translating...
Author |
Message |
JayLo
|
Posted: Wed Jan 29, 2003 2:26 pm Post subject: translating... |
|
|
I need help with a translator that follows some type of letter code such as
A = Z, B = Y, C = X... etc. Is there any way to do that? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
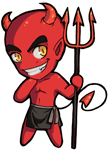
|
Posted: Wed Jan 29, 2003 3:04 pm Post subject: (No subject) |
|
|
umm... well if you got the key for what letters to change to what... then you can do it...
instead of writing a bunch of IF statments though, you can setup an array with range corresponding to ASCII value of the letter. This way you can decode the message with a single line inside your loop.
code: |
for i:1..length(text)
put CodeArray(ord(text(i)))
end for
|
you just have to fill in your CodeArray with letters to use... such as CodeArray(65) would = Z and CodeArray(66) = Y, etc.
65 stands for A and 66 for B, etc |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DarkHelmet
|
Posted: Thu Jan 30, 2003 2:38 pm Post subject: (No subject) |
|
|
i don't know if this will help you.
procedure code(var strword : string)
var intlength: int
var strletter : string
var strtemp : string
var intnum : int
intlength := length(strword)
strtemp := ""
for i : 1..intlength
intnum := ord(strword(i..i))
if strword(i..i) not= " " then
intnum := 90 - (intnum - 65)
end if
strletter := chr(intnum)
strtemp := strtemp + strletter
end for
strword := strtemp
end code
note that this only works if all the letters are capital, and if there are no odd characters. if you want lower case switch the 90 with 122 and the 65 with 97. |
|
|
|
|
 |
JayLo
|
Posted: Thu Jan 30, 2003 6:15 pm Post subject: undercase?? |
|
|
Encode Procedure
procedure encodeText
encoded := ""
encode := GUI.GetText (encoderTextField)
lengthEncode := length (encode)
for i : 1 .. lengthEncode
encoding := intstr (ord (encode (i)))
encoded := encoded + encoding
end for
GUI.SetText (encoderTextField, "")
GUI.SetSelection (decoderTextField, 0, 0)
GUI.SetActive (decoderTextField)
GUI.SetText (decoderTextField, encoded)
end encodeText
Decode Procedure
procedure decodeText
decoded := ""
decodeWord := GUI.GetText (decoderTextField)
lengthDecode := length (decodeWord)
decode := GUI.GetText (decoderTextField)
for i : 1 .. lengthDecode by 2
decoding := chr (strint (decode (i .. i + 1)))
decoded := decoded + decoding
end for
GUI.SetText (encoderTextField, "")
GUI.SetText (decoderTextField, "")
GUI.SetSelection (encoderTextField, 0, 0)
GUI.SetActive (encoderTextField)
GUI.SetText (encoderTextField, decoded)
end decodeText
My current problem is getting the decodeText procedure to decode the 3 digit ASCII undercase letters... argh... |
|
|
|
|
 |
JayLo
|
Posted: Fri Jan 31, 2003 12:25 pm Post subject: entire program |
|
|
import GUI
var passWin : int := Window.Open ("graphics:200;100,nocursor,nobuttonbar,position:center;middle,popup,title:Password")
var passTextField : int
var passLabel : int
var pass : string
var goodpass : string := "dump"
var passwordFont : int := Font.New ("Heffaklump:10")
var verify : boolean := false
GUI.SetBackgroundColour (42)
procedure checkPassword
pass := GUI.GetText (passTextField)
if pass = goodpass then
verify := true
end if
end checkPassword
procedure passwordEntered (text : string)
GUI.SetSelection (passTextField, 0, 0)
GUI.SetActive (passTextField)
checkPassword
end passwordEntered
passLabel := GUI.CreateLabelFull (0, 0, "eNter pAsSwoRd...", maxx, maxy, GUI.TOP + GUI.CENTER, passwordFont)
passTextField := GUI.CreateTextFieldFull (50, maxy div 2, 100, "", passwordEntered, GUI.INDENT, passwordFont, 0)
loop
exit when GUI.ProcessEvent or verify = true
end loop
GUI.CloseWindow (passWin)
var encoderLabel : int
var encoderTextField : int
var encodeButton : int
var encode : string
var lengthEncode : int
var encoding : string
var encoded : string := ""
var decoderLabel : int
var decoderTextField : int
var decodeButton : int
var decode : string
var lengthDecode : int
var decoding : string
var decoded : string := ""
var decodeWord : string
var fontID : int := Font.New ("WillyWonka:12")
var codeWord : int := Font.New ("verdana:10")
