Author |
Message |
MysticVegeta

|
Posted: Fri Jan 21, 2005 5:19 pm Post subject: Hmm breaking strings |
|
|
If I have a string i wanna break it by 2. What do i do?
For eg: 1234567890 will result in
24680
see what i mean? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
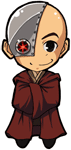
|
Posted: Fri Jan 21, 2005 5:24 pm Post subject: (No subject) |
|
|
You need to use subtrings and a forloop that goes up by 2.
example:
code: |
var temp : string := "1234567890"
for i : 1 .. length (temp) by 2
put temp (i+1)
end for
|
The i+1 is the index of the string, you need to add 1 b/c it whould give u the odd numbers other wise.
The length function returns the number of chars in a string |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
MysticVegeta

|
Posted: Fri Jan 21, 2005 8:14 pm Post subject: (No subject) |
|
|
Hacker Dan wrote: You need to use subtrings and a forloop that goes up by 2.
example:
code: |
var temp : string := "1234567890"
for i : 1 .. length (temp) by 2
put temp (i+1)
end for
|
The i+1 is the index of the string, you need to add 1 b/c it whould give u the odd numbers other wise.
The length function returns the number of chars in a string
oO I was having a simple error <_> i forgot +1 lol thanks a lot btw |
|
|
|
|
 |
MysticVegeta

|
Posted: Sat Jan 22, 2005 11:07 am Post subject: (No subject) |
|
|
Uh yea i almost forgot, what if i have a string like this
code: | var a : string := "18"
var breaks : array 1 .. length (a) of string
for x : 1 .. length (a)
breaks (x) := a (x)
put breaks (x)
end for
|
How do i add 1+8 after breaking them? |
|
|
|
|
 |
basketball4ever
|
Posted: Sat Jan 22, 2005 11:31 am Post subject: (No subject) |
|
|
wat do u mean adding em....??? u really got to work on your english. |
|
|
|
|
 |
Bacchus

|
Posted: Sat Jan 22, 2005 12:28 pm Post subject: (No subject) |
|
|
im guessing either added them to gether (18=1+8=9) or adding them back together (18=1+8=18) to do that:
1st one
code: | var a : string := "18"
var breaks : array 1 .. length (a) of string
var result : int:=0
for x : 1 .. length (a)
breaks (x) := a (x)
result+=intstr(a(x))
put breaks (x)
end for |
2nd one
code: | var a : string := "18"
var breaks : array 1 .. length (a) of string
var result : string:=""
for x : 1 .. length (a)
breaks (x) := a (x)
result+=a(x)
put breaks (x)
end for |
theres other ways but meh |
|
|
|
|
 |
basketball4ever
|
Posted: Sat Jan 22, 2005 12:33 pm Post subject: (No subject) |
|
|
i'm guessing the first one... cos theres no point in adding them back together i guess  |
|
|
|
|
 |
MysticVegeta

|
Posted: Sat Jan 22, 2005 1:59 pm Post subject: (No subject) |
|
|
Bacchus wrote: im guessing either added them to gether (18=1+8=9) or adding them back together (18=1+8=18) to do that:
1st one
code: | var a : string := "18"
var breaks : array 1 .. length (a) of string
var result : int:=0
for x : 1 .. length (a)
breaks (x) := a (x)
result+=intstr(a(x))
put breaks (x)
end for |
2nd one
code: | var a : string := "18"
var breaks : array 1 .. length (a) of string
var result : string:=""
for x : 1 .. length (a)
breaks (x) := a (x)
result+=a(x)
put breaks (x)
end for |
theres other ways but meh
Ok , it works but what if i want to add strings stored in an array for eg:
Number 1 -> 182345
Number 2 -> 870934
but i want the results to be 23 for number 1
31 for Number2
Does it mean i have to convert results into array too? and make 2 of them to make each of them store the total of one of the number?
How do i do that? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Bacchus

