A few questions on binary addition
Author |
Message |
Pa|2a|)oX
|
Posted: Wed Feb 16, 2005 8:54 pm Post subject: A few questions on binary addition |
|
|
Hello everyone, firstly I appreciate any feedback that you may have.
I have two problems. The program I am making is a binary addition program that takes any two binary values and adds them together. Certain variables in the program need to be interchanged between string and integer variables to get certain functions to work (i.e. length (), addition, and indexing var (x)).
To properly add two binary values together, I need both amounts to be equal length. The only way I have found of doing this is with string variables. However, I cannot add these values together in the string form, so I have to use strint to convert them into integers. The problem with strint is that it drops and leading zeros and just takes the significant digits of the string value (i.e. if the value is 00000010 it just takes 10).
Is there a way around this? I need to keep all the zeros.
The other thing is the addition of these integers. I cannot add strings which is a real pain in the but, because I cannot index an integer variable (I cannot query the variable to tell me what number is in x position).
With a string variable I can say:
put word1 (3)
but I cannot do the same with an integer.
Is there a way around this?
Thanks for any responses, I hope I made this as clear as possible to understand.
All the best,
Kostia |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Bacchus

|
Posted: Wed Feb 16, 2005 9:20 pm Post subject: (No subject) |
|
|
nope no way around, can index integers and cant add string (besides adding parts onto each other to make a longer string). my sugestion is to convert the binary number to regular count and add it that way before changing it back to binary. |
|
|
|
|
 |
Tony
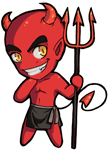
|
Posted: Thu Feb 17, 2005 9:47 am Post subject: (No subject) |
|
|
Pa|2a|)oX : please use more descriptive titles (edited this for you, but next time follow the guidelines)
Bacchus : that was not very nice of you.
now to the questions..
indexing integers:
Turing: |
function intIndex (number, i : int) : real
result floor (((number / (10 ** i )) - floor (number / (10 ** i ))) * 10)
end intIndex
var num : int := 12345678
for i : 1 .. 8
put i, "th index of ", num, " is : ", intIndex (num, i )
end for
|
index starts from the right and it doesn't work for numbers over 9 digits in length. But if you're working with bytes (8 bits) you should be in the clear.
Ether way, if you're adding binary - work in binary. None of that fansy "watch me turn binary into string into integer back into string and finally back into binary" Ekk
Turing: |
var binary1 : string := "0010" % binary 2
var binary2 : string := "0101" % binary 5
put strint (binary1, 2) + strint (binary2, 2)
%2 + 5 = 7
|
|
|
|
|
|
 |
Pa|2a|)oX
|
Posted: Thu Feb 17, 2005 1:53 pm Post subject: (No subject) |
|
|
Thanks for your help. I got it to work.. Check it out.. used arrays.
code: |
View.Set ("graphics:340;200,position:bottom;left,nobuttonbar")
drawfillbox (0, 0, maxx, maxy, black)
colorback (black)
color (yellow)
%Variable Declaration
var snum1, snum2 : string
%Get snum1, snum2
put "Please enter the first binary number.."
get snum1
put "Please enter the second binary number.."
get snum2
%Variable length of snum1,snum2
var lnum1 : int := length (snum1)
var lnum2 : int := length (snum2)
%Length of StringNum1 is Greater
procedure glnum1
var dif : int := lnum1 - lnum2
var lenum2 : string := snum2
for x : 1 .. dif
lenum2 := "0" + lenum2
end for
%%%%%%%%%%%
var add1, add2 : array 1 .. lnum1 of int
var final : array 1 .. lnum1 of int
var fdigit : int := 0
var carry : int := 0
for i : 1 .. lnum1
add1 (i) := strint (snum1 (i))
end for
for i : 1 .. lnum1
add2 (i) := strint (lenum2 (i))
end for
for decreasing k : lnum1 .. 1
if k = 1 and add1 (k) + add2 (k) = 0 and carry = 0 then
fdigit := 0
elsif k = 1 and add1 (k) + add2 (k) = 1 and carry = 0 then
fdigit := 1
elsif k = 1 and add1 (k) + add2 (k) = 1 and carry = 1 then
fdigit := 10
elsif k = 1 and add1 (k) + add2 (k) = 2 and carry = 0 then
fdigit := 10
elsif k = 1 and add1 (k) + add2 (k) = 2 and carry = 1 then
fdigit := 11
end if
%%%%
if add1 (k) + add2 (k) = 0 and carry = 0 then
final (k) := 0
carry := 0
elsif add1 (k) + add2 (k) = 0 and carry = 1 then
final (k) := 1
carry := 0
elsif add1 (k) + add2 (k) = 1 and carry = 0 then
final (k) := 1
carry := 0
elsif add1 (k) + add2 (k) = 1 and carry = 1 then
final (k) := 0
carry := 1
elsif add1 (k) + add2 (k) = 2 and carry = 0 then
final (k) := 0
carry := 1
elsif add1 (k) + add2 (k) = 2 and carry = 1 then
final (k) := 1
carry := 1
end if
end for
put "The addition these two values produce.."