var titleFont : int := Font.New ("Chinese Takeaway:20")
var copyright : int := Font.New ("Gemelli:10")
var quitButton : int
var stremout : int
procedure encodeTextEntered (text : string)
GUI.SetSelection (encoderTextField, 0, 0)
GUI.SetActive (encoderTextField)
end encodeTextEntered
procedure decodeTextEntered (text : string)
GUI.SetSelection (decoderTextField, 0, 0)
GUI.SetActive (decoderTextField)
end decodeTextEntered
procedure encodeText
encoded := ""
encode := GUI.GetText (encoderTextField)
lengthEncode := length (encode)
for i : 1 .. lengthEncode
encoding := intstr (ord (encode (i)))
encoded := encoded + encoding
end for
GUI.SetText (encoderTextField, "")
GUI.SetSelection (decoderTextField, 0, 0)
GUI.SetActive (decoderTextField)
GUI.SetText (decoderTextField, encoded)
open : stremout, "encoded for posting.txt", put
put : stremout, encoded
close : stremout
end encodeText
procedure decodeText
decoded := ""
decodeWord := GUI.GetText (decoderTextField)
lengthDecode := length (decodeWord)
decode := GUI.GetText (decoderTextField)
for i : 1 .. lengthDecode by 2
decoding := chr (strint (decode (i .. i + 1)))
decoded := decoded + decoding
end for
GUI.SetText (encoderTextField, "")
GUI.SetText (decoderTextField, "")
GUI.SetSelection (encoderTextField, 0, 0)
GUI.SetActive (encoderTextField)
GUI.SetText (encoderTextField, decoded)
end decodeText
var window : int := Window.Open ("graphics:200;300,nocursor,nobuttonbar,position:center;middle,popup,title:Translator Beta")
GUI.SetBackgroundColor (42)
Font.Draw ("translate.", 30, maxy - 20, titleFont, black)
Font.Draw ("operation d.u.m.p.", maxx - 110, 10, copyright, black)
Font.Draw ("caps, numbers, and punctuation.", maxx - 200, 20, copyright, black)
encoderLabel := GUI.CreateLabelFull (5, 250, "EnCoder", 0, 0, 0, fontID)
encoderTextField := GUI.CreateTextFieldFull (70, 250, 100, "", encodeTextEntered, GUI.INDENT, codeWord, 0)
encodeButton := GUI.CreateButton (70, 200, 0, "EnCodE", encodeText)
decoderLabel := GUI.CreateLabelFull (5, 100, "dEcoDer", 0, 0, 0, fontID)
decoderTextField := GUI.CreateTextFieldFull (70, 100, 100, "", decodeTextEntered, GUI.INDENT, codeWord, 0)
decodeButton := GUI.CreateButton (70, 150, 0, "dEcoDe", decodeText)
quitButton := GUI.CreateButton (70, 50, 0, "Quit", GUI.Quit)
loop
exit when GUI.ProcessEvent
end loop
Window.Close (window) |
|
|
|
|
 |
Tony
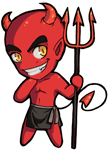
|
Posted: Fri Jan 31, 2003 3:49 pm Post subject: (No subject) |
|
|
nice program... I like how it looks
too bad it works only for caps... you can fix that by checking the first digit of the next letter you want to decode... if it starts with a 1, then you read next 3 digits for the letter this should cover characters 20-199 which includes all of the alphanumeric characters
also you need to have a pattern mask of sords. What I mean is that you need to apply a math function to each ASCII value so it would be harder to recognize the pattern. ASCII values just stand out too much. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
JayLo
|
Posted: Sat Feb 01, 2003 9:02 am Post subject: thanks. |
|
|
thanks for the encouragement... about sords, would it be like an if statement for each value? |
|
|
|
|
 |
JayLo
|
Posted: Wed Feb 12, 2003 12:22 pm Post subject: hello?? |
|
|
need help... please please reply. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
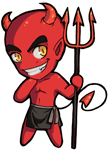
|
Posted: Wed Feb 12, 2003 12:32 pm Post subject: (No subject) |
|
|
what I mean is that you need to apply some math function to the ASCII value to hide it. Such as if the value is 500, noone can guess which letter it is since its out of range, and even if they are inside the range (you need that if you can to post coded message in character format) it will not make sence.
such as
code: |
getch(c)
put ord(c) +1
|
that +1 will shift ASCII value up 1 and if you output CHR() of that number, it will be next character alphabetically (so A+1 is B)
the more complex the function, the harder it is to guess the original ASCII value.
(ord(character)*2 +5)/3
and to decode it, you use reverse:
chr((number*3 - 5)/2) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|