have a loop and in it get your random number.
if the done (random number) = false then do your switch program if not then continue the loop and get a new number.
if it dose do the program after make sure you turn done (randomcase) to true or you will have a problem when you exit.
to exit the loop after the if statement just call exit when done (randomcase) = true
this way may be slightly slow on a bad day because it can draw the same number a bunch of times.
I would not recomend this method as though it would almost surely end, though there is the possibility of it never finishing. Though this would would not reasonably happen, this will make it unpredictable, and every now and then it will take a while, especally with larger arrays.
The more reasonable approach would be to copy the array completely, then shuffle the new array. Shuffling requires a for loop, a temporary variable of the same type (i.e. string), and two random numbers. You would then run the for loop, within it you would choose two random elements of the array, make temp the first, then make the first the second, then make the second the first. In suido code it would look like this:
code: | var list1, list2 : array 1 to whatever of string
assign values to list1
copy to list2
var rand1, rand2 : int
var temp : string
for 1 to a reasonable amount (probably 10-20 times size of array)
assign random values to rand1 and rand2
temp becomes list2 (rand1)
list2 (rand1) becomes list2 (rand2)
list2 (rand2) becomes temp
end for |
I'll leave you to fill in the blanks. |
|
|
|
|
 |
Fozmik
|
Posted: Mon Jan 29, 2007 7:06 pm Post subject: Re: Randomizing Array Values |
|
|
Thanks to both of you for your help. Bored, that works perfectly. |
|
|
|
|
 |
Tony
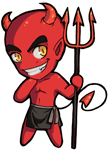
|
|
|
|
 |
jrblast
|
Posted: Thu Feb 08, 2007 8:05 pm Post subject: RE:Randomizing Array Values |
|
|
You could also have 2 arrays (this time flexible, both of em.), that one fully declared, and another that starts as 1 .. 0. They would be equal length and you would do something like this code: | var randomNum : int
for i : 1 .. upper (caseArray) %run as many times as there are cases
randint (randomNum, 1, upper (caseArray)) %pick one of the remaining cases
new otherCaseArray, upper (otherCaseArray) + 1 %create a spot for it then mov it into the other array (at its highest spot)
otherCaseArray (upper (otherCaseArray := caseArray (randomNum)
for j : randomNum .. upper (caseArray) - 1 %remove the section of the array that has now been moved into the other one
caseArray (j) := caseArray (i + 1)
end for
new caseArray, upper (caseArray) - 1
end for
|
hope you can understand that. |
|
|
|
|
 |
|
|