put fdigit ..
if lnum1 > 1 then
for t : 2 .. lnum1
put final (t) ..
end for
end if
end glnum1
%Equal Amount
procedure glequal
var add1, add2 : array 1 .. lnum1 of int
var final : array 1 .. lnum1 of int
var fdigit : int := 0
var carry : int := 0
for i : 1 .. lnum1
add1 (i) := strint (snum1 (i))
end for
for i : 1 .. lnum2
add2 (i) := strint (snum2 (i))
end for
for decreasing k : lnum1 .. 1
if k = 1 and add1 (k) + add2 (k) = 0 and carry = 0 then
fdigit := 0
elsif k = 1 and add1 (k) + add2 (k) = 1 and carry = 0 then
fdigit := 1
elsif k = 1 and add1 (k) + add2 (k) = 1 and carry = 1 then
fdigit := 10
elsif k = 1 and add1 (k) + add2 (k) = 2 and carry = 0 then
fdigit := 10
elsif k = 1 and add1 (k) + add2 (k) = 2 and carry = 1 then
fdigit := 11
end if
%%%%
if add1 (k) + add2 (k) = 0 and carry = 0 then
final (k) := 0
carry := 0
elsif add1 (k) + add2 (k) = 0 and carry = 1 then
final (k) := 1
carry := 0
elsif add1 (k) + add2 (k) = 1 and carry = 0 then
final (k) := 1
carry := 0
elsif add1 (k) + add2 (k) = 1 and carry = 1 then
final (k) := 0
carry := 1
elsif add1 (k) + add2 (k) = 2 and carry = 0 then
final (k) := 0
carry := 1
elsif add1 (k) + add2 (k) = 2 and carry = 1 then
final (k) := 1
carry := 1
end if
end for
put "The addition these two values produce.."
put fdigit ..
if lnum1 > 1 then
for t : 2 .. lnum1
put final (t) ..
end for
end if
end glequal
%Procedure call
if lnum1 > lnum2 then
glnum1
elsif lnum1 < lnum2 then
put "Not correct arithmetic, second binary number must be smaller than the first."
else
glequal
end if
|
|
|
|
|
|
 |
Tony
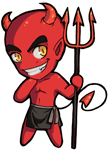
|
Posted: Thu Feb 17, 2005 2:29 pm Post subject: (No subject) |
|
|
what's with the limitations? why must the second binary number be smaller than the first?
here's a minor modification
Turing: |
View.Set ("graphics:340;200,position:bottom;left,nobuttonbar")
drawfillbox (0, 0, maxx, maxy, black)
colorback (black)
color (yellow)
%Variable Declaration
var snum1, snum2 : string
%Get snum1, snum2
loop
put "Please enter the first binary number.."
get snum1
put "Please enter the second binary number.."
get snum2
put "The addition these two values produce.."
put intstr (strint (snum1, 2) + strint (snum2, 2), length (intstr (strint (snum1, 2) + strint (snum2, 2))), 2)
end loop
|
 |
|
|
|
|
 |
Bacchus

|
Posted: Thu Feb 17, 2005 4:03 pm Post subject: (No subject) |
|
|
tony wrote: Bacchus : that was not very nice of you.
??? how was i not nice? he asked if there way to index integer and i didnt think there was a way so i said there wasnt. kno i kno there is, good idea on that. i still say it would be easier to just convert it to an accuall integer. but good none the less |
|
|
|
|
 |
Pa|2a|)oX
|
|
|
|
 |
Tony
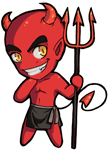
|
Posted: Thu Feb 17, 2005 5:38 pm Post subject: (No subject) |
|
|
Bacchus: you should have said that you don't know of a way to do such. Only if you're sure because of a documented limitation.. anyways
Pa|2a|)oX: lookup (Turing -> F10) the functionality of strint() and intstr(). They have an option of working in a base of choice. If for example you were to replace all the , 2 to , 16 your program would be in hexadecimal mode.
btw, there's some extra junk in there that I took out, but I guess it didn't edit.. replace length(...) with 0 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Pa|2a|)oX
|
Posted: Thu Feb 17, 2005 9:11 pm Post subject: (No subject) |
|
|
Thank Tony. However the teacher wouldn't accept that, he wanted there to be an algorythm for solving this problem, and not just using built in functions.
I'm actually quite proud that I got it to work like that  |
|
|
|
|
 |
Tony
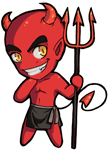
|
Posted: Fri Feb 18, 2005 10:12 am Post subject: (No subject) |
|
|
Pa|2a|)oX wrote: Thank Tony. However the teacher wouldn't accept that, he wanted there to be an algorythm for solving this problem, and not just using built in functions.
yeah.. I'm just being lazy (although in programming it is great thing)
do whatever you're doing. You can pull off a joke about how that is still cheating since you're not using assembly And just use my code snippet to varify your results. |
|
|
|
|
 |
|
|