Image Processing
Author |
Message |
Spartan_117

|
Posted: Thu Jul 28, 2005 9:07 am Post subject: Image Processing |
|
|
Im making a program that will analyze an image and distinguish between the sand, the water, and the air. To do this i am analyzing RGB (Red, Green, and Blue) values. Right now it takes a small picture of the sand (inputed by the user), and figures out the min and max RGB values creating a range of RGB values for the sand. It does the same for water and air. Then it scans the main image and draws a line at the border between sand and water, and water and air. If you take a look at the picture i've attached, you'll see exactly what type of picture im scanning.
Although this seems easy, the program is still far from perfect. the problem is that the RGB range for sand and water intersect (cross). SOme areas of water (due to the lighting possibly) hav the same RGB values as sand. This makes the program think that the water is sand, or the sand is water sometimes.
Basically i'd like some input on other ideas for scanning this image since reading the colour doesnt work perfectly.
thx
Description: |
|
Filesize: |
225.05 KB |
Viewed: |
2526 Time(s) |

|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Delos

|
Posted: Thu Jul 28, 2005 9:26 am Post subject: (No subject) |
|
|
Ok...what you've gotten yourself into here is what's called edge detection. It's pretty complex stuff it is. AFAIK, it involves some level of calculus to work correctly. I'll see if I can find anything useful for you - been wanting to make something like this for a while now.
|
|
|
|
|
 |
Spartan_117

|
Posted: Thu Jul 28, 2005 12:24 pm Post subject: (No subject) |
|
|
hmmm i guess it is pretty complicated, well thx for your interest, and ill reply with anything new aswell
thx Delos
|
|
|
|
|
 |
Delos

|
Posted: Thu Jul 28, 2005 12:57 pm Post subject: (No subject) |
|
|
If you just need to do that for the pics and don't need to actually make the proggie, you can do it in IrfanView.
|
|
|
|
|
 |
Spartan_117

|
Posted: Thu Jul 28, 2005 1:18 pm Post subject: (No subject) |
|
|
Well i actually need to make the program, but ill take a look into this program as well. (For motivation i guess )
THe Program works quite amazingly too, so fast.
|
|
|
|
|
 |
Spartan_117

|
Posted: Tue Aug 02, 2005 12:03 pm Post subject: (No subject) |
|
|
so Delos, get anywhere with the Edge DetectioN? I've tried and i've got nothing. Keeps detecting the wrong thing. But im only using colours to detect. WHat kind of calculus can u use to do this?
|
|
|
|
|
 |
Tony
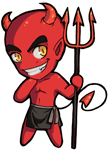
|
Posted: Tue Aug 02, 2005 2:17 pm Post subject: (No subject) |
|
|
Some points to be noted about edge detection:
An edge is a continues line along the perimeter of material. You have to view it as such, it's not connect the dots.
Try to work out the problem backwards. Take the solution, trace out the lines yourself and note the patterns. You should see that colour texture above the line is different from the one below the line. An important part to notice is that colours should be looked at in regions, not per pixel. If there's a grain of sand floating in the water, it should not neccessarly be considered a separate object.
Take an average colour value of various radii sizes, and compare that against another location. Try to figure out how rapidly it changes. A suddent change should point at an edge, but check the points nearby to see if that makes sense.
Calculus that Delos has mentioned comes in when you're analyzing rates of change between various parts: colour gradients, slopes of edge-lines, etc.
Oh yeah... and adjusting the values such as sample radius and allowable rate of change will set the tolerance and will generate a range of results from basic "sand/water/air" to more detailed "this patch of sand makes a shape".
While developing the program, I suggest starting out with a basic black & white picture for testing purposes, and then working your way up from there -- mixing in some grays are you go along.
|
|
|
|
|
 |
Spartan_117

