Putting a fraction into lowest terms
Author |
Message |
Brutus
|
Posted: Mon Jan 17, 2005 12:30 pm Post subject: Putting a fraction into lowest terms |
|
|
Hey everyone, I'm new here so I'm not really sure how to post, but here goes. I am making a program that is supposed to calculate slpoe of a line segment. I can get the slope, but the problem is getting it into lowest terms. Any one know how I would achieve this? here is my program so far:
code: |
%Analytic Geometry program opening
var coor1 : int
coor1 := 0
var coor2 : int
coor2 := 0
var coor3 : int
coor3 := 0
var coor4 : int
coor4 := 0
var answer : int
put "Welcome to the Analytic Geometry program. This program is designed to calculate the slope, length and equasion of a line."
delay (8000)
cls
loop
put "Please enter the 'x' co-ordinate for the first endpoint of your line."
get coor1
put "Now the 'y' co-ordinate for the first endpoint of your line."
get coor2
put "Ok thanks. Now I need the 'x' co-ordinate for the second endpoint."
get coor3
put "Got it. Now the 'y' co-ordinate for the second endpoint"
get coor4
delay (5000)
cls
put "Alright, I've got it all worked out now, so go ahead and choose an option."
loop
put "For the slope of you line, press 1."
put "For the length of your line, press 2."
put "For the equasion of your line, press 3."
put "To re-enter your numbers, press 4."
get answer
exit when answer = 4
if answer = 1 then
put "The slope of your line is ", coor2 - coor4, "/", coor1 - coor3, "."
elsif answer = 2 then
put "The length of your line is "
elsif answer = 3 then
put "The equasion of your line is "
else
put "Hey! Come on now buddy. I was nice enough to bail you out by doing all the hard stuff for you, so do me a favour and choose one of the options I gave you."
end if
delay (10000)
cls
end loop
end loop
|
thanks in advance to whoever helps me |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
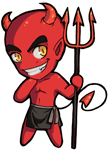
|
Posted: Mon Jan 17, 2005 1:27 pm Post subject: (No subject) |
|
|
to get a fraction in its lowest term, you find the factors of both nominator and denominator and divide them both by their common factors. |
|
|
|
|
 |
Bacchus

|
Posted: Mon Jan 17, 2005 3:34 pm Post subject: (No subject) |
|
|
i sugest using mod to see the largest common denometer
code: | var num1,num2,highest:int
num1:=6
num2:=9
if num1<num2 then
for decreasing i:num1..1
if num1 mod i =0 & num2 mod i =0 then
highest:=i
exit
end if
end for
elsif num1<num2 then
for decreasing i:num2..1
if num1 mod i =0 & num2 mod i =0 then
highest:=i
exit
end if
end for
else
highest:=num1
end if | not the bset but u get the idea |
|
|
|
|
 |
wtd
|
Posted: Mon Jan 17, 2005 4:54 pm Post subject: (No subject) |
|
|
Indentation is a good thing.  |
|
|
|
|
 |
AsianSensation
|
Posted: Mon Jan 17, 2005 6:34 pm Post subject: (No subject) |
|
|
check up on Euclid's Algorithm. It's a very good way to find the GCD between two numbers and there are surprisingly quite alot of computer programming code out there regarding Euclid's Algorithm. |
|
|
|
|
 |
Bacchus

|
Posted: Mon Jan 17, 2005 9:55 pm Post subject: (No subject) |
|
|
wtd wrote: Indentation is a good thing.  ya i kno but i didnt wrote it in turing and im lazy  |
|
|
|
|
 |
[Gandalf]

|
Posted: Tue Jan 18, 2005 9:00 pm Post subject: (No subject) |
|
|
Here's a quick tip on making your program more 'efficient'.
code: |
var coor4 : int
coor4 := 0 |
turns into
code: |
var coor4 := 0 %or if you prefer: var coor4 : int := 0
|
If anything, I would use the mod function - its probably more simple than some Eulcid's Algorithm ( lol, but it might not work as well). |
|
|
|
|
 |
AsianSensation
|
Posted: Tue Jan 18, 2005 9:37 pm Post subject: (No subject) |
|
|
Euclid's Algorithm is an algorithm, that is to say, a set of instructions that you can follow and produce some result, that could be used over and over again until the desired answer is found.
Mod in turing is a function, and it only returns only 1 value. They are not the samething.
Besides, Euclid's algorithm, when typed into CS code, will need the mod function. As the math logic behind it even calls for the use of mod. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Brutus
|
Posted: Wed Jan 19, 2005 12:24 pm Post subject: (No subject) |
|
|
Ok, thanks very much to all that posted.
Bacchus, I tryed to use your idea, but i had to add the abs thing due to negative number. So here's what I have now.
code: | procedure lowestterms (var rise, run: int)
if abs (rise) > abs (run) then
for i: decreasing abs run .. 2
if rise mod i = 0 and run mod i - 0 then
rise:= rise/i
run:= run/i
end if
end for
else for i: decreasing abs (rise) .. 2
if run mod i = 0 and rise mod i = 0 then
rise := rise/i
run:= run/i
end if
end for
end if
end lowestterms |
Problem is now, that it won't run with the "i" and decreasing things. Any idea why? |
|
|
|
|
 |
scottyrush13
|
Posted: Wed Jan 19, 2005 12:32 pm Post subject: (No subject) |
|
|
i think the other i's inside the for statement need to be in brackets |
|
|
|
|
 |
Bacchus

|
Posted: Wed Jan 19, 2005 3:44 pm Post subject: (No subject) |
|
|
no but the decreasing part has to come b4 the i ex) for decreasing i:5..1 |
|
|
|
|
 |
Brutus
|
Posted: Thu Jan 20, 2005 12:03 pm Post subject: (No subject) |
|
|
Thanks scotty and Bacchus. Hahaha, ya, the decreasing comes before the "i". Also, the "/" had to be changed to div. I hate it when the hardest answer to find is the simple one.... Anyways, thanks again. |
|
|
|
|
 |
phuong

|
Posted: Tue May 31, 2005 11:24 am Post subject: (No subject) |
|
|
if you're still interested... (don't think so but anyway)
here's a program that CAN reduce fractions. check it out.
here's the datafile for it too. |
|
|
|
|
 |
[Gandalf]

|
Posted: Wed Jun 01, 2005 10:10 am Post subject: (No subject) |
|
|
Well, I don't know - this was quite a while ago. I would just post something in the source code section - a new topic.
Also, when you submit something, include all the files neccessary. |
|
|
|
|
 |
|
|