Help With Game
Author |
Message |
Skeletor
|
Posted: Thu Jun 09, 2005 10:09 am Post subject: Help With Game |
|
|
Alright, so I've set out to make a Cannons type game where there is a player on each side shooting a ball at each other (like some very old DOS games some may remember). I took the projectile code from http://www.compsci.ca/v2/viewtopic.php?t=2406&highlight=cannons
and converted it from records to variables and encased it in a class. It works in that you can set a specified velocity and angle for the ball to fire, but for some reason you have to hold down the fire key to keep the ball from turning white (thus disappearing). On top of that, the ball seems to enter some decreasing arch if you just hold the fire key down. I've asked some people I know and they have no idea what is wrong and how to fix it.
code: |
class projectileMovement
import Input
export Projectile, Bounce, Movement, Controls
var startx : real := 0
var starty : real := 0
var x : real := 0
var y : real := 0
var angle : real := 0
var velocity : real := 0
var bounciness : real := 10
var weight : real := 10
var time1 : real := 0.0
procedure Projectile
x := (velocity * cosd (angle) * time1) + startx
y := (velocity * sind (angle) * time1 - (weight) * time1 ** 2 / 2) + starty
end Projectile
procedure Bounce
if y <= starty then
if (velocity) > 0 then
time1 := 0
startx := x
starty := y
velocity -= bounciness
end if
if (velocity) < 0 then
velocity := 0
end if
end if
end Bounce
procedure Movement
loop
time1 += .1 %The time and how fast it is moving
Projectile %applying movements to the projectile
Bounce %Detect ground and bounce back
drawfilloval (round (x), round (y), 5, 5, 12) %draw the projectile
View.Update
delay (10)
drawfilloval (round (x), round (y), 5, 5, white) %erase the projectile
exit
end loop
end Movement
procedure Controls
var key1 : array char of boolean
loop
Input.KeyDown (key1)
if key1 ('w') then
velocity += 1
if velocity > 90
then
velocity := 90
end if
put velocity
elsif key1 ('s') then
velocity -= 1
if velocity < 0
then
velocity := 0
end if
put velocity
elsif key1 ('a') then
angle += 1
if angle > 90
then
angle := 90
end if
put angle
elsif key1 ('d') then
angle -= 1
if angle < 0
then
angle := 0
end if
put angle
elsif key1 ('f') then
Projectile
Bounce
Movement
exit
end if
end loop
startx := 0
starty := 0
x := 0
y := 0
end Controls
end projectileMovement
var player : ^projectileMovement
new player
loop
player -> Controls
end loop
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
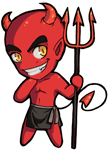
|
Posted: Thu Jun 09, 2005 6:03 pm Post subject: Re: Help With Game |
|
|
Skeletor wrote: I've asked some people I know and they have no idea what is wrong and how to fix it.
Well similarly to your situation, those some other people have no idea what's wrong because it's not their code ether. That what happens when you copy somebody else's program.
Turing: |
procedure Movement
loop
time1 + = . 1 %The time and how fast it is moving
Projectile %applying movements to the projectile
Bounce %Detect ground and bounce back
drawfilloval (round (x ), round (y ), 5, 5, 12) %draw the projectile
View.Update
delay (10)
drawfilloval (round (x ), round (y ), 5, 5, white) %erase the projectile
/*-->*/ exit /*omg, why is there an exit here?*/
end loop
end Movement
|
notice the 3rd last line |
|
|
|
|
 |
Skeletor
|
Posted: Fri Jun 10, 2005 9:45 am Post subject: (No subject) |
|
|
Because if there isn't an exit there the program only executes once. |
|
|
|
|
 |
StarGateSG-1

|
Posted: Fri Jun 10, 2005 10:57 am Post subject: (No subject) |
|
|
Not true, it will still work but you have to chnage it.
Also, I disapprove of you using other people's code, first because it isn;t yours, if you don't understand it how are you suppose to fix problems. It goes against programer ethic's. |
|
|
|
|
 |
Drakain Zeil
|
Posted: Fri Jun 10, 2005 3:42 pm Post subject: (No subject) |
|
|
Ethics is something that most of these kids today don't have. Everything is given to them, thinking is discouraged... I don't like it. |
|
|
|
|
 |
Skeletor
|
Posted: Fri Jun 10, 2005 7:50 pm Post subject: (No subject) |
|
|
Thanks for all the patronizing comments.
I'm not here for an ethics lesson (for the love of god, I am enrolled at York University for Philosophy)... 'Kids these days'? I'd like you to ponder the saying 'The more things change, the more they stay the same.'
Secondly I am not a programmer and, thus, thought building upon what someone has already come up with would be better than trying it from scratch. Besides, the poster of the original code never said it was copyrighted.
Now will someone please help me? Or will there just be ten more posts jumping on the good ole' putdown bandwagon? |
|
|
|
|
 |
Cervantes