|
Posted: Wed Aug 03, 2005 8:43 am Post subject: (No subject) |
|
|
Quote: Some points to be noted about edge detection:
An edge is a continues line along the perimeter of material. You have to view it as such, it's not connect the dots.
Try to work out the problem backwards. Take the solution, trace out the lines yourself and note the patterns. You should see that colour texture above the line is different from the one below the line. An important part to notice is that colours should be looked at in regions, not per pixel. If there's a grain of sand floating in the water, it should not neccessarly be considered a separate object.
Take an average colour value of various radii sizes, and compare that against another location. Try to figure out how rapidly it changes. A suddent change should point at an edge, but check the points nearby to see if that makes sense.
Calculus that Delos has mentioned comes in when you're analyzing rates of change between various parts: colour gradients, slopes of edge-lines, etc.
Oh yeah... and adjusting the values such as sample radius and allowable rate of change will set the tolerance and will generate a range of results from basic "sand/water/air" to more detailed "this patch of sand makes a shape".
While developing the program, I suggest starting out with a basic black & white picture for testing purposes, and then working your way up from there -- mixing in some grays are you go along.
thx, for the help, ill keep that in mind. hopefully all goes well. 8)
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Delos

|
Posted: Wed Aug 03, 2005 8:29 pm Post subject: (No subject) |
|
|
Ok, well this sorta works. It's pretty damn primitive, and it doesn't even go as far as being able to incorporate Tony's suggestions. However, it's something to start from, so take what ideas you can from it.
To run, just put it in a directory with some pics, and change 'picName' to whatever pic you want.
Also, you can use 'breakPoint' to change the senstivity. Try numbers that are between 1-2 for best results (1.15, as it's set to, works pretty well).
Turing: |
var picName : string := "pic.bmp"
var pic : int
var pW, pH : int
type pixel :
record
rC, gC, bC : real
end record
var picData : flexible array 1 .. 0, 1 .. 0 of pixel
pic := Pic.FileNew (picName )
pW := Pic.Width (pic )
pH := Pic.Height (pic )
setscreen ("graphics:" + intstr (pW ) + ";" + intstr (pH ) + ";nobuttonbar")
Pic.Draw (pic, 0, 0, picCopy)
new picData, pW, pH
for i : 1 .. pW
for j : 1 .. pH
RGB.GetColour (whatdotcolour (i, j ), picData (i, j ).rC, picData (i, j ).gC, picData (i, j ).bC )
end for
end for
const breakPoint : real := 1. 15
function _div (num1, num2 : real) : boolean
if num1 = 0| num2 = 0 then
result true
else
if max (num1, num2 ) / min (num1, num2 ) > breakPoint then
result true
else
result false
end if
end if
end _div
for i : 1 .. upper (picData )
for j : 1 .. upper (picData, 2) - 1
if _div (picData (i, j ).rC, picData (i, j + 1).rC )|
_div (picData (i, j ).bC, picData (i, j + 1).bC )|
_div (picData (i, j ).gC, picData (i, j + 1).gC ) then
picData (i, j ).rC := 1
picData (i, j ).gC := 1
picData (i, j ).bC := 1
else
picData (i, j ).rC := 0
picData (i, j ).gC := 0
picData (i, j ).bC := 0
end if
RGB.SetColour (1, picData (i, j ).rC, picData (i, j ).gC, picData (i, j ).bC )
drawdot (i, j, 1)
end for
end for
|
|
|
|
|
|
 |
zylum

|
Posted: Thu Aug 04, 2005 1:20 am Post subject: (No subject) |
|
|
ok, here's my attempt. dont get too excited, its really quite slow and not all that accurate... what it does is detect where the water is and then draws the line at the border between water and sand and water and air.
my algorithm first blurs the image, the it contrasts it to the max. then it removes any blobs that are smaller than the specified size. and finally it draws the boundaries. even with such a small image it takes about 40 seconds
heres a cropped version of the image i used. the full image will take way too long (a minute or two).
Description: |
|
Filesize: |
110.89 KB |
Viewed: |
2518 Time(s) |

|
Description: |
|
 Download |
Filename: |
image processing.t |
Filesize: |
4.57 KB |
Downloaded: |
140 Time(s) |
|
|
|
|
|
 |
Spartan_117

|
Posted: Thu Aug 04, 2005 8:05 am Post subject: (No subject) |
|
|
Well mine is used for this type of picture only. What mine does is it asks for a sample pic of the sand and water. Then it checks for the edge of the sand and the water. This way its more specific.
|
|
|
|
|
 |
|
|