random number HELP
Author |
Message |
milz
|
Posted: Thu May 18, 2006 3:51 pm Post subject: random number HELP |
|
|
hey. how do u randomize a series of numbers without repeating lets say for example i need numbers 1 to 10 and i want 10 outputs but i dont 'want any of the numbers to repeat
plz post anything that cud help plz aite thankx[/code] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
TheOneTrueGod

|
Posted: Thu May 18, 2006 4:09 pm Post subject: (No subject) |
|
|
Thats the inefficient way. Theres a MUCH better way, a something like this:
code: |
var ar : array 1..10 of int
var temp,r : int
for i : 1..upper(ar)
ar (i) := i
end for
for i : 1..upper(ar)
r := Rand.Int(1,10)
temp := ar(r)
ar(r) := ar(i)
ar(i) := temp
end for
|
This way, if you have 1000 elements, you won't get hung up on the last one for an indefinite time.
NOTE: DO NOT USE CODE THAT YOU DO NOT UNDERSTAND. YOU ***WILL*** GET SCREWED OVER LATER. If you can't understand what the code does, post and get help. |
|
|
|
|
 |
XeroX
|
Posted: Thu May 18, 2006 4:15 pm Post subject: (No subject) |
|
|
ya, sorry about that |
|
|
|
|
 |
Tony
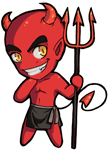
|
Posted: Thu May 18, 2006 4:15 pm Post subject: (No subject) |
|
|
hah, that's an interesting way of looking at the problem. Depending on the application, this might be better  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TheOneTrueGod

|
Posted: Thu May 18, 2006 4:19 pm Post subject: (No subject) |
|
|
Whats the difference between the two? Aside from one going from top to bottom, as opposed to bottom to top, and one outputting the numbers mid loop? |
|
|
|
|
 |
Cervantes

|
Posted: Thu May 18, 2006 4:36 pm Post subject: (No subject) |
|
|
The problem with yours, TheOneTrueGod, is that it won't always output a nice random list. The randomness of the list is a function of the number of times you swap elements and the luck of the draw, when it comes to selecting which elements to swap.
For example, given your array from 1 to 10, you could go through 20 iterations of swapping elements, but it is entirely possible that one each of those 20 iterations you select to swap elements 1 and 2. The result: a perfectly ordered list. |
|
|
|
|
 |
TheOneTrueGod

|
Posted: Thu May 18, 2006 5:16 pm Post subject: (No subject) |
|
|
Ah, I see, makes sense.
Though in effect, that perfectly ordered list should still be a possibility with randomness. If you shuffle a deck of cards, the laws of probability state that eventually (given enough time) that deck will return to its original state (You probably won't be around to enjoy it though ).
Anyways, thanks for info |
|
|
|
|
 |
Cervantes

|
Posted: Fri May 19, 2006 5:31 pm Post subject: (No subject) |
|
|
TheOneTrueGod wrote:
Though in effect, that perfectly ordered list should still be a possibility with randomness. If you shuffle a deck of cards, the laws of probability state that eventually (given enough time) that deck will return to its original state (You probably won't be around to enjoy it though  ).
Good point. Though that's possible with Tony's solution as well. With yours, it's also possible, but more common, I should think. Too common.
Tony's solution really mimicks how it is really done: Drawing a random number from a set of diminishing numbers. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
zylum

|
Posted: Fri May 19, 2006 11:33 pm Post subject: (No subject) |
|
|
i have to disagree. if you run some tests, you will see that in both cases the odds of a number being placed in its original position are equal at about 10%. the disadvantage with Tonys solution is that it doesnt reorder the array. it just outputs the sequence of numbers. the result array has many repeats. also, if you want to repeat the process multiple times, you have to reinitialize the array where as in TheOneTrueGods way you dont need to. |
|
|
|
|
 |
|
|