|
Posted: Sat Jan 22, 2005 4:24 pm Post subject: (No subject) |
|
|
code: | var a : string := "182345"
var breaks : array 1 .. length (a) of string
var result : int:=0
for x : 1 .. length (a)
breaks (x) := a (x)
result+=intstr(a(x))
put breaks (x)
end for
put "ruslting amount is: ",result | still works, all you need is integer variable set to 0 then just add the number |
|
|
|
|
 |
basketball4ever
|
Posted: Sat Jan 22, 2005 5:25 pm Post subject: (No subject) |
|
|
Bacchus wrote: code: | var a : string := "182345"
var breaks : array 1 .. length (a) of string
var result : int:=0
for x : 1 .. length (a)
breaks (x) := a (x)
result+=intstr(a(x))
put breaks (x)
end for
put "ruslting amount is: ",result | still works, all you need is integer variable set to 0 then just add the number
Instead of intstr... it should be strint ur changing the original string to an int |
|
|
|
|
 |
Bacchus

|
Posted: Sat Jan 22, 2005 5:45 pm Post subject: (No subject) |
|
|
oops had it backwards, srry i was using intstr earlier and quite a bit of it |
|
|
|
|
 |
basketball4ever
|
Posted: Sat Jan 22, 2005 6:06 pm Post subject: (No subject) |
|
|
Bacchus wrote: oops had it backwards, srry i was using intstr earlier and quite a bit of it
no worries : P just trying to help out our non-english friend "mystic vegeta" here  |
|
|
|
|
 |
MysticVegeta

|
Posted: Sat Jan 22, 2005 8:27 pm Post subject: (No subject) |
|
|
Um incase you dont know, please read my question again, i am too advanced to point that strint thing out, i just looked at it and didnt bother to waste time on posting that... i just changed it when i was using it. Oh yea. Incase you dont know, here is the problem i am trying to solve and here is the code i have for it so far, so i dont see the point of being non - english jackie.
http://www.ldcsb.on.ca/schools/sta/DWITE/Problem1Nov2004.pdf
code: |
var one : int
var lines, answer, lines2, backtostr, break2 : array 1 .. 3 of string
var stringint, intans, backtoint : array 1 .. 3 of int
open : one, "Data11.txt", get
for x : 1 .. 3
get : one, lines (x)
if length (lines (x)) mod 2 = 0 then
for a : 1 .. length (lines (x)) by 2
lines2 (x) := lines (x) (a + 1)
end for
else
lines (x) := lines (x) (1 .. * -1)
for a : 1 .. length (lines (x)) by 2
lines2 (x) := lines (x) (a + 1)
stringint (x) := strint (lines2 (x))
intans (x) := stringint (x) * 2
backtostr (x) := intstr (intans (x))
for s : 1 .. length (backtostr (x))
break2 (x) := backtostr (x) (s)
backtoint (x) := strint (break2 (x))
put backtoint (x)
end for
end for
end if
end for
close (one)
open : one, "Out11.txt", put
for s : 1 .. 3
end for
|
|
|
|
|
|
 |
Cervantes

|
Posted: Sat Jan 22, 2005 8:51 pm Post subject: (No subject) |
|
|
MysticVegeta wrote: i am too advanced to point that strint thing out
MysticVegeta wrote: I know that your level doesnt stand a chance against mine, Yes i am the one that taught your mom and dad how to program
You know, modesty is a good thing. |
|
|
|
|
 |
MysticVegeta

|
Posted: Sat Jan 22, 2005 9:39 pm Post subject: (No subject) |
|
|
Cervantes wrote: MysticVegeta wrote: i am too advanced to point that strint thing out
MysticVegeta wrote: I know that your level doesnt stand a chance against mine, Yes i am the one that taught your mom and dad how to program
You know, modesty is a good thing.
I know man, I really do. But the thing is that basketball4ever is in my TIK class and always jealous and bothers me in every topic even tho i dont start the argument.... Ok anyways can you help me in my problem? |
|
|
|
|
 |
|