An Open Challenge
Author |
Message |
chrispminis
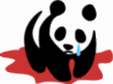
|
Posted: Wed Mar 01, 2006 6:32 pm Post subject: (No subject) |
|
|
Aha. I recognize right away that the first one, is very much like Turing. And while I can sort of see how the second one works, the first one is much more familiar to me. So I guess it makes more sense. That's probably the point you ewre trying to make? Wanna explain the second one? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Wed Mar 01, 2006 8:06 pm Post subject: (No subject) |
|
|
Well, we can simplify a few things.
code: | puts "Hello #{first_name1} #{last_name1} and #{first_name2} #{last_name2}!" |
Is equivalent to:
code: | Printf.printf "Hello %s %s and %s %s!\n" first_name1 last_name1
first_name2 last_name2 |
Thus we can simplify the two programs to:
code: | def greet(names)
if names.length == 0
elsif names.length == 1
first_name = names[0][0]
last_name = names[0][1]
elsif names.length == 2
first_name1 = names[0][0]
last_name1 = names[0][1]
first_name2 = names[1][0]
last_name2 = names[1][1]
else
end
end |
And:
code: | let greet =
function
| [] ->
| [(first_name, last_name)] ->
| [(first_name1, last_name1); (first_name2, last_name2)] ->
| _ -> |
Now, if the names array/list is empty, it's length will be zero. In the first of the two examples, we test for that.
If there is only one name, the length will be one. We can then use:
To access that name. Then we can further subscript that to get the individual parts of the name which we give suitable names.
If there are two names, well then we can do the same thing, basically.
If there are more than two elements, we have a catch-all clause.
Now... let's look at the O'Caml code. There are two notable things going on here.
- I don't ever have "names" anywhere.
- I don't have any "something = ..." variable assignments.
We have to think of functions as a way of mapping one value onto another (or an action). The "greet" function maps an empty list onto an action. The syntax allows us to express this directly, with no need to worry abount testing for the length of a particular list.
Next we say that a list with a single tuple of two names maps to a different action. The pattern mathching automatically gives names to the individualk parts of that name.
A list with two tuples of names maps to a different action, and the match anything pattern (underscore) provides a default. |
|
|
|
|
 |
chrispminis
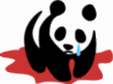
|
Posted: Wed Mar 01, 2006 11:46 pm Post subject: (No subject) |
|
|
Holy Crap! I actually understand what you saying. I think. I just looked back, and I understand now sort of how the second program works. In fact, I think I might understand the second one better but only because...
What do the numbers in the brackets do?
code: |
first_name1 = names[0][0]
last_name1 = names[0][1]
first_name2 = names[1][0]
last_name2 = names[1][1]
|
|
|
|
|
|
 |
wtd
|
Posted: Thu Mar 02, 2006 12:42 am Post subject: (No subject) |
|
|
Well, let's say the array you're passing to the greet method looks like:
code: | names = [["foo", "bar"], ["wooble", "ninja"]] |
That is, it's an array which contains two other arrays.
So:
Gets you:
Therefore:
Gets you "foo". |
|
|
|
|
 |
chrispminis
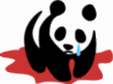
|
Posted: Thu Mar 02, 2006 8:51 pm Post subject: (No subject) |
|
|
Ah I see. What language was all that in anyways? And I know that there is a separate thread for this but... What programming language do you recommend I try, remembering that I AM a Turing Baby. And also, when should I switch? Are there certain concepts in Turing I should master in a familiar environment before starting a new language? |
|
|
|
|
 |
wtd
|
Posted: Thu Mar 02, 2006 9:00 pm Post subject: (No subject) |
|
|
The languages were Ruby and O'Caml.
Learn another language now. It is possible to hold multiple programming languages in your mind at once. I would mention, though, that I do not advocate "switching". You learn multiple programming languages so you can choose between them and always have the best tool for the job. |
|
|
|
|
 |
Cervantes

|
Posted: Fri Mar 03, 2006 6:19 pm Post subject: (No subject) |
|
|
chrispminis wrote: Ah I see. What language was all that in anyways? And I know that there is a separate thread for this but... What programming language do you recommend I try, remembering that I AM a Turing Baby. And also, when should I switch? Are there certain concepts in Turing I should master in a familiar environment before starting a new language?
If you're going to learn Ruby, learning the basics of OOP will be a great help, as it is 100% object oriented. Tutorial One, Two, and Three available.
If you go to O'Caml, you'll definately want to be familiar with functions, though that's probably not a problem. O'Caml supports OOP, so knowing it would be helpful. However, you're not restricted to coding in an OOP manner in O'Caml, so it's not entirely necessary.
Learning a new language can't hurt. If you try and don't think you can do it, go back to Turing, learn more, write more advanced programs, and try again. |
|
|
|
|
 |
|
|