Problem with turing or my computer?
Author |
Message |
Piro24
|
Posted: Mon Oct 23, 2006 4:13 pm Post subject: Problem with turing or my computer? |
|
|
Why is that my program has a very hard time drawing boxes, but not ovals...
Ex - Here is my code for following an oval. It runs well.
code: | setscreen ("offscreenonly")
var x, y, b : int
loop
Mouse.Where (x, y, b)
Draw.FillOval (x, y, 10, 10, black)
View.Update
cls
end loop
|
Here is the same code, but with a box...
code: |
setscreen ("offscreenonly")
var x, y, b : int
loop
Mouse.Where (x, y, b)
Draw.FillBox (x, y, 10, 10, black)
View.Update
cls
end loop
|
Now here is the program I want to use, but for some reason, it runs slow - horribly!
code: | setscreen ("offscreenonly")
var x, y, b : int
var xB : int := maxx div 2
var yB : int := maxy div 2
loop
Mouse.Where (x, y, b)
Draw.FillBox (xB - 27, yB - 27, xB + 27, yB + 27, green)
if b = 1 and x > xB - 27 and x < xB + 27 and y > yB - 27 and y < yB + 27 then
xB := x
yB := y
end if
View.Update
cls
end loop |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Clayton

|
Posted: Mon Oct 23, 2006 4:44 pm Post subject: (No subject) |
|
|
its the View.Update + setscreen ("offscreenonly") combo. Because View.Update initiates the use of a second "offscreen" buffer, it eats memory whenever View.Update is called to draw the offscreen buffer to the monitor.
for the full explanation check out my View.Set and View.Update tutorial in the Turing Walkthrough |
|
|
|
|
 |
Piro24
|
Posted: Mon Oct 23, 2006 5:19 pm Post subject: (No subject) |
|
|
Freakman, I understand what you are trying to say, but here is an example that eliminates the problem of View.Update and setscreen...
In this code, the circle moves around nicely on my computer, with great flow.
code: | setscreen ("offscreenonly")
var x, y, b : int
loop
Mouse.Where (x, y, b)
Draw.FillOval (x, y, 25, 25, black)
View.Update
cls
end loop |
Than, I use the same code to make a box move like that...The results are a very glitchy box moving around the screen at a terrible rate...
code: | setscreen ("offscreenonly")
var x, y, b : int
loop
Mouse.Where (x, y, b)
Draw.FillBox (x, y, x - 30, y - 30, black)
View.Update
cls
end loop |
So than I thought it might even be the addition of the subtraction in the parameters of the box...So I input that in with the circle - but still, the circle runs smoothly...
code: | setscreen ("offscreenonly")
var x, y, b : int
loop
Mouse.Where (x, y, b)
Draw.FillOval (x, y, x - 10, x - 10, black)
View.Update
cls
end loop |
So in conclusion, I don't understand how the setscreen/View.Update could be the problem, seeing as it runs fine with an oval, but not a box. Also, it's obviously the the addition of extra operations in the parameters of the box...So that is where I need help. |
|
|
|
|
 |
ericfourfour
|
Posted: Mon Oct 23, 2006 5:45 pm Post subject: (No subject) |
|
|
simply throw a delay in.
Eg. delay (10) - delays the program for 10 miliseconds.
There are 1000 miliseconds in a second. |
|
|
|
|
 |
Ultrahex
|
Posted: Mon Oct 23, 2006 5:46 pm Post subject: (No subject) |
|
|
This Is Turing, Ummm, im curious now if this is how other graphical stuff works... hmmm oh well |
|
|
|
|
 |
Piro24
|
Posted: Mon Oct 23, 2006 5:56 pm Post subject: (No subject) |
|
|
ericfourfour wrote: simply throw a delay in.
Eg. delay (10) - delays the program for 10 miliseconds.
There are 1000 miliseconds in a second.
Hmm...Works perfect with a 1 millisecond delay...
Thanks
You think you could explain to me why, just so I understand...I like to understand things, not just know them  |
|
|
|
|
 |
ZeroPaladn
|
Posted: Wed Oct 25, 2006 12:58 pm Post subject: (No subject) |
|
|
All of yoru code runs fine on my computer, and this is a school computer!
As for the delay, it is completely useless in this program. Delay simply pauses the execution of the code inside the program for said milleseconds. |
|
|
|
|
 |
Ultrahex
|
Posted: Wed Oct 25, 2006 3:32 pm Post subject: (No subject) |
|
|
Zero Padlin, Hes Needs The Delay for some odd reason, we have not figured it out yet!, maybe a bug in turing or something. We really are not going to bother looking at it, and yes ive tried on 3 PCs now so :/ |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
iamcow
|
Posted: Wed Oct 25, 2006 4:36 pm Post subject: (No subject) |
|
|
everybody talks about how offscreenonly slows you down, but i've never noticed it, not even with my old 667 mhz comp |
|
|
|
|
 |
ericfourfour
|
Posted: Wed Oct 25, 2006 5:32 pm Post subject: (No subject) |
|
|
Putting the delay in has something to do with keeping the cpu consistently working or else your program will bottleneck and lag (I'm no expert). |
|
|
|
|
 |
[Gandalf]

