Author |
Message |
ZeroPaladn
|
Posted: Thu Sep 29, 2005 1:07 pm Post subject: shortening code pops up prob... |
|
|
Hey, my decimal/binary/hex program is almost complete (damn math), and im try to shorten some code. this is the code that i made to convert decimal to binary, and you have to know how to do math between binary and decimal to see how this code works. regardless, its buggy, here it is.
code: |
var numin : int
var binout : array 1..8 of int
var num2binmath : int := 128
get numin
for i : 1..8
if numin < num2binmath then
binout (i) := 0
else
numin := numin - num2binmath
binout (i) := 1
num2binmath := num2binmath div 2
end if
end for
for o : 1..8
put binout (o)..
end for
|
thanks guys |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
codemage

|
Posted: Thu Sep 29, 2005 2:02 pm Post subject: (No subject) |
|
|
Your algorithm is fundamentally flawed.
Try again using this pseudo-code:
1. Let D= the number we wish to convert from decimal to binary.
2. Repeat until D=0:
a) If D is odd, put "1" in the leftmost open column, and subtract 1 from D.
b) else (D is even), put "0" in the leftmost open column.
c) Divide D by 2.
End Repeat
This is a slim algorithm, I did it in 15 lines - if you've got significantly more than that then you can still cut back. |
|
|
|
|
 |
ZeroPaladn
|
Posted: Thu Sep 29, 2005 8:52 pm Post subject: (No subject) |
|
|
thanks alot, the old code took up about 80 lines what youve just decribed in 15. Thats what i hate about my prgramming, i can do it, but its alot of code. |
|
|
|
|
 |
wtd
|
Posted: Thu Sep 29, 2005 8:55 pm Post subject: (No subject) |
|
|
Look for patterns. Places where you're doing repetitive work. Then let the computer do the repitition. |
|
|
|
|
 |
ZeroPaladn
|
Posted: Fri Sep 30, 2005 8:52 am Post subject: (No subject) |
|
|
HURRAY! fixed it! the area where it was doing the dividing, it wonly worked when it went thru that part of the for loop, so it didnt use that command when then number was smaller than was asked, so a bunch of 0's popped up. just move the calculation (num2binmath := num2binmath div 2), like so...
code: |
var numin : int
var binout : array 1 .. 8 of int
var num2binmath : int := 128
get numin
for i : 1 .. 8
if numin < num2binmath then
binout (i) := 0
else
numin := numin - num2binmath
binout (i) := 1
end if
num2binmath := num2binmath div 2
end for
for o : 1 .. 8
put binout (o) ..
end for
|
and it works. thanks wtd. |
|
|
|
|
 |
codemage

|
Posted: Fri Sep 30, 2005 12:03 pm Post subject: (No subject) |
|
|
Here's the algorithm I described, now that you've solved it. (For reference's sake).
code: | var dec : int
var bin : array 1 .. 8 of int
get dec
for decreasing counter : 8 .. 1
if dec mod 2 = 1 then
bin (counter) := 1
dec := dec - 1
else
bin (counter) := 0
end if
dec := dec div 2
end for
for counter : 1 .. 8
put bin (counter) ..
end for |
|
|
|
|
|
 |
[Gandalf]

|
Posted: Fri Sep 30, 2005 7:40 pm Post subject: (No subject) |
|
|
Ah, I posted this in the other thread by mistake... Here's what I got:
code: | var inputNum : int
var binaryOut : array 1 .. 8 of int := init (0, 0, 0, 0, 0, 0, 0, 0)
get inputNum
for count : 1 .. 8
if inputNum mod 2 = 1 then
binaryOut (count) := 1
else
binaryOut (count) := 0
end if
inputNum := inputNum div 2
end for
for decreasing count : 8 .. 1
put binaryOut (count) ..
end for |
|
|
|
|
|
 |
ZeroPaladn
|
Posted: Mon Oct 03, 2005 9:02 am Post subject: (No subject) |
|
|
aha... ive studied both of your subbimmisons and somethings bugging me... what the hell does mod do? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
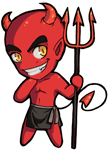
|
Posted: Mon Oct 03, 2005 10:23 am Post subject: (No subject) |
|
|
mod gives you a remainder of the division. |
|
|
|
|
 |
codemage

|
Posted: Mon Oct 03, 2005 11:57 am Post subject: (No subject) |
|
|
6 mod 2 = 0
7 mod 2 = 1
20 mod 5 = 0
23 mod 5 = 3
etc.
A very useful operator/function (depending on the language) in certain spots. |
|
|
|
|
 |
wtd
|
Posted: Mon Oct 03, 2005 12:46 pm Post subject: (No subject) |
|
|
Who needs loops?
code: | function dec2bin (number : int) : string
if number = 0 then
result "0"
else
result (dec2bin (number div 2) + strint (number mod 2))
end if
end dec2bin |
|
|
|
|
|
 |
ZeroPaladn
|
Posted: Mon Oct 03, 2005 12:56 pm Post subject: (No subject) |
|
|
so tahts what it does, ive read the turing help all it says is "modulo"... whatever that is. |
|
|
|
|
 |
|