|
Posted: Fri Jun 10, 2005 8:17 pm Post subject: (No subject) |
|
|
If you're not a programmer, why are you using classes? If you're in University, the math/physics here should not be difficult. Therefore, start the code from scratch. You'll learn more, and maybe come to appreciate programming. Most importantly, you'll have done it yourself, will understand it entirely, and be able to make modifications as you choose. I can pretty well guarentee you that if you start it from scratch, you'll be able to fix this problem.
Lastly, the fact that you are "enrolled at York University for Philosophy" is no excuse to steal code. I know what you are saying, don't worry; I've just twisted it a bit. You're really saying that you think your understand of ethics is better than ours, and that what you're doing is perfectly fine. Guess again! Generally, something like this would be okay, provided you are not planning to submit this program to anyone as your own work. However, in a case like this, even if you aren't going to submit it to anyone, it's not okay because you learn nothing (or, less, at the very least).
Cheers |
|
|
|
|
 |
Skeletor
|
Posted: Fri Jun 10, 2005 8:36 pm Post subject: (No subject) |
|
|
It looks like it is the bandwagon afterall.
As for university... I did say 'Philosophy' for a reason. And that reason is I haven't take math since Grade 11 and I've never taken physics. I wanted to do a cannons game but I had no clue how to do the math for it. So I found code that did the calculations and voila!
Any actual help? Or just more of the same? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
lyam_kaskade

|
Posted: Fri Jun 10, 2005 9:17 pm Post subject: (No subject) |
|
|
If you're in university, why muck about with Turing? The only reason most of us are here is because the high school curriculum forces us to. There's far better languages you could learn (with larger communities surrounding them as well).
As for your code, you could always pm Homer_Simpson, considering he's the one who wrote it.
However, lest you ignore me because I don't give you what you want, I've made a few corrections:
The reason you had to hold down f to fire was, as Tony said, there was an exit statement right there. If you knew anything about loops, you'd have realized that. Tutorial here: http://www.compsci.ca/v2/viewtopic.php?t=3678
I also put a locate command by the put statements, so it doesn't look quite so stupid. tutorial here: [url]http://www.compsci.ca/v2/viewtopic.php?t=7629 [/url]
Furthermore, an error Homer_Simpson made while coding, was that all objects move down at the same rate, (that is, acceleration due to gravity) regardless of weight. So i removed that variable altogether (I suppose he might have wanted to show projectiles on different planets, however). If you had a basic understanding of physics, you'd have seen that one as well.
If you're serious about learning Turing, I suggest the walkthrough here:
[url] http://www.compsci.ca/v2/viewtopic.php?t=8808[/url]
However, if you're really just into having people write code for you, I suggest you just download the game you're thinking of and play it. If it's the one I'm thinking of, you can find it here:
http://www.classicgaming.com/scorch/
code: |
class projectileMovement
import Input
export Projectile, Bounce, Movement, Controls
View.Set ("offscreenonly")
var startx : real := 0
var starty : real := 0
var x : real := 0
var y : real := 0
var angle : real := 0
var velocity : real := 0
var bounciness : real := 10
var time1 : real := 0.0
procedure Projectile
x := (velocity * cosd (angle) * time1) + startx
y := (velocity * sind (angle) * time1 - 9.81 * time1 ** 2 / 2) + starty
end Projectile
procedure Bounce
if y <= starty then
if (velocity) > 0 then
time1 := 0
startx := x
starty := y
velocity -= bounciness
end if
if (velocity) < 0 then
velocity := 0
end if
end if
end Bounce
procedure Movement
loop
time1 += .1 %The time and how fast it is moving
Projectile %applying movements to the projectile
Bounce %Detect ground and bounce back
drawfilloval (round (x), round (y), 5, 5, 12) %draw the projectile
View.Update
delay (10)
drawfilloval (round (x), round (y), 5, 5, white) %erase the projectile
exit when velocity = 0 or x > maxx
end loop
end Movement
procedure Controls
var key1 : array char of boolean
loop
Input.KeyDown (key1)
delay (10)
if key1 ('w') then
velocity += 1
if velocity > 300
then
velocity := 300
end if
locate (2, 2)
put velocity
elsif key1 ('s') then
velocity -= 1
if velocity < 0
then
velocity := 0
end if
locate (2, 2)
put velocity
elsif key1 ('a') then
angle += 1
if angle > 90
then
angle := 90
end if
locate (2, 2)
put angle
elsif key1 ('d') then
angle -= 1
if angle < 0
then
angle := 0
end if
locate (2, 2)
put angle
elsif key1 ('f') then
Projectile
Bounce
Movement
exit
end if
View.Update
end loop
startx := 0
starty := 0
x := 0
y := 0
end Controls
end projectileMovement
var player : ^projectileMovement
new player
loop
player -> Controls
end loop
|
Lastly, I just have to laugh. A degree in philosophy. Hahaha. But to each his own, I guess. |
|
|
|
|
 |
Cervantes

|
Posted: Fri Jun 10, 2005 9:43 pm Post subject: (No subject) |
|
|
Skeletor wrote: It looks like it is the bandwagon afterall.
No man, we're just trying to tell you that using someone elses code without understanding how it works is not the way to go. Programming is all about learning, and you learn nothing by using someone else's code that you don't understand. What wtd says is very, very true:
wtd, in several threads, wrote:
"It's better to be wrong, and know why, than to be right by chance."
lyam_kaskade wrote:
Lastly, I just have to laugh. A degree in philosophy. Hahaha. But to each his own, I guess.
 |
|
|
|
|
 |
|
|