|
Posted: Wed Oct 25, 2006 6:45 pm Post subject: (No subject) |
|
|
Eh? You put the delay in so that your computer doesn't keep working when it doesn't have to, redrawing things at a pace faster than it matters. It's the same concept as limiting frames per second in a game, after a certain point it doesn't make a visual difference.
And if you don't think offscreenonly with View.Update slows down your program try this:
code: | var timeA, timeB : int
setscreen ("offscreenonly")
for i : 1 .. 5000
Draw.FillOval (Rand.Int (1, maxx), Rand.Int (1, maxy), 10, 10, Rand.Int (1, 15))
View.Update
end for
timeA := Time.Elapsed
cls
setscreen ("nooffscreenonly")
for i : 1 .. 5000
Draw.FillOval (Rand.Int (1, maxx), Rand.Int (1, maxy), 10, 10, Rand.Int (1, 15))
end for
timeB := Time.Elapsed - timeA
cls
put "With offscreenonly: ", timeA, " milliseconds"
put "Without offscreenonly: ", timeB, " milliseconds" |
It takes about twice as long with offscreenonly. |
|
|
|
|
 |
Piro24
|
Posted: Wed Oct 25, 2006 7:02 pm Post subject: (No subject) |
|
|
[Gandalf] wrote: Eh? You put the delay in so that your computer doesn't keep working when it doesn't have to, redrawing things at a pace faster than it matters. It's the same concept as limiting frames per second in a game, after a certain point it doesn't make a visual difference.
And if you don't think offscreenonly with View.Update slows down your program try this:
code: | var timeA, timeB : int
setscreen ("offscreenonly")
for i : 1 .. 5000
Draw.FillOval (Rand.Int (1, maxx), Rand.Int (1, maxy), 10, 10, Rand.Int (1, 15))
View.Update
end for
timeA := Time.Elapsed
cls
setscreen ("nooffscreenonly")
for i : 1 .. 5000
Draw.FillOval (Rand.Int (1, maxx), Rand.Int (1, maxy), 10, 10, Rand.Int (1, 15))
end for
timeB := Time.Elapsed - timeA
cls
put "With offscreenonly: ", timeA, " milliseconds"
put "Without offscreenonly: ", timeB, " milliseconds" |
It takes about twice as long with offscreenonly.
I wasn't doubting offscreenonly didn't slow down your computer, I was saying that in that particular instance it wouldn't make difference...Unless it has preferences towards circles/boxes. |
|
|
|
|
 |
[Gandalf]

|
Posted: Wed Oct 25, 2006 10:03 pm Post subject: (No subject) |
|
|
Piro24, that was in response to this post:
iamcow wrote: everybody talks about how offscreenonly slows you down, but i've never noticed it, not even with my old 667 mhz comp
Not yours.  |
|
|
|
|
 |
Dan
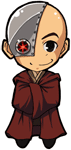
|
Posted: Thu Oct 26, 2006 1:02 pm Post subject: (No subject) |
|
|
The reason why a dealy of any amount helps is becues with out a dealy the process runing the program has full or magor control/prority in the cpu. This means that it will be using the cpu as much as it can and not leting other process use it (at least the % of the cpu the process was given, probly about 50%). Once you add a dealy it gives up the cpu to other process on the computer allowing things like windows backgorund tasks (like making the mouse work) regain cpu time or at least more cpu time.
In reality the verson with out the dealy is runing faster but it is taking cpu cycels away from things in the windows API like updating the screen, moving the mouse, ect. When you add the dealy it gives up some cycels so the windows API and other background process can keep up and things seem to run smoother.
At least this is my theroy, and i know other langues work like this but with out more knowalge of how dealy realy works deep down in turing it is hard to say for shure.
P.S. on hyper thread, duel core or newer cpus this will not be as much as an issue as the other part of the cpu is doing the background work. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
|
|