Need help with creating odds
Author |
Message |
Scooter341
|
Posted: Mon Apr 11, 2005 10:35 am Post subject: Need help with creating odds |
|
|
Thank you Cervantes for the help with the music checking proc. My program is a Slot Machine game and it picks random numbers between 1 and 5 to display instead of cherries or whatever. What I'm hoping to do now is to set up an odds system where the odds of getting three 1's is greater than three 4's, but I have no idea where to start. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
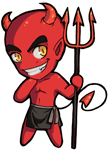
|
Posted: Mon Apr 11, 2005 10:52 am Post subject: (No subject) |
|
|
You should be rolling for a range that represents a value.
Such as in Rand.Int(1,10) :: 1~5 represents 1, 6~8 represents 2, 9~10 represents 3.
This gives you:
50% 1
30% 2
20% 3
It would be easier (and better) to with with a reference array, but a few if statements to check for ranges would do. |
|
|
|
|
 |
Mazer

|
Posted: Mon Apr 11, 2005 10:55 am Post subject: (No subject) |
|
|
I would think you wouldn't be trying to specify the odds of getting 3 of a specific number, just the odds (let's say probability instead) of getting 1 specified number.
ie, instead of doing Rand.Int(1, 5) which would give an equal probability of getting each number, do something like Rand.Int(1, 10).
Then, if you get some number in the range of 1 to 3, let it be a 1. If you get 4 - 5 let that be two. If you get 6 - 8, let it be 3. Then 9 is 4, and 10 is 5. In this way:
- you have 3 out of 10 chances (30% chance) of getting a 1
- you have 2 out of 10 chances (20% chance) of getting a 2
- you have 3 out of 10 chances (30% chance) of getting a 3
- you have 1 out of 10 chances (10% chance) of getting a 4
- you have 1 out of 10 chances (10% chance) of getting a 5
And the example code might look like:
code: |
var randomNumber : int % a number from our random function
var finalNumber : int % the number determined by our probability stuff
randomNumber := Rand.Int(1, 10)
if randomNumber >= 1 and randomNumber <= 3 then
finalNumber := 1
elsif randomNumber >= 4 and randomNumber <= 5 then
finalNumber := 2
elsif randomNumber >= 6 and randomNumber <= 8 then
finalNumber := 3
elsif randomNumber = 9 then
finalNumber := 4
elsif randomNumber = 10 then
finalNumber := 5
end if
put finalNumber
|
That's just a simple way of writing it. Hope that helps you out somewhat, let me know if you need me to clarify/rephrase anything.
-Nick |
|
|
|
|
 |
Scooter341
|
Posted: Tue Apr 12, 2005 12:28 pm Post subject: (No subject) |
|
|
Thanks a bunch Coutsos. It works great now. All I have to do is set up a GUI. |
|
|
|
|
 |